How to Write Multi-Line Strings in PHP
- Use the Escape Sequence to Write Multi-Line Strings in PHP
- Use the Concatenation Assignment Operator to Write Multi-Line Strings in PHP
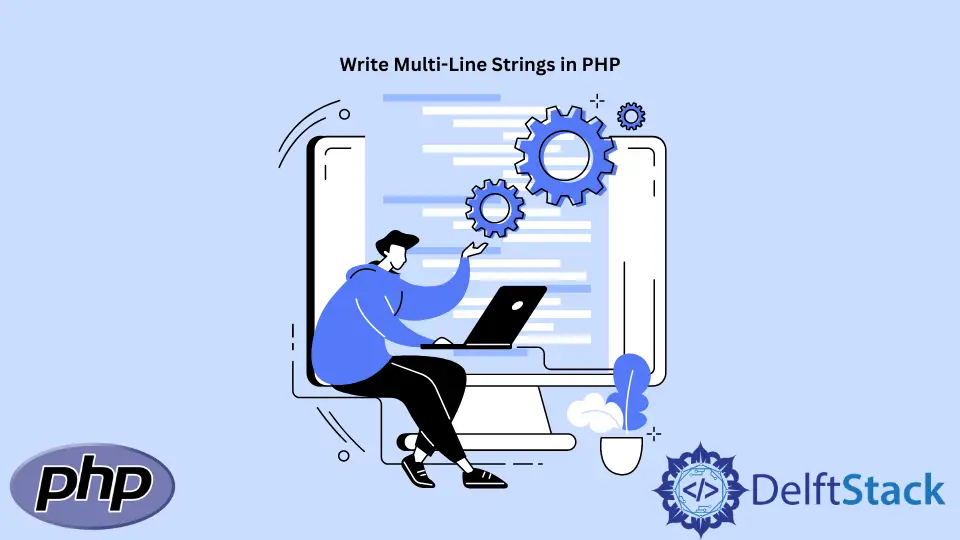
This article will introduce different methods to write multi-line strings in PHP.
Use the Escape Sequence to Write Multi-Line Strings in PHP
In PHP, we have multiple escape sequences. We will mention only two here. The simplest method is to use the \n
escape sequence. We use this escape sequence with double-quotes. The correct syntax to use this escape sequence is as follows.
echo("\n");
The program below shows the way by which we can use the \n
escape sequence to write multi-line strings in PHP.
<?php
echo("This is the first line \nThis is the second line");
?>
Output:
This is the first line
This is the second line
We can use \n
twice to create an empty line between two text lines.
<?php
echo("This is the first line \n\nThis is the third line");
?>
Output:
This is the first line
This is the third line
We can also use the \r\n
escape sequence to write multi-line strings. It creates the new line as well because it is the carriage return. The carriage return resets the pointer and starts it from the left. The correct syntax to use it is as follows:
echo("\r\n");
The program that applies this method to write multi-line strings is as follows:
<?php
echo("This is the first line \r\nThis is the third line");
?>
Output:
This is the first line
This is the third line
Use the Concatenation Assignment Operator to Write Multi-Line Strings in PHP
In PHP, we can also use the concatenation assignment operator to write multi-line strings. The concatenation assignment operator is .=
. The concatenation assignment operator appends the strings on the right side. We will also use PHP_EOL
to create a new line. The correct syntax to use this operator is as follows.
$string1 .= $string2;
The details of these variables are as follows.
Variables | Description |
---|---|
$string1 |
It is the string with which we want to append a new string on the right side. |
$string2 |
It is the string that we want to concatenate with the first string. |
The program below shows the way by which we can use the concatenation assignment operator and PHP_EOL
to write multi-line strings in PHP.
<?php
$mystring1 = "This is the first line." . PHP_EOL;
$mystring2 = "This is the second line";
$mystring1 .= $mystring2;
echo($mystring1);
?>
Output:
This is the first line.
This is the second line
Likewise, we can use this operator to write N multi-line strings.
<?php
$mystring1 = "This is the first line." . PHP_EOL;
$mystring2 = "This is the second line" . PHP_EOL;
$mystring3 = "This is the third line" . PHP_EOL;
$mystring4 = "This is the fourth line" . PHP_EOL;
$mystring5 = "This is the fifth line";
$mystring1 .= $mystring2 .= $mystring3 .= $mystring4 .= $mystring5;
echo($mystring1);
?>
Output:
This is the first line.
This is the second line
This is the third line
This is the fourth line
This is the fifth line