PHP での SoapClient の使用例
- PHP の Web サービスから関数と型を取得する
- PHP SOAP の例 1:温度変換
- PHP SOAP の例 2: Say Hello
- PHP SOAP の例 3:単純な演算
- PHP SOAP の例 4:国情報
-
PHP SOAP の例 5:
NuSOAP
を使用した温度変換 -
ボーナスの例:
cURL
を使用した SOAP 呼び出し
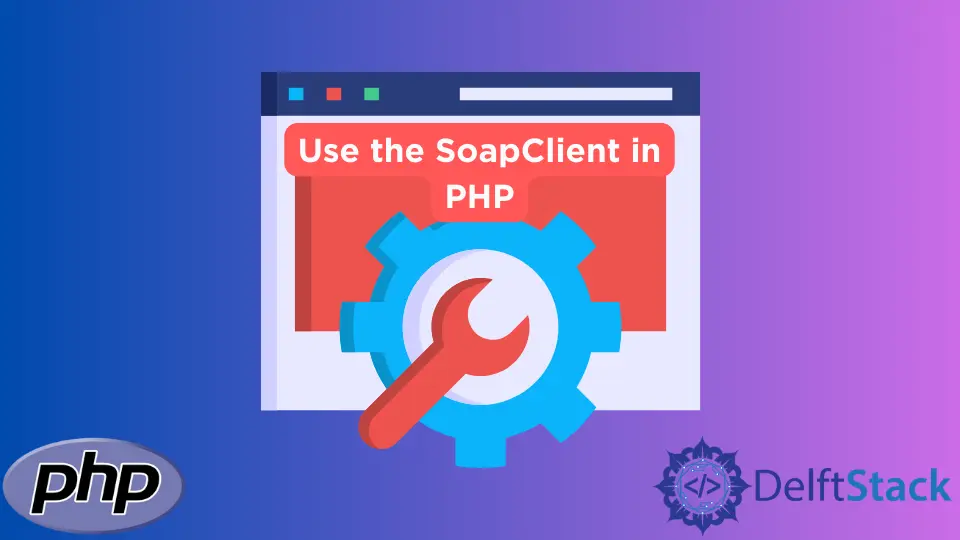
この記事では、PHP SoapClient
を使用して WSDL ファイルを操作する 6つの例を紹介します。その前に、Web サービスから関数と型を取得する方法について説明します。
これは、Web サービスを使用する場合に必須です。
PHP の Web サービスから関数と型を取得する
Web サービスを使用する前に最初に行う必要があるのは、その機能とタイプを知ることです。これは、サービス関数がサービスからデータを返すためです。
そして型は、その関数に供給するかもしれない引数のデータ型です。ここでかもしれない
と言ったのは、後でわかることですが、すべてのサービス関数が引数を必要とするわけではないからです。
PHP では、以下を使用して、サービスから関数と型を取得できます。サービス URL を別の URL に置き換えると、PHP は関数の名前とタイプを返します。
コードが機能するには、インターネットに接続する必要があります。
<?php
$client = new SoapClient("http://www.dneonline.com/calculator.asmx?WSDL");
echo "<b>The functions are:</b> <br/>";
echo "<pre>";
var_dump($client->__getFunctions());
echo "</pre>";
echo "<b>The types are:</b> <br />";
echo "<pre>";
var_dump($client->__getTypes());
echo "</pre>";
?>
出力(以下は抜粋です):
<b>The functions are:</b> <br/><pre>array(8) {
[0]=>
string(32) "AddResponse Add(Add $parameters)"
[1]=>
string(47) "SubtractResponse Subtract(Subtract $parameters)"
[2]=>
string(47) "MultiplyResponse Multiply(Multiply $parameters)"
[3]=>
string(41) "DivideResponse Divide(Divide $parameters)"
[4]=>
string(32) "AddResponse Add(Add $parameters)"
[5]=>
string(47) "SubtractResponse Subtract(Subtract $parameters)"
[6]=>
string(47) "MultiplyResponse Multiply(Multiply $parameters)"
[7]=>
string(41) "DivideResponse Divide(Divide $parameters)"
}
</pre><b>The types are:</b> <br /><pre>array(8) {
[0]=>
string(36) "struct Add {
int intA;
int intB;
}"
[1]=>
string(38) "struct AddResponse {
int AddResult;
}"
[2]=>
string(41) "struct Subtract {
int intA;
int intB;
}"
..........
ノート:
types
の下に、関数に定義されたパラメーターのデータ型があります。- コードで関数に引数を指定するときは、データ型が同じである必要があります。
- 型定義に
int intA
のようなものが表示される場合、引数はintA
という名前の整数である必要があります。
サービス関数とタイプを取得する方法がわかったので、サービスの操作を開始できます。
PHP SOAP の例 1:温度変換
W3schools は、温度コンバーターの WSDL ファイルを提供します。PHP から、このファイルに接続して温度変換を実行できます。
これ(および SoapClient
を使用したその他の SOAP 操作)の手順は次のとおりです。
-
新しい
SoapClient
オブジェクトを作成します。 -
使用するデータを定義します。このデータは、サービスの機能が処理できる形式である必要があります。
-
サービスの関数を呼び出します。
-
結果を表示します。
これらの手順は、次のコードで実装されています。
<?php
// Create the client object and pass in the
// URL of the SOAP server.
$soap_client = new SoapClient('https://www.w3schools.com/xml/tempconvert.asmx?WSDL');
// Create an associative array of the data
// that you'll pass into one of the functions
// of the client.
$degrees_in_celsius = array('Celsius' => '25');
// Use a function of the client for the
// conversion.
$convert_to_fahrenheit = $soap_client->CelsiusToFahrenheit($degrees_in_celsius);
// Show the result using var_dump. Other PHP
// functions like echo will not work.
echo "<pre>";
var_dump($convert_to_fahrenheit);
echo "</pre>";
// Repeat the same process. This time, use the
// FahrenheitToCelsius function to convert a
// temperature from Fahrenheit to Celsius.
$degrees_in_fahrenheit = array('Fahrenheit' => '25');
$convert_to_celsius = $soap_client->FahrenheitToCelsius($degrees_in_fahrenheit);
echo "<pre>";
var_dump($convert_to_celsius);
echo "</pre>";
?>
出力:
<pre>object(stdClass)#2 (1) {
["CelsiusToFahrenheitResult"]=>
string(2) "77"
}
</pre><pre>object(stdClass)#3 (1) {
["FahrenheitToCelsiusResult"]=>
string(17) "-3.88888888888889"
}
</pre>
PHP SOAP の例 2: Say Hello
2 番目の例は、learnwebservices.com
を使用した sayhello
メッセージです。SoapClient
を使用してサービスへの SOAP 呼び出しを開始するだけです。
その後、SayHello
メソッドを呼び出して、希望の名前を渡します。次に、Message
メソッドにアクセスしてメッセージを表示できます。
メッセージは Hello your_desired_name!
である必要があります。たとえば、your_desired_name
が Martinez
の場合、Hello Martinez!
の出力が得られます。
これは、次のコードで行ったことです。
<?php
$soap_client = new SoapClient('https://apps.learnwebservices.com/services/hello?wsdl');
// Call a function of the soap client.
$say_hello = $soap_client->SayHello(['Name' => 'Martinez']);
// Print the message from the client.
echo $say_hello->Message;
?>
出力:
Hello Martinez!
PHP SOAP の例 3:単純な演算
この例では、簡単な計算を実行できる Web サービスを使用しています。このような算術には、加算、除算、乗算、および減算が含まれます。
ただし、ここでは、サービスを使用して追加および分割する方法のみを示します。サービスについての理解が深まるので、残りを試してみてください。
このサービスの算術関数では、番号に名前を付ける必要があります。これらの名前は intA
と intB
であるため、簡単にするためにこれらの名前を連想配列の番号に割り当てます。
その後、この配列をサービスの追加
および分割
関数に渡します。次のコードは、これらすべての動作と計算結果を示しています。
<?php
// Connect to a SOAP client that allows you
// to do basic calculations.
$soap_client = new SoapClient("http://www.dneonline.com/calculator.asmx?WSDL");
// Define data that'll use with the client. This
// time the client requires that arguments that
// you'll pass in has the name intA and intB.
// Therefore, we use that name in our array.
$numbers_for_the_client = array("intA" => 222, "intB" => 110);
$add_the_numbers = $soap_client->Add($numbers_for_the_client);
$divide_the_numbers = $soap_client->Divide($numbers_for_the_client);
// Show the results of the addition and
// division of numbers.
echo "<pre>";
var_dump($add_the_numbers);
var_dump($divide_the_numbers);
echo "</pre>";
?>
出力:
<pre>object(stdClass)#2 (1) {
["AddResult"]=>
int(332)
}
object(stdClass)#3 (1) {
["DivideResult"]=>
int(2)
}
</pre>
PHP SOAP の例 4:国情報
国に関する特定の詳細を取得したい場合は、この例のサービスが適しています。このサービスには、国の詳細を返す多くの機能があります。
これらの関数のいくつかは、ListOfCountryNameByCodes
と ListOfContinentsByName
です。以下では、ListOfCountryNameByCodes
を使用して世界の国を印刷します。
<?php
// A soap client that allows you to view information
// about countries in the world
$soap_client = new SoapClient("http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso?WSDL");
// Use a client function to print country names.
$list_countries_names_by_code = $soap_client->ListOfCountryNamesByCode();
/*
Here is another function you can try out.
$list_of_continents_by_name = $client->ListOfContinentsByName();
*/
// Show the results.
echo "<pre>";
var_dump($list_countries_names_by_code);
echo "</pre>";
?>
出力(以下は抜粋です):
<pre>object(stdClass)#2 (1) {
["ListOfCountryNamesByCodeResult"]=>
object(stdClass)#3 (1) {
["tCountryCodeAndName"]=>
array(246) {
[0]=>
object(stdClass)#4 (2) {
["sISOCode"]=>
string(2) "AD"
["sName"]=>
string(7) "Andorra"
}
[1]=>
object(stdClass)#5 (2) {
["sISOCode"]=>
string(2) "AE"
["sName"]=>
string(20) "United Arab Emirates"
}
[2]=>
object(stdClass)#6 (2) {
["sISOCode"]=>
string(2) "AF"
["sName"]=>
string(11) "Afghanistan"
}
....
PHP SOAP の例 5:NuSOAP
を使用した温度変換
NuSOAP
は、Web サービスへの SOAP 接続を可能にする PHP ツールです。動作モードは SoapClient
を使用するのと似ていますが、次の違いがあります。
nusoap_client
には、引数としてサービスアドレスとwsdl
が必要です。- 作業するデータは XML である必要があります。
NuSOAP
を使用するには、SourceForge
からダウンロードして、現在の作業ディレクトリにドロップします。次に、温度変換に次のコードを使用します。
前に詳述した例外を除いて、手順は同じであることがわかります。もう 1つ、コードは PHP5
で機能し、PHP8
で bool
を返します。
<?php
// This PHP script use NUSOAP for SOAP connection
// and it works in PHP5 ONLY.
require_once('nusoap.php');
// Specify the WSDL file and initiate a new
// NUSOAP client.
$wsdl = "https://www.w3schools.com/xml/tempconvert.asmx?WSDL";
$nusoap_client = new nusoap_client($wsdl, 'wsdl');
// Call on the required method of the client.
$function_from_client = "CelsiusToFahrenheit";
// We'll save the result of the entire operation
// in an array.
$result = array();
// Temperature for conversion.
$temperature = 86;
// Specify the temperature as an XML file
$temperature_as_xml= '<CelsiusToFahrenheit xmlns="https://www.w3schools.com/xml/">
<Celsius>' . $temperature . '</Celsius>
</CelsiusToFahrenheit>';
// If the function exists in the client, initiate
// the conversion.
if (isset($function_from_client)) {
$result['response'] = $nusoap_client->call($function_from_client, $temperature_as_xml);
}
// Show the results
echo "<pre>" . $temperature . ' Celsius => ' . $result['response']['CelsiusToFahrenheitResult'] . ' Fahrenheit' . "</pre>";
?>
出力:
<pre>86 Celsius => 186.8 Fahrenheit</pre>
ボーナスの例:cURL
を使用した SOAP 呼び出し
cURL
を使用して、Web サービスへの SOAP 呼び出しを行うことができます。ここでも、W3Schools 温度変換サービスを使用します。
一方、cURL
では、サービスを操作するときに次のことを行う必要があります。
- データは
石鹸封筒
にある必要があります。 - コンテンツヘッダーをブラウザに送信する必要があります。
cURL
接続オプションを定義する必要があります。
次のコードでは、これらすべてとそれ以上のことを行います。コードコメントは、各ステップで何が起こっているかを詳しく説明しています。
<?php
// This script allows you to use w3schools
// convert via curl.
$webservice_address = "https://www.w3schools.com/xml/tempconvert.asmx";
// The number you'll like to convert, in this case
// we'll like to convert a temperature in celsius
// fahrenheit.
$temperature = 56;
// Pass the temperature as an XML file.
// The XML format is based on the official
// format for the SOAP client by w3schools.
// You can find more at:
$temperature_as_xml = '<?xml version="1.0" encoding="utf-8"?>
<soap12:Envelope
xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="https://www.w3.org/2001/XMLSchema"
xmlns:soap12="https://www.w3.org/2003/05/soap-envelope">
<soap12:Body>
<CelsiusToFahrenheit xmlns="https://www.w3schools.com/xml/">
<Celsius>' . $temperature . '</Celsius>
</CelsiusToFahrenheit>
</soap12:Body>
</soap12:Envelope>';
// Define content headers
$content_headers = array(
'Content-Type: text/xml; charset=utf-8',
'Content-Length: '.strlen($temperature_as_xml)
);
// Initiate the CURL connection and define
// the connection options.
$init_curl_connection = curl_init($webservice_address);
curl_setopt($init_curl_connection, CURLOPT_POST, true);
curl_setopt($init_curl_connection, CURLOPT_HTTPHEADER, $content_headers);
curl_setopt($init_curl_connection, CURLOPT_POSTFIELDS, $temperature_as_xml);
curl_setopt($init_curl_connection, CURLOPT_RETURNTRANSFER, true);
curl_setopt($init_curl_connection, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($init_curl_connection, CURLOPT_SSL_VERIFYPEER, false);
// Use the curl_exec function to get the
// received data.
$recieved_data = curl_exec($init_curl_connection);
// Save the connection result in another variable.
// This will allow us to use it in an "if" statement
// without causing ambiguity.
$connection_result = $recieved_data;
// Check if there is an error.
if ($connection_result === FALSE) {
printf("CURL error (#%d): %s<br>\n", curl_errno($init_curl_connection),
htmlspecialchars(curl_error($init_curl_connection)));
}
// Close the CURL connection
curl_close ($init_curl_connection);
// Show the received data.
echo $temperature . ' Celsius => ' . $recieved_data . ' Fahrenheit';
?>
出力(ブラウザ内):
56 Celsius => 132.8 Fahrenheit
出力(詳細バージョン):
Celsius => <?xml version="1.0" encoding="utf-8"?>
<soap:Envelope xmlns:soap="https://www.w3.org/2003/05/soap-envelope" xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="https://www.w3.org/2001/XMLSchema">
<soap:Body>
<CelsiusToFahrenheitResponse xmlns="https://www.w3schools.com/xml/">
<CelsiusToFahrenheitResult>132.8</CelsiusToFahrenheitResult>
</CelsiusToFahrenheitResponse><
/soap:Body></soap:Envelope>Fahrenheit
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn