PHP에서 SoapClient 사용에 대한 예
- PHP의 웹 서비스에서 함수 및 유형 가져오기
- PHP SOAP 예제 1: 온도 변환
- PHP SOAP 예제 2: 인사하기
- PHP SOAP 예제 3: 간단한 산술
- PHP SOAP 예제 4: 국가 정보
-
PHP SOAP 예제 5:
NuSOAP
를 사용한 온도 변환 -
보너스 예:
cURL
을 사용한 SOAP 호출
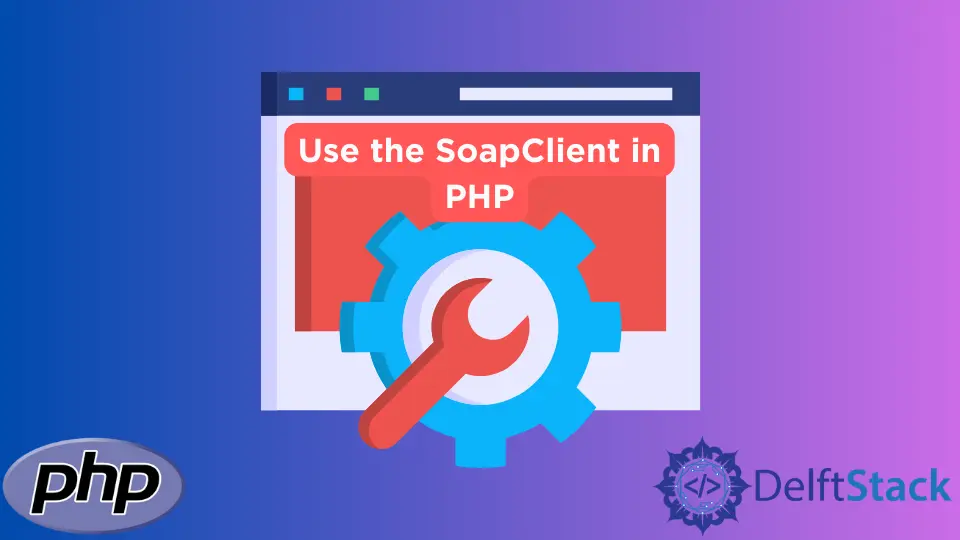
이 기사에서는 PHP SoapClient
를 사용하여 WSDL 파일로 작업하는 6가지 예를 보여줍니다. 그 전에 웹 서비스에서 함수와 유형을 가져오는 방법을 설명합니다.
웹 서비스를 사용하려면 필수입니다.
PHP의 웹 서비스에서 함수 및 유형 가져오기
웹 서비스를 사용하기 전에 가장 먼저 해야 할 일은 웹 서비스의 기능과 유형을 아는 것입니다. 서비스 함수가 서비스에서 데이터를 반환하기 때문입니다.
그리고 유형은 함수에 제공할 수 있는 인수의 데이터 유형입니다. 나중에 알게 되겠지만 모든 서비스 기능에 인수가 필요한 것은 아니기 때문에 “가능합니다"라고 말합니다.
PHP에서는 다음을 사용하여 서비스에서 함수와 유형을 가져올 수 있습니다. 서비스 URL을 다른 URL로 바꾸면 PHP가 함수의 이름과 유형을 반환합니다.
코드가 작동하려면 인터넷에 연결되어 있어야 합니다.
<?php
$client = new SoapClient("http://www.dneonline.com/calculator.asmx?WSDL");
echo "<b>The functions are:</b> <br/>";
echo "<pre>";
var_dump($client->__getFunctions());
echo "</pre>";
echo "<b>The types are:</b> <br />";
echo "<pre>";
var_dump($client->__getTypes());
echo "</pre>";
?>
출력(다음은 발췌):
<b>The functions are:</b> <br/><pre>array(8) {
[0]=>
string(32) "AddResponse Add(Add $parameters)"
[1]=>
string(47) "SubtractResponse Subtract(Subtract $parameters)"
[2]=>
string(47) "MultiplyResponse Multiply(Multiply $parameters)"
[3]=>
string(41) "DivideResponse Divide(Divide $parameters)"
[4]=>
string(32) "AddResponse Add(Add $parameters)"
[5]=>
string(47) "SubtractResponse Subtract(Subtract $parameters)"
[6]=>
string(47) "MultiplyResponse Multiply(Multiply $parameters)"
[7]=>
string(41) "DivideResponse Divide(Divide $parameters)"
}
</pre><b>The types are:</b> <br /><pre>array(8) {
[0]=>
string(36) "struct Add {
int intA;
int intB;
}"
[1]=>
string(38) "struct AddResponse {
int AddResult;
}"
[2]=>
string(41) "struct Subtract {
int intA;
int intB;
}"
..........
메모:
types
아래에서 함수에 대해 정의된 매개변수의 데이터 유형을 찾을 수 있습니다.- 코드에서 함수에 인수를 제공할 때 데이터 유형이 동일해야 합니다.
- 유형 정의에
int intA
와 같은 항목이 있는 경우 인수는intA
라는 이름의 정수여야 합니다.
이제 서비스 함수 및 유형을 가져오는 방법을 알았으므로 서비스 작업을 시작할 수 있습니다.
PHP SOAP 예제 1: 온도 변환
W3schools는 온도 변환기 WSDL 파일을 제공합니다. PHP에서 이 파일에 연결하여 온도 변환을 수행할 수 있습니다.
이를 위해 취해야 할 단계(및 SoapClient
를 사용한 기타 SOAP 작업)는 다음과 같습니다.
-
새
SoapClient
개체를 만듭니다. -
작업할 데이터를 정의합니다. 이 데이터는 서비스 기능이 사용할 수 있는 형식이어야 합니다.
-
서비스의 기능을 호출합니다.
-
결과를 보여줍니다.
다음 코드에서 이러한 단계를 구현했습니다.
<?php
// Create the client object and pass in the
// URL of the SOAP server.
$soap_client = new SoapClient('https://www.w3schools.com/xml/tempconvert.asmx?WSDL');
// Create an associative array of the data
// that you'll pass into one of the functions
// of the client.
$degrees_in_celsius = array('Celsius' => '25');
// Use a function of the client for the
// conversion.
$convert_to_fahrenheit = $soap_client->CelsiusToFahrenheit($degrees_in_celsius);
// Show the result using var_dump. Other PHP
// functions like echo will not work.
echo "<pre>";
var_dump($convert_to_fahrenheit);
echo "</pre>";
// Repeat the same process. This time, use the
// FahrenheitToCelsius function to convert a
// temperature from Fahrenheit to Celsius.
$degrees_in_fahrenheit = array('Fahrenheit' => '25');
$convert_to_celsius = $soap_client->FahrenheitToCelsius($degrees_in_fahrenheit);
echo "<pre>";
var_dump($convert_to_celsius);
echo "</pre>";
?>
출력:
<pre>object(stdClass)#2 (1) {
["CelsiusToFahrenheitResult"]=>
string(2) "77"
}
</pre><pre>object(stdClass)#3 (1) {
["FahrenheitToCelsiusResult"]=>
string(17) "-3.88888888888889"
}
</pre>
PHP SOAP 예제 2: 인사하기
두 번째 예는 learnwebservices.com
을 사용하는 “안녕하세요” 메시지입니다. SoapClient
를 사용하여 서비스에 대한 SOAP 호출을 시작하기만 하면 됩니다.
그런 다음 SayHello
메서드를 호출하고 원하는 이름을 전달합니다. 그런 다음 Message
메소드에 액세스하여 메시지를 표시할 수 있습니다.
메시지는 Hello your_desired_name!
이어야 합니다. 예를 들어 your_desired_name
이 Martinez
인 경우 Hello Martinez!
의 출력이 표시됩니다.
이것이 다음 코드에서 수행한 작업입니다.
<?php
$soap_client = new SoapClient('https://apps.learnwebservices.com/services/hello?wsdl');
// Call a function of the soap client.
$say_hello = $soap_client->SayHello(['Name' => 'Martinez']);
// Print the message from the client.
echo $say_hello->Message;
?>
출력:
Hello Martinez!
PHP SOAP 예제 3: 간단한 산술
이 예에서는 간단한 산술을 수행할 수 있는 웹 서비스를 사용합니다. 이러한 산술에는 더하기, 나누기, 곱하기 및 빼기가 포함됩니다.
다만, 서비스를 이용하여 덧셈과 나눗셈을 하는 방법만 보여드리겠습니다. 나머지는 서비스를 더 잘 이해할 수 있기 때문에 시도해야 합니다.
이 서비스의 산술 함수를 사용하려면 숫자에 이름이 있어야 합니다. 이러한 이름은 intA
및 intB
이므로 쉽게 하기 위해 이러한 이름을 연관 배열의 숫자에 할당합니다.
그런 다음 이 배열을 서비스의 Add
및 Divide
함수에 전달합니다. 다음 코드는 이러한 모든 동작과 계산 결과를 보여줍니다.
<?php
// Connect to a SOAP client that allows you
// to do basic calculations.
$soap_client = new SoapClient("http://www.dneonline.com/calculator.asmx?WSDL");
// Define data that'll use with the client. This
// time the client requires that arguments that
// you'll pass in has the name intA and intB.
// Therefore, we use that name in our array.
$numbers_for_the_client = array("intA" => 222, "intB" => 110);
$add_the_numbers = $soap_client->Add($numbers_for_the_client);
$divide_the_numbers = $soap_client->Divide($numbers_for_the_client);
// Show the results of the addition and
// division of numbers.
echo "<pre>";
var_dump($add_the_numbers);
var_dump($divide_the_numbers);
echo "</pre>";
?>
출력:
<pre>object(stdClass)#2 (1) {
["AddResult"]=>
int(332)
}
object(stdClass)#3 (1) {
["DivideResult"]=>
int(2)
}
</pre>
PHP SOAP 예제 4: 국가 정보
국가에 대한 특정 세부 정보를 얻으려면 이 예의 서비스가 적합합니다. 이 서비스에는 국가의 세부 정보를 반환하는 많은 기능이 있습니다.
이러한 기능 중 일부는 ListOfCountryNameByCodes
및 ListOfContinentsByName
입니다. 다음에서는 ListOfCountryNameByCodes
를 사용하여 세계의 국가를 인쇄합니다.
<?php
// A soap client that allows you to view information
// about countries in the world
$soap_client = new SoapClient("http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso?WSDL");
// Use a client function to print country names.
$list_countries_names_by_code = $soap_client->ListOfCountryNamesByCode();
/*
Here is another function you can try out.
$list_of_continents_by_name = $client->ListOfContinentsByName();
*/
// Show the results.
echo "<pre>";
var_dump($list_countries_names_by_code);
echo "</pre>";
?>
출력(다음은 발췌):
<pre>object(stdClass)#2 (1) {
["ListOfCountryNamesByCodeResult"]=>
object(stdClass)#3 (1) {
["tCountryCodeAndName"]=>
array(246) {
[0]=>
object(stdClass)#4 (2) {
["sISOCode"]=>
string(2) "AD"
["sName"]=>
string(7) "Andorra"
}
[1]=>
object(stdClass)#5 (2) {
["sISOCode"]=>
string(2) "AE"
["sName"]=>
string(20) "United Arab Emirates"
}
[2]=>
object(stdClass)#6 (2) {
["sISOCode"]=>
string(2) "AF"
["sName"]=>
string(11) "Afghanistan"
}
....
PHP SOAP 예제 5: NuSOAP
를 사용한 온도 변환
‘NuSOAP’는 웹 서비스에 SOAP 연결을 할 수 있게 해주는 PHP 도구입니다. 작동 모드는 ‘SoapClient’를 사용하는 것과 같지만 다음과 같은 차이점이 있습니다.
nusoap_client
에는 서비스 주소와wsdl
이 인수로 필요합니다.- 작업할 데이터는 XML이어야 합니다.
NuSOAP
를 사용하려면 SourceForge
에서 다운로드하여 현재 작업 디렉토리에 놓으십시오. 그런 다음 온도 변환에 다음 코드를 사용합니다.
앞에서 설명한 예외를 제외하고 단계가 동일함을 알 수 있습니다. 한 가지 더, 코드는 PHP5
에서 작동하고 PHP8
에서는 bool
을 반환합니다.
<?php
// This PHP script use NUSOAP for SOAP connection
// and it works in PHP5 ONLY.
require_once('nusoap.php');
// Specify the WSDL file and initiate a new
// NUSOAP client.
$wsdl = "https://www.w3schools.com/xml/tempconvert.asmx?WSDL";
$nusoap_client = new nusoap_client($wsdl, 'wsdl');
// Call on the required method of the client.
$function_from_client = "CelsiusToFahrenheit";
// We'll save the result of the entire operation
// in an array.
$result = array();
// Temperature for conversion.
$temperature = 86;
// Specify the temperature as an XML file
$temperature_as_xml= '<CelsiusToFahrenheit xmlns="https://www.w3schools.com/xml/">
<Celsius>' . $temperature . '</Celsius>
</CelsiusToFahrenheit>';
// If the function exists in the client, initiate
// the conversion.
if (isset($function_from_client)) {
$result['response'] = $nusoap_client->call($function_from_client, $temperature_as_xml);
}
// Show the results
echo "<pre>" . $temperature . ' Celsius => ' . $result['response']['CelsiusToFahrenheitResult'] . ' Fahrenheit' . "</pre>";
?>
출력:
<pre>86 Celsius => 186.8 Fahrenheit</pre>
보너스 예: cURL
을 사용한 SOAP 호출
cURL
을 사용하여 웹 서비스에 대한 SOAP 호출을 할 수 있습니다. 다시 W3Schools 온도 변환 서비스를 사용합니다.
한편, cURL
을 사용하여 서비스 작업 시 다음을 수행해야 합니다.
- 데이터는
Soap Envelope
에 있어야 합니다. - 콘텐츠 헤더를 브라우저로 보내야 합니다.
cURL
연결 옵션을 정의해야 합니다.
다음 코드에서 이 모든 작업과 그 이상을 수행합니다. 코드 주석은 각 단계에서 진행되는 작업을 자세히 설명합니다.
<?php
// This script allows you to use w3schools
// convert via curl.
$webservice_address = "https://www.w3schools.com/xml/tempconvert.asmx";
// The number you'll like to convert, in this case
// we'll like to convert a temperature in celsius
// fahrenheit.
$temperature = 56;
// Pass the temperature as an XML file.
// The XML format is based on the official
// format for the SOAP client by w3schools.
// You can find more at:
$temperature_as_xml = '<?xml version="1.0" encoding="utf-8"?>
<soap12:Envelope
xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="https://www.w3.org/2001/XMLSchema"
xmlns:soap12="https://www.w3.org/2003/05/soap-envelope">
<soap12:Body>
<CelsiusToFahrenheit xmlns="https://www.w3schools.com/xml/">
<Celsius>' . $temperature . '</Celsius>
</CelsiusToFahrenheit>
</soap12:Body>
</soap12:Envelope>';
// Define content headers
$content_headers = array(
'Content-Type: text/xml; charset=utf-8',
'Content-Length: '.strlen($temperature_as_xml)
);
// Initiate the CURL connection and define
// the connection options.
$init_curl_connection = curl_init($webservice_address);
curl_setopt($init_curl_connection, CURLOPT_POST, true);
curl_setopt($init_curl_connection, CURLOPT_HTTPHEADER, $content_headers);
curl_setopt($init_curl_connection, CURLOPT_POSTFIELDS, $temperature_as_xml);
curl_setopt($init_curl_connection, CURLOPT_RETURNTRANSFER, true);
curl_setopt($init_curl_connection, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($init_curl_connection, CURLOPT_SSL_VERIFYPEER, false);
// Use the curl_exec function to get the
// received data.
$recieved_data = curl_exec($init_curl_connection);
// Save the connection result in another variable.
// This will allow us to use it in an "if" statement
// without causing ambiguity.
$connection_result = $recieved_data;
// Check if there is an error.
if ($connection_result === FALSE) {
printf("CURL error (#%d): %s<br>\n", curl_errno($init_curl_connection),
htmlspecialchars(curl_error($init_curl_connection)));
}
// Close the CURL connection
curl_close ($init_curl_connection);
// Show the received data.
echo $temperature . ' Celsius => ' . $recieved_data . ' Fahrenheit';
?>
출력(브라우저에서):
56 Celsius => 132.8 Fahrenheit
출력(상세 버전):
Celsius => <?xml version="1.0" encoding="utf-8"?>
<soap:Envelope xmlns:soap="https://www.w3.org/2003/05/soap-envelope" xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="https://www.w3.org/2001/XMLSchema">
<soap:Body>
<CelsiusToFahrenheitResponse xmlns="https://www.w3schools.com/xml/">
<CelsiusToFahrenheitResult>132.8</CelsiusToFahrenheitResult>
</CelsiusToFahrenheitResponse><
/soap:Body></soap:Envelope>Fahrenheit
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn