How to Get PHP String Length
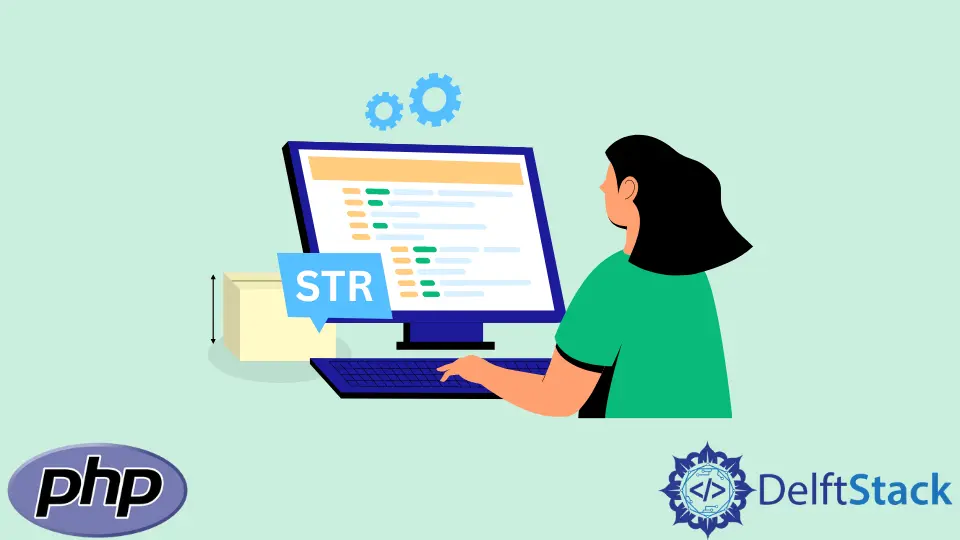
Measuring the length of a string in PHP is a fundamental task that every developer encounters. Whether you’re validating user input, processing text data, or simply manipulating strings, knowing how to accurately determine the size of a string is essential.
In this article, we’ll explore two primary functions in PHP that help you achieve this: strlen()
and mb_strlen()
. The former is perfect for standard strings, while the latter is crucial for multibyte character encodings, ensuring that you handle languages and characters beyond the basic ASCII set. Let’s dive into these functions and see how they can be used effectively.
Understanding String Length in PHP
When working with strings in PHP, you might find yourself needing to know how many characters or bytes a string contains. This is particularly important when dealing with user inputs or data processing where the length of the string can affect functionality. PHP provides built-in functions that make this task straightforward.
Using strlen() Function
The strlen()
function is the most commonly used method for measuring the length of a string in PHP. It returns the number of bytes in a string, which is usually the same as the number of characters for single-byte character encodings like ASCII. However, it’s important to note that strlen()
may not give accurate results for multibyte encodings, such as UTF-8.
Here’s how you can use the strlen()
function:
<?php
$string = "Hello, World!";
$length = strlen($string);
echo $length;
?>
Output:
13
In this example, we define a string variable $string
containing “Hello, World!”. The strlen()
function is then called, and it calculates the length of the string, which is 13 bytes. This method is efficient and works well for simple cases where the string consists of standard ASCII characters.
However, if your application needs to handle strings with multibyte characters, such as those in languages like Chinese or Arabic, strlen()
may not suffice. This is where the mb_strlen()
function comes into play.
Using mb_strlen() Function
The mb_strlen()
function is part of the Multibyte String (mbstring) extension in PHP. It is designed to handle multibyte character encodings, making it ideal for applications that require support for internationalization. This function returns the number of characters in a string, rather than bytes, which is crucial when dealing with multibyte encodings.
Here’s how to use the mb_strlen()
function:
<?php
$string = "こんにちは"; // "Hello" in Japanese
$length = mb_strlen($string, 'UTF-8');
echo $length;
?>
Output:
5
In this code snippet, we define a string variable $string
that contains “こんにちは”, which translates to “Hello” in Japanese. By using mb_strlen()
, we specify the encoding as ‘UTF-8’, ensuring that the function accurately counts the characters. The output is 5, reflecting the correct number of characters in the string, regardless of the byte size.
Using mb_strlen()
is essential in applications that require accurate character counting for multibyte strings, ensuring that your application behaves correctly across different languages and character sets.
Conclusion
In summary, measuring string length in PHP is a straightforward task thanks to the strlen()
and mb_strlen()
functions. While strlen()
works well for standard ASCII strings, mb_strlen()
is the go-to choice for multibyte character encodings. Understanding the differences between these functions can help you build more robust applications that handle text data efficiently. Whether you’re working on a simple project or a complex web application, knowing how to measure string length accurately is a skill that will serve you well.
FAQ
-
What is the difference between strlen() and mb_strlen()?
strlen() counts bytes, while mb_strlen() counts characters, making the latter suitable for multibyte encodings. -
Can I use strlen() for multibyte strings?
Yes, but it may not provide accurate results for characters outside the ASCII range. -
Do I need to enable the mbstring extension to use mb_strlen()?
Yes, the mbstring extension must be enabled in your PHP configuration to use mb_strlen(). -
How can I check if mbstring is enabled in PHP?
You can use the phpinfo() function or check the output of the function extension_loaded(‘mbstring’). -
Are there other string functions I should know about in PHP?
Yes, functions like substr(), str_replace(), and strpos() are also commonly used for string manipulation in PHP.