How to Replace Space With Dash in PHP
-
Replace Space With Dash Using the
str_replace()
Function in PHP -
Replace Space With Dash Using the
str_ireplace()
Function in PHP -
Replace Space With Dash Using the
preg_replace()
Function in PHP
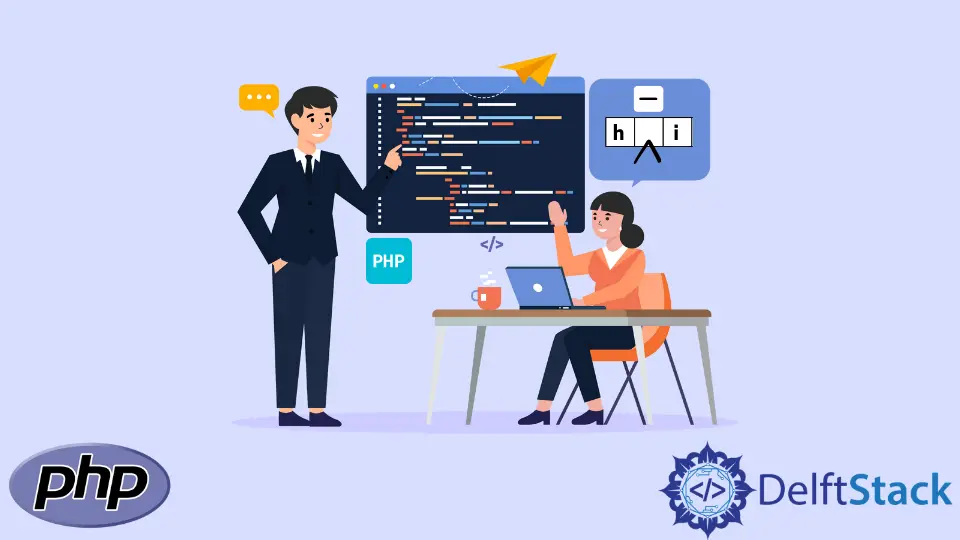
PHP provides three functions to replace string/array with another string/array in the string/array.
This article will introduce all these functions to replace space(" "
) with dash(-
).
Replace Space With Dash Using the str_replace()
Function in PHP
The str_replace()
function is an integrated PHP function that will replace all occurrences of the search string with the replacement string. It returns a string or an array depending on the subject passed where all occurrences of search
in subject
are replaced by the given replace
value. This function is case-sensitive, which means search
is not equal to SEARCH
. This function does not support the regex; if you want regex to get replaced, use preg_replace()
.
Syntax of str_replace()
str_replace(
array|string $search,
array|string $replace,
string|array $subject,
int &$count = null
): string|array
Parameters of str_replace()
This function accepts 4 parameters out of which three are obligatory and 1 is non-compulsory.
$searchVal
: This parameter is often of both string and array types. It specifies the string that is to be replaced by thereplaceVal
.$replaceVal
: This parameter is often of both string and array types. It specifies the string with which you want to replace the$searchVal
.$subjectVal
: This parameter is often of both string and array types. It includes the string or array of strings on which you want to perform search & replace.$count
: It is a non-compulsory parameter. If passed, its value will be set to the total number of replacement operations performed on the string$subjectVal
.
If search
and replace
are arrays, then str_replace()
takes a value from each array and uses them to search and replace the subject. An empty string will be used for the rest of the replacement values if replace
has fewer values than the search. If a search
is an array and replace
is a string, then the replacement string is used for each search
value. If search
or replace
are both arrays, PHP will process their elements first to last.
Return Values
It returns a string or an array relying on the subject handed with the replace values.
Example Code
<?php
$subjectVal = "It was nice sunny day.";
$resStr = str_replace(' ', '-', $subjectVal);
print_r($resStr);
?>
Output:
It-was-nice-sunny-day.
Replace Space With Dash Using the str_ireplace()
Function in PHP
It returns a string or an array with all occurrences of search
in subject
(ignoring case) replaced with the given replace
value. It’s a case-insensitive model of str_replace()
.
Syntax of str_ireplace()
str_ireplace(
array|string $search,
array|string $replace,
string|array $subject,
int &$count = null
): string|array
Example Code
<?php
$subjectVal = "It was nice sunny day.";
$resStr2 = str_ireplace(' ', '-', $subjectVal);
print_r($resStr2);
?>
Output:
It-was-nice-rainy-day.
Replace Space With Dash Using the preg_replace()
Function in PHP
The preg_replace()
function is a PHP in-built function that is used to perform a regular expression for search
and replace
the content.
Syntax of preg_replace()
preg_replace(
string|array $pattern,
string|array $replacement,
string|array $subject,
int $limit = -1,
int &$count = null
): string|array|null
Parameters
$pattern
: This parameter is often of both string and array of strings. It contains the string element, which is used to search the content.$replacement
: It is an obligatory parameter that specifies the string or an array with strings to replace. If this parameter is a string and the pattern parameter is an array, all patterns will get replaced by that string. If both pattern and replacement parameters are arrays, each pattern will be replaced by the replacement counterpart. If there are fewer elements within the replacement array than in the pattern array, any extra patterns will be replaced by an empty string.$subject
: This parameter is often of both string and array of strings on which search and replace should be performed. If it is handled as an array, then the search and replacement are performed on each element of the subject; also, the return value is an array.$limit
: This parameter specifies the maximum feasible replacements for every pattern in each subject string; the default value is-1
(no limit).$count
: An non-compulsory parameter, which will be the number of replacements to be done.
Return Values
preg_replace()
returns an array or string relying on the subject parameter passed. If matches are found, the new subject will be returned; otherwise, the subject will be returned unchanged or null if an error occurred.
Example Code:
<?php
$str = "Welcome to PHP";
$str = preg_replace('/\s+/', '-', $str);
echo $str;
?>
output:
Welcome-to--PHP
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn