Examples on Using the SoapClient in PHP
- Get the Functions and Types From a Web Service in PHP
- PHP SOAP Example 1: Temperature Conversion
- PHP SOAP Example 2: Say Hello
- PHP SOAP Example 3: Simple Arithmetic
- PHP SOAP Example 4: Country Information
-
PHP SOAP Example 5: Temperature Conversion Using
NuSOAP
-
Bonus Example: SOAP Call With
cURL
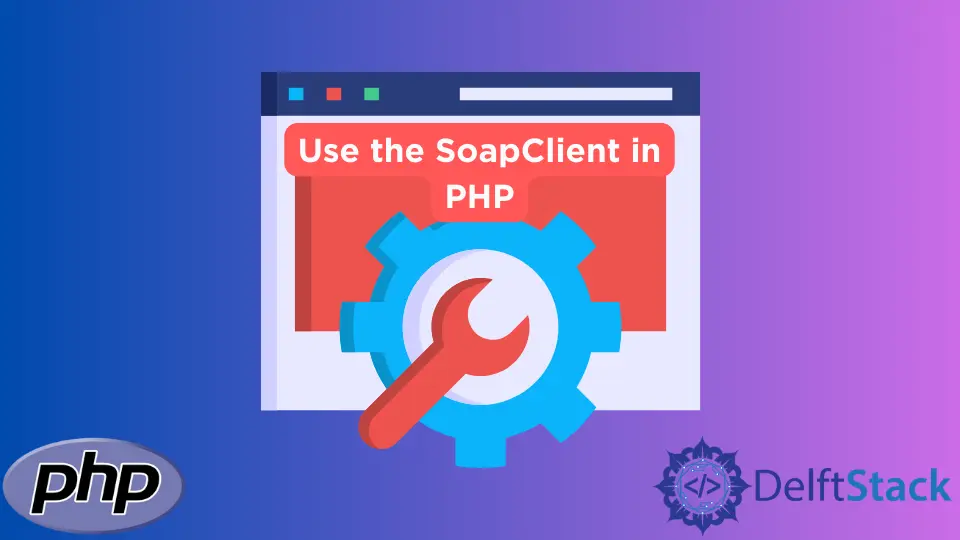
This article presents six examples of working with WSDL files using PHP SoapClient
. Before that, we’ll explain how you can get the function and types from a web service.
This is a must if you want to use the web service.
Get the Functions and Types From a Web Service in PHP
The first thing you must do before using a web service is to know its functions and types. That’s because a service function will return data from the service.
And the type is the data type of arguments you might supply to the function. We say might because, as you’ll find out later, not all service functions require an argument.
In PHP, you can use the following to get the functions and types from a service. Replace the service URL with another one, and PHP will return the names and types of the functions.
You should be connected to the internet for the code to work.
<?php
$client = new SoapClient("http://www.dneonline.com/calculator.asmx?WSDL");
echo "<b>The functions are:</b> <br/>";
echo "<pre>";
var_dump($client->__getFunctions());
echo "</pre>";
echo "<b>The types are:</b> <br />";
echo "<pre>";
var_dump($client->__getTypes());
echo "</pre>";
?>
Output (the following is an excerpt):
<b>The functions are:</b> <br/><pre>array(8) {
[0]=>
string(32) "AddResponse Add(Add $parameters)"
[1]=>
string(47) "SubtractResponse Subtract(Subtract $parameters)"
[2]=>
string(47) "MultiplyResponse Multiply(Multiply $parameters)"
[3]=>
string(41) "DivideResponse Divide(Divide $parameters)"
[4]=>
string(32) "AddResponse Add(Add $parameters)"
[5]=>
string(47) "SubtractResponse Subtract(Subtract $parameters)"
[6]=>
string(47) "MultiplyResponse Multiply(Multiply $parameters)"
[7]=>
string(41) "DivideResponse Divide(Divide $parameters)"
}
</pre><b>The types are:</b> <br /><pre>array(8) {
[0]=>
string(36) "struct Add {
int intA;
int intB;
}"
[1]=>
string(38) "struct AddResponse {
int AddResult;
}"
[2]=>
string(41) "struct Subtract {
int intA;
int intB;
}"
..........
Note:
- Under
types
, you’ll find the data type of the parameters defined for a function. - The data type must be the same when you supply arguments to the function in your code.
- If you see something like
int intA
in a type definition, the arguments must be an integer namedintA
.
Now that you know how to get a service function and type, you can start working with a service.
PHP SOAP Example 1: Temperature Conversion
W3schools provides a temperature converter WSDL file. From PHP, you can connect to this file to perform temperature conversion.
The steps to take for this (and other SOAP operations with SoapClient
) are as follows:
-
Create a new
SoapClient
object. -
Define the data that you’ll like to work with. This data should be in a format the function of the service can work with.
-
Call the function of the service.
-
Show the results.
We’ve implemented these steps in the following code.
<?php
// Create the client object and pass in the
// URL of the SOAP server.
$soap_client = new SoapClient('https://www.w3schools.com/xml/tempconvert.asmx?WSDL');
// Create an associative array of the data
// that you'll pass into one of the functions
// of the client.
$degrees_in_celsius = array('Celsius' => '25');
// Use a function of the client for the
// conversion.
$convert_to_fahrenheit = $soap_client->CelsiusToFahrenheit($degrees_in_celsius);
// Show the result using var_dump. Other PHP
// functions like echo will not work.
echo "<pre>";
var_dump($convert_to_fahrenheit);
echo "</pre>";
// Repeat the same process. This time, use the
// FahrenheitToCelsius function to convert a
// temperature from Fahrenheit to Celsius.
$degrees_in_fahrenheit = array('Fahrenheit' => '25');
$convert_to_celsius = $soap_client->FahrenheitToCelsius($degrees_in_fahrenheit);
echo "<pre>";
var_dump($convert_to_celsius);
echo "</pre>";
?>
Output:
<pre>object(stdClass)#2 (1) {
["CelsiusToFahrenheitResult"]=>
string(2) "77"
}
</pre><pre>object(stdClass)#3 (1) {
["FahrenheitToCelsiusResult"]=>
string(17) "-3.88888888888889"
}
</pre>
PHP SOAP Example 2: Say Hello
Our second example is a say hello
message using learnwebservices.com
. All you have to do is initiate a SOAP call to the service using SoapClient
.
Afterward, call on its SayHello
method and pass in your desired name. Then you can access the Message
method to display the message.
The message should be Hello your_desired_name!
. For example, if your_desired_name
is Martinez
, you’ll get an output of Hello Martinez!
.
This is what we’ve done in the next code.
<?php
$soap_client = new SoapClient('https://apps.learnwebservices.com/services/hello?wsdl');
// Call a function of the soap client.
$say_hello = $soap_client->SayHello(['Name' => 'Martinez']);
// Print the message from the client.
echo $say_hello->Message;
?>
Output:
Hello Martinez!
PHP SOAP Example 3: Simple Arithmetic
In this example, we use a web service that allows you to perform simple arithmetic. Such arithmetic includes addition, division, multiplication, and subtraction.
However, we’ll only show how to add and divide using the service. You should try out the rest because it’ll give you a better understanding of the service.
The arithmetic functions of this service require that the numbers have a name. These names are intA
and intB
, so we assign these names to the numbers in an associative array to make it easy.
Afterward, we pass this array to the service’s Add
and Divide
functions. The following code shows all these in action and the calculation results.
<?php
// Connect to a SOAP client that allows you
// to do basic calculations.
$soap_client = new SoapClient("http://www.dneonline.com/calculator.asmx?WSDL");
// Define data that'll use with the client. This
// time the client requires that arguments that
// you'll pass in has the name intA and intB.
// Therefore, we use that name in our array.
$numbers_for_the_client = array("intA" => 222, "intB" => 110);
$add_the_numbers = $soap_client->Add($numbers_for_the_client);
$divide_the_numbers = $soap_client->Divide($numbers_for_the_client);
// Show the results of the addition and
// division of numbers.
echo "<pre>";
var_dump($add_the_numbers);
var_dump($divide_the_numbers);
echo "</pre>";
?>
Output:
<pre>object(stdClass)#2 (1) {
["AddResult"]=>
int(332)
}
object(stdClass)#3 (1) {
["DivideResult"]=>
int(2)
}
</pre>
PHP SOAP Example 4: Country Information
If you want to get certain details about a country, the service in this example is for you. The service has many functions that’ll return details of a country.
Some of these functions are ListOfCountryNameByCodes
and ListOfContinentsByName
. In the following, we use ListOfCountryNameByCodes
to print the countries in the world.
<?php
// A soap client that allows you to view information
// about countries in the world
$soap_client = new SoapClient("http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso?WSDL");
// Use a client function to print country names.
$list_countries_names_by_code = $soap_client->ListOfCountryNamesByCode();
/*
Here is another function you can try out.
$list_of_continents_by_name = $client->ListOfContinentsByName();
*/
// Show the results.
echo "<pre>";
var_dump($list_countries_names_by_code);
echo "</pre>";
?>
Output (the following is an excerpt):
<pre>object(stdClass)#2 (1) {
["ListOfCountryNamesByCodeResult"]=>
object(stdClass)#3 (1) {
["tCountryCodeAndName"]=>
array(246) {
[0]=>
object(stdClass)#4 (2) {
["sISOCode"]=>
string(2) "AD"
["sName"]=>
string(7) "Andorra"
}
[1]=>
object(stdClass)#5 (2) {
["sISOCode"]=>
string(2) "AE"
["sName"]=>
string(20) "United Arab Emirates"
}
[2]=>
object(stdClass)#6 (2) {
["sISOCode"]=>
string(2) "AF"
["sName"]=>
string(11) "Afghanistan"
}
....
PHP SOAP Example 5: Temperature Conversion Using NuSOAP
NuSOAP
is a PHP tool that allows you to make SOAP connections to a web service. The mode of operation is like using a SoapClient
, but with the following difference.
- The
nusoap_client
requires the service address andwsdl
as its arguments. - The data you’ll work on must be in XML.
To use NuSOAP
, download it from SourceForge
and drop it in your current working directory. Then use the following code for the temperature conversion.
You’ll observe the steps are the same, with the exception detailed earlier. One more thing, the code works in PHP5
, and it returns bool
in PHP8
.
<?php
// This PHP script use NUSOAP for SOAP connection
// and it works in PHP5 ONLY.
require_once('nusoap.php');
// Specify the WSDL file and initiate a new
// NUSOAP client.
$wsdl = "https://www.w3schools.com/xml/tempconvert.asmx?WSDL";
$nusoap_client = new nusoap_client($wsdl, 'wsdl');
// Call on the required method of the client.
$function_from_client = "CelsiusToFahrenheit";
// We'll save the result of the entire operation
// in an array.
$result = array();
// Temperature for conversion.
$temperature = 86;
// Specify the temperature as an XML file
$temperature_as_xml= '<CelsiusToFahrenheit xmlns="https://www.w3schools.com/xml/">
<Celsius>' . $temperature . '</Celsius>
</CelsiusToFahrenheit>';
// If the function exists in the client, initiate
// the conversion.
if (isset($function_from_client)) {
$result['response'] = $nusoap_client->call($function_from_client, $temperature_as_xml);
}
// Show the results
echo "<pre>" . $temperature . ' Celsius => ' . $result['response']['CelsiusToFahrenheitResult'] . ' Fahrenheit' . "</pre>";
?>
Output:
<pre>86 Celsius => 186.8 Fahrenheit</pre>
Bonus Example: SOAP Call With cURL
You can use cURL
to make a SOAP call to a web service. Again we’ll use the W3Schools Temperature Conversion service.
Meanwhile, with cURL
, you must do the following when working with a service.
- The data should be in a
Soap Envelope
. - You must send content headers to the browser.
- You must define the
cURL
connection options.
We do all these and more in the following code; the code comments detail what’s going on at each step.
<?php
// This script allows you to use w3schools
// convert via curl.
$webservice_address = "https://www.w3schools.com/xml/tempconvert.asmx";
// The number you'll like to convert, in this case
// we'll like to convert a temperature in celsius
// fahrenheit.
$temperature = 56;
// Pass the temperature as an XML file.
// The XML format is based on the official
// format for the SOAP client by w3schools.
// You can find more at:
$temperature_as_xml = '<?xml version="1.0" encoding="utf-8"?>
<soap12:Envelope
xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="https://www.w3.org/2001/XMLSchema"
xmlns:soap12="https://www.w3.org/2003/05/soap-envelope">
<soap12:Body>
<CelsiusToFahrenheit xmlns="https://www.w3schools.com/xml/">
<Celsius>' . $temperature . '</Celsius>
</CelsiusToFahrenheit>
</soap12:Body>
</soap12:Envelope>';
// Define content headers
$content_headers = array(
'Content-Type: text/xml; charset=utf-8',
'Content-Length: '.strlen($temperature_as_xml)
);
// Initiate the CURL connection and define
// the connection options.
$init_curl_connection = curl_init($webservice_address);
curl_setopt($init_curl_connection, CURLOPT_POST, true);
curl_setopt($init_curl_connection, CURLOPT_HTTPHEADER, $content_headers);
curl_setopt($init_curl_connection, CURLOPT_POSTFIELDS, $temperature_as_xml);
curl_setopt($init_curl_connection, CURLOPT_RETURNTRANSFER, true);
curl_setopt($init_curl_connection, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($init_curl_connection, CURLOPT_SSL_VERIFYPEER, false);
// Use the curl_exec function to get the
// received data.
$recieved_data = curl_exec($init_curl_connection);
// Save the connection result in another variable.
// This will allow us to use it in an "if" statement
// without causing ambiguity.
$connection_result = $recieved_data;
// Check if there is an error.
if ($connection_result === FALSE) {
printf("CURL error (#%d): %s<br>\n", curl_errno($init_curl_connection),
htmlspecialchars(curl_error($init_curl_connection)));
}
// Close the CURL connection
curl_close ($init_curl_connection);
// Show the received data.
echo $temperature . ' Celsius => ' . $recieved_data . ' Fahrenheit';
?>
Output (in the browser):
56 Celsius => 132.8 Fahrenheit
Output (detailed version):
Celsius => <?xml version="1.0" encoding="utf-8"?>
<soap:Envelope xmlns:soap="https://www.w3.org/2003/05/soap-envelope" xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="https://www.w3.org/2001/XMLSchema">
<soap:Body>
<CelsiusToFahrenheitResponse xmlns="https://www.w3schools.com/xml/">
<CelsiusToFahrenheitResult>132.8</CelsiusToFahrenheitResult>
</CelsiusToFahrenheitResponse><
/soap:Body></soap:Envelope>Fahrenheit
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn