How to Ignore Case Sensitivity When Comparing Strings in PHP
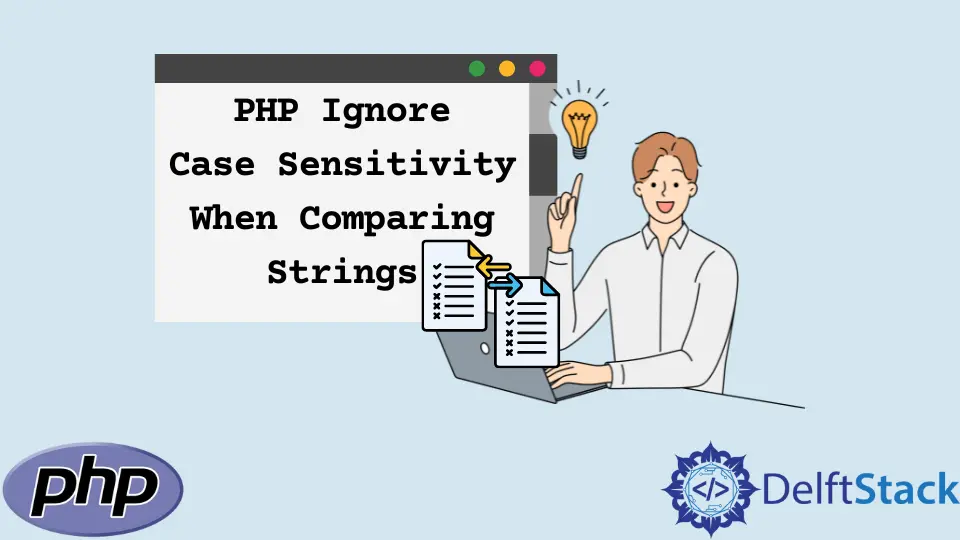
This tutorial demonstrates how to ignore character cases in PHP.
PHP Ignore Case Sensitivity When Comparing Strings
Sometimes when comparing two strings, we need to ignore the case of both strings. PHP provides a built-in method, strcasecmp()
, to compare two strings while ignoring the case.
The strcasecmp()
method takes two parameters; both are strings that will be compared. This method will return the values:
strcasecmp()
will return 0 if the two strings are equal.strcasecmp()
will return <0 if the first string is less than the second string.strcasecmp()
will return >0 if the first string is greater than the second string.
This method only compares the strings and then returns a value. If the strings have only a difference in the case, it will always return 0.
See example:
<?php
$String1 = "This is Delftstack.com";
$String2 = "This is Delftstack.com";
// Both the strings are equal in case
$Result=strcasecmp($String1, $String2);
echo "The result for two equal strings is: ".$Result."<br>";
$String1 = "this is delftstack.com";
$String2 = "THIS IS DELFTSTACK.COM";
// first string is lowercase than the second string
$Result=strcasecmp($String1, $String2);
echo "The result for first string lowercase and second string uppercase is : ".$Result."<br>";
$String1 = "THIS IS DELFTSTACK.COM";
$String2 = "this is delftstack.com";
// first string is uppercase, then the second string
$Result=strcasecmp($String1, $String2);
echo "The result for first string uppercse and second string lowercase is: ".$Result;
?>
The code above will compare the strings while ignoring the case where strings have different cases. See output:
The result for two equal strings is: 0
The result for first string lowercase and second string uppercase is : 0
The result for first string uppercse and second string lowercase is: 0
As we can see for the method strcasecmp()
, all the strings in lowercase, uppercase, and sentence case are equal; that is why it always returns 0. Let’s try an example where the strings are not equal by words or characters:
<?php
$String1 = "delftstack.com";
$String2 = "THIS IS DELFTSTACK.COM";
// first string is lowercase, then the second string
$Result=strcasecmp($String1, $String2);
echo "The result for first string is lower then second string : ".$Result."<br>";
$String1 = "THIS IS DELFTSTACK.COM";
$String2 = "delftstack.com";
// First string is greater then second string
$Result=strcasecmp($String1, $String2);
echo "The result for first string is greater then second string: ".$Result;
?>
The strcasecmp()
method will return a numeric value in a negative or positive number. See output:
The result for first string is lower then second string : -16
The result for first string is greater then second string: 16
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook