How to Convert String to Boolean in PHP
-
Use the
settype()
Function to Convert String to Boolean in PHP - Use the Cast Operators to Typecast String to Boolean in PHP
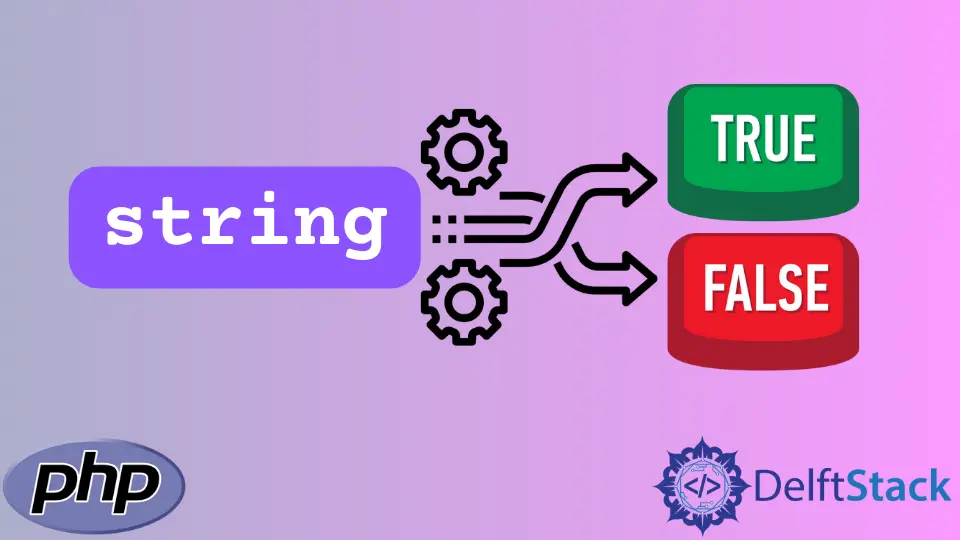
This article will introduce a few ways of converting a string into a Boolean in PHP.
Use the settype()
Function to Convert String to Boolean in PHP
We can use the settype()
function to convert a string to a Boolean in PHP. The function can convert one data type to another.
The syntax of the settype()
function is written below.
settype($var, $type)
Here, the $var
is the variable that will be converted. It can be of any data type.
The option $type
resembles the data type to which $var
is to be converted. The $var
option represents the data types like "boolean"
, "integer"
, "float"
, "string"
, etc.
The function returns a Boolean value, true
in case of success and false
in failure.
We can supply a string as the first parameter and the type "boolean"
as the second parameter in the settype()
function to convert the string to Boolean.
For example, create a custom function stringToBoolean()
that takes a parameter $str
. Inside the function, use the settype()
function where the parameter $str
is the first parameter and "boolean"
is the second parameter.
Use the var_dump()
function to show the information of the $str
variable. Next, outside the function, call the stringToBoolean()
three times with different arguments.
The arguments for each invocation are "yoyo"
, ""
and "0"
.
In the example below, each string is converted into a Boolean. The text "yoyo"
has a true
Boolean value while ""
and "0"
have the false
Boolean value.
Thus, we can convert the strings into Boolean using PHP’s settype()
function.
Example Code:
function stringToBoolean($str){
settype($str, "boolean");
var_dump($str);
}
stringToBoolean("yoyo");
stringToBoolean("");
stringToBoolean("0");
Output:
bool(true)
bool(false)
bool(false)
Use the Cast Operators to Typecast String to Boolean in PHP
We can easily convert a data type to another using the cast operator in PHP. We can use the cast operator just before the data type is cast.
The cast operator is the data type written between the round brackets. Some examples of cast operators are (int)
, (boolean)
, (string)
, etc.
To typecast a string to Boolean, we should use the (boolean)
cast operator just before the string.
For example, create a variable $bool1
and assign (boolean)"hey"
to it. Next, print the variable using var_dump()
function.
Similarly, typecast the strings ""
and "0"
into Boolean.
As a result, we can see the strings getting typecasted into Boolean. The Boolean value of the strings is shown in the output section.
Thus, we can typecast string to Boolean using cast operators in PHP.
Example Code:
$bool1 = (boolean)"hey";
$bool2 = (boolean)"";
$bool3 = (boolean)"0";
var_dump($bool1);
var_dump($bool2);
var_dump($bool3);
Output:
bool(true)
bool(false)
bool(false)
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedInRelated Article - PHP String
- How to Remove All Spaces Out of a String in PHP
- How to Convert DateTime to String in PHP
- How to Convert String to Date and Date-Time in PHP
- How to Convert an Integer Into a String in PHP
- How to Convert an Array to a String in PHP
- How to Convert a String to a Number in PHP