How to Get UTC Time in PHP
-
Use
gmdate()
to Get UTC Time -
Use
strtotime()
to Get UTC Time -
Use
date()
to Get UTC Time -
Use
date_default_timezone_set()
to Get UTC Time -
Use
DateTime
Object to Get UTC Time
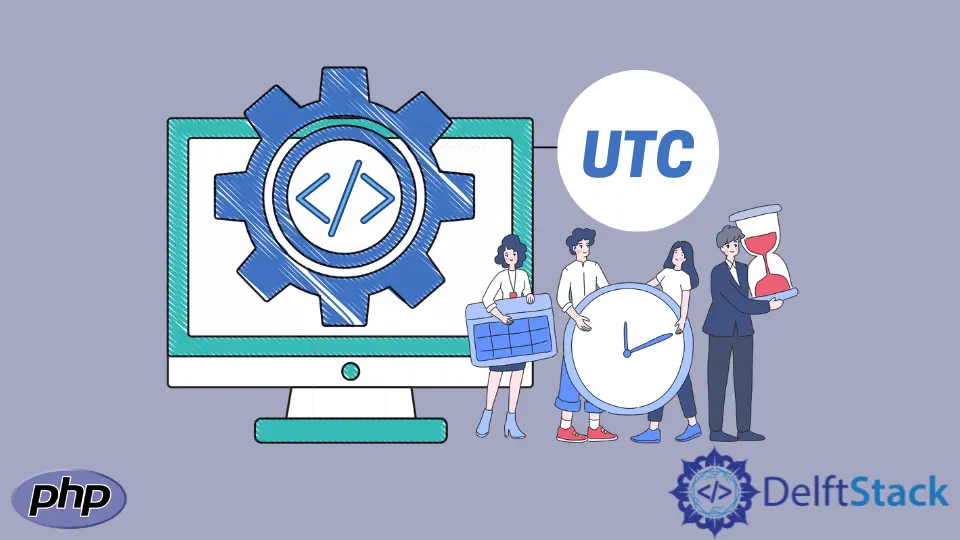
This article teaches you how to get UTC in PHP using five methods. These methods will use date_default_timezone_set()
, gmdate()
, strtotime()
, date()
, and the DateTime
object.
Use gmdate()
to Get UTC Time
The gmdate()
function will format a date and time in UTC. Once you supply gmdate()
with your date time format, e.g. Y-m-d H:i:s
, it’ll show it in UTC.
We’ve set the current time zone in the following code to Detroit, Michigan. This will show the time in Detroit and what it’s like in UTC.
Example Code:
<?php
// Use the time in Detroit, Michigan, as the default
// time.
date_default_timezone_set("America/Detroit");
// We get the current time in Detroit, Michigan.
$time_in_Detroit = date('Y-m-d H:i:s', time());
// We get the UTC time using the gmdate() function
$utc_time = gmdate("Y-m-d H:i:s");
// For comparison, we show the time in Detroit and
// what it'll be in UTC.
echo "The current time in Detroit is: " . $time_in_Detroit . PHP_EOL;
echo "The UTC is: " . $utc_time;
?>
Output (you’ll have different results):
The current time in Detroit is: 2022-07-27 17:32:36
The UTC is: 2022-07-27 21:32:36
Use strtotime()
to Get UTC Time
The method strtotime()
will parse a textual date time into a Unix timestamp. After that, you can do what you want with the timestamp.
In the following code, we get a timestamp from strtotime()
using gmdate()
. Then we pass the timestamp as the second parameter of the date()
function.
Doing this allows you to write your date format and get the result in UTC. For comparison, we’ve set the time zone of the script to Guatemala.
Example Code:
<?php
// Use the time in Guatemala as the default
// time.
date_default_timezone_set("America/Guatemala");
// We get the current time in Guatemala
$time_in_Guatemala = date('Y-m-d H:i:s', time());
// We get the UTC UNIX timestamp
$utc_timestamp = strtotime(gmdate("M d Y H:i:s"));
// We convert the UNIX timestamp to UTC
$utc_time = date('Y-m-d H:i:s', $utc_timestamp);
// For comparison, we show the time in Guatemala
// and what it'll be in UTC.
echo "The time in Guatemala is: " . $time_in_Guatemala . PHP_EOL;
echo "The UTC is: " . $utc_time;
?>
Output (you’ll have different results):
The time in Guatemala is: 2022-07-27 15:48:01
The UTC is: 2022-07-27 21:48:01
Use date()
to Get UTC Time
We’ll use the date()
function to format a date, but with some math, date()
will return the UTC. The math involves subtracting the time zone offset from the epoch time, and then you pass the result as the second parameter of the date()
function.
For comparison, we’ve changed the time zone to Adelaide, Australia. When you run the code below, you’ll see the UTC and what it’s like in Adelaide.
Example Code:
<?php
// Use the time in Adelaide, Australia, as the default
// time.
date_default_timezone_set("Australia/Adelaide");
// We get the current time in Adelaide
$time_in_Adelaide = date('Y-m-d H:i:s', time());
// We get the UTC time using the date() function.
$utc_time = date("Y-m-d H:i:s", time() - date("Z"));
// For comparison, we show the time in Adelaide
// and what it'll be in UTC.
echo "The time in Adelaide is: " . $time_in_Adelaide . PHP_EOL;
echo "The UTC is: " . $utc_time;
?>
Output (you’ll have different results):
The time in Adelaide is: 2022-07-28 07:56:31
The UTC is: 2022-07-27 22:26:31
Use date_default_timezone_set()
to Get UTC Time
The date_default_timezone_set()
function is used to change the time zone. We can also use it to return the UTC by changing its parameter to UTC
.
As a result, it’ll always be in UTC, irrespective of the time in the PHP script.
<?php
// Set the default time zone to UTC and all
// date and time of the current PHP script will
// be in UTC.
date_default_timezone_set("UTC");
// As usual, get the date, but this time, it'll
// return the time in UTC. That's because this script
// has its time zone set to UTC.
$utc_time = date('Y-m-d H:i:s', time());
echo "The UTC is: " . $utc_time;
?>
Output (you’ll have different results):
The UTC is: 2022-07-27 22:36:02
Use DateTime
Object to Get UTC Time
A combination of the DateTime
object and the DateTimeZone
object can return the UTC. To return the UTC, set the first and second parameters of DateTime
to now
and new \DateTimeZone("UTC")
, and then you can show the result in RFC 850 format.
Example Code:
<?php
// Get the UTC using the DateTime object.
// Its first parameter should be the
// string "now". And its second parameter should
// be a new DateTimeZone object and its parameter
// should be the string "UTC".
$utc_time = new \DateTime("now", new \DateTimeZone("UTC"));
// Format the date to show it's a UTC date
// A sample output is: Wednesday, 27-Jul-22 15:02:41 UTC
echo $utc_time->format(\DateTime::RFC850);
?>
Output (you’ll have different results):
Wednesday, 27-Jul-22 23:00:42 UTC
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn