How to Convert String to Float in PHP
- Use the Type Casting to Convert a String to Float in PHP
-
Use the
floatval()
Function to Convert a String to Float in PHP -
Use the
number_format()
Function to Convert a String to Float in PHP
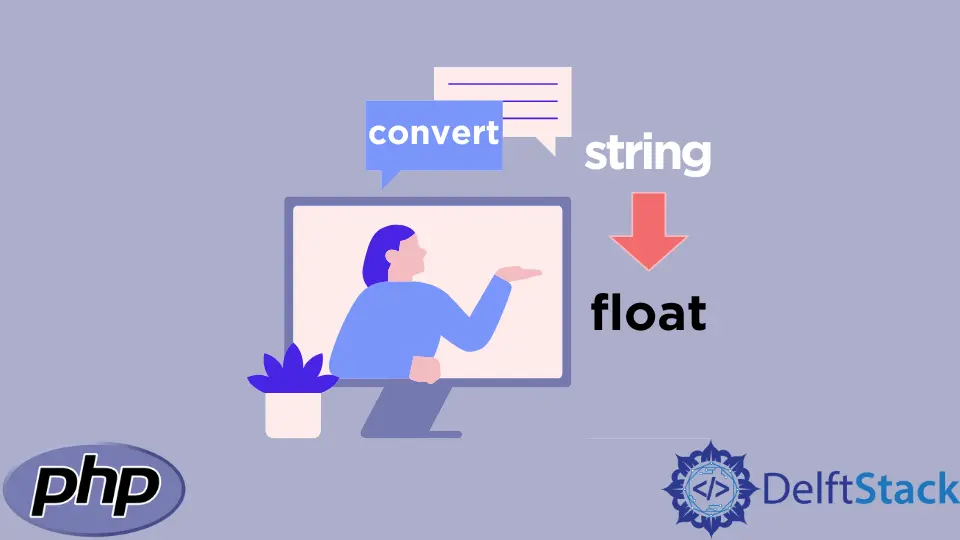
This article will introduce different methods to convert a string to float in PHP.
Use the Type Casting to Convert a String to Float in PHP
We can use type casting to convert one data type to the variable of another data type. Using typecasting, we can convert a string to float in PHP. The correct syntax to use type casting for the conversion of a string to float is as follows.
$floatVar = (float) $stringVar;
This is one of the simplest methods to convert PHP string to float. The program below shows how we can use the type casting to convert a string to float in PHP.
<?php
$mystring = "0.5674";
echo("This float number is of string data type ");
echo($mystring);
echo("\n");
$myfloat = (float) $mystring;
echo("Now, this float number is of float data type ");
echo($myfloat);
?>
Output:
This float number is of string data type 0.5674
Now, this float number is of float data type 0.5674
Use the floatval()
Function to Convert a String to Float in PHP
Another method is to use PHP’s floatval()
function to convert a string to float. This function extracts the float value from the passed variable. For example, if the given variable is a string that contains a float value, then this function will extract that value. The correct syntax to use this function is as follows.
floatval($variable);
The floatval()
function has only one parameter. The detail of its parameter is as follows.
Variables | Description |
---|---|
$variable |
It is the variable that contains the float value. It can be of any data type. But, it cannot be an object. |
This function returns the extracted float value. The program below shows how we can use the floatval()
function to convert a string to float in PHP.
<?php
$mystring = "0.5674";
echo("This float number is of string data type ");
echo($mystring);
echo("\n");
$myfloat = floatval($mystring);
echo("Now, this float number is of float data type ");
echo($myfloat);
?>
Output:
This float number is of string data type 0.5674
Now, this float number is of float data type 0.5674
The function has returned the extracted float value. We have stored this value in $myfloat
variable.
Use the number_format()
Function to Convert a String to Float in PHP
We can also use the number_format()
function to convert a string to float. The number_format()
function is used for the formatting of the numbers is a number is passed as a parameter. If we pass a string containing the number as a parameter, it extracts the number from the string first. The correct syntax to use this function is as follows.
number_format($number, $decimals, $decimalpoint, $thousandseperator);
The number_format()
function has four parameters. The details of its parameters are as follows.
Variables | Description |
---|---|
$number |
It is the number that we want to format. In our case, it will be the string containing the float value. |
$decimals |
This parameter is used to specify the number of decimals after the decimal point. If not passed, then the function rounds the float value. |
$decimalpoint |
It is the symbol for the decimal point. It is . by default. |
$thousandseperator |
It is the symbol for the thousand separator. Its default value is , . |
This function returns the formatted float value. The program below shows the ways by which we can use the number_format()
function to convert a string to float in PHP.
<?php
$mystring = "0.5674";
echo("This float number is of string data type ");
echo($mystring);
echo("\n");
$myfloat = number_format($mystring, 4);
echo("Now, this float number is of float data type ");
echo($myfloat);
?>
Output:
This float number is of string data type 0.5674
Now, this float number is of float data type 0.5674
The function has returned the extracted float value. We have stored this value in the $myfloat
variable.