PHP で UTC 時刻を取得する
-
gmdate()
を使用して UTC 時刻を取得する -
strtotime()
を使用して UTC 時刻を取得する -
date()
を使用して UTC 時刻を取得する -
date_default_timezone_set()
を使用して UTC 時刻を取得する -
DateTime
オブジェクトを使用して UTC 時刻を取得する
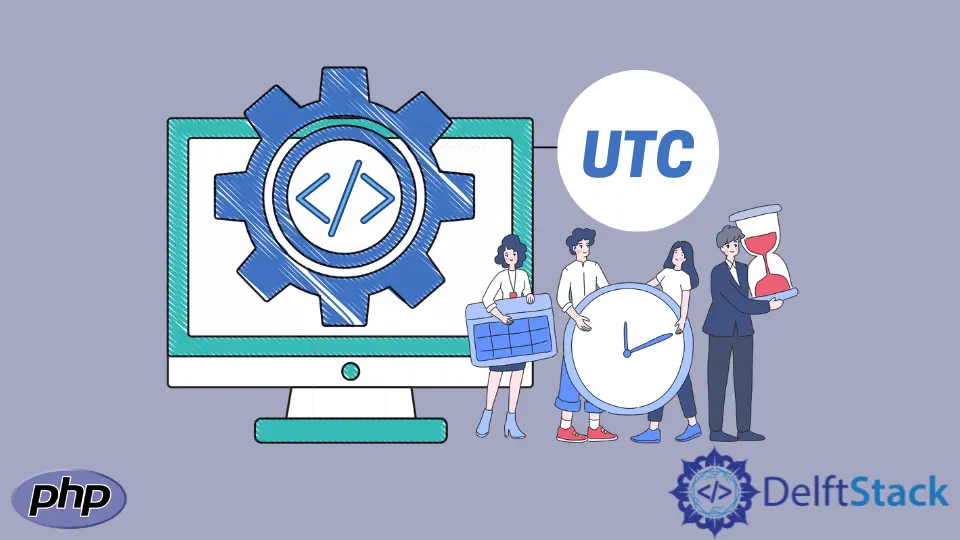
この記事では、5つのメソッドを使用して PHP で UTC を取得する方法を説明します。 これらのメソッドは、date_default_timezone_set()
、gmdate()
、strtotime()
、date()
、および DateTime
オブジェクトを使用します。
gmdate()
を使用して UTC 時刻を取得する
gmdate()
関数は、日付と時刻を UTC でフォーマットします。 gmdate()
に日時形式を指定すると、たとえば Y-m-d H:i:s
、UTC で表示されます。
次のコードでは、現在のタイム ゾーンをミシガン州デトロイトに設定しています。 これにより、デトロイトの時刻と UTC の時刻が表示されます。
コード例:
<?php
// Use the time in Detroit, Michigan, as the default
// time.
date_default_timezone_set("America/Detroit");
// We get the current time in Detroit, Michigan.
$time_in_Detroit = date('Y-m-d H:i:s', time());
// We get the UTC time using the gmdate() function
$utc_time = gmdate("Y-m-d H:i:s");
// For comparison, we show the time in Detroit and
// what it'll be in UTC.
echo "The current time in Detroit is: " . $time_in_Detroit . PHP_EOL;
echo "The UTC is: " . $utc_time;
?>
出力 (異なる結果が得られます):
The current time in Detroit is: 2022-07-27 17:32:36
The UTC is: 2022-07-27 21:32:36
strtotime()
を使用して UTC 時刻を取得する
メソッド strtotime()
は、テキストの日付時刻を Unix タイムスタンプに解析します。 その後、タイムスタンプを使用して必要なことを行うことができます。
次のコードでは、gmdate()
を使用して strtotime()
からタイムスタンプを取得します。 次に、date()
関数の 2 番目のパラメーターとしてタイムスタンプを渡します。
これを行うと、日付形式を記述して結果を UTC で取得できます。 比較のために、スクリプトのタイム ゾーンをグアテマラに設定しました。
コード例:
<?php
// Use the time in Guatemala as the default
// time.
date_default_timezone_set("America/Guatemala");
// We get the current time in Guatemala
$time_in_Guatemala = date('Y-m-d H:i:s', time());
// We get the UTC UNIX timestamp
$utc_timestamp = strtotime(gmdate("M d Y H:i:s"));
// We convert the UNIX timestamp to UTC
$utc_time = date('Y-m-d H:i:s', $utc_timestamp);
// For comparison, we show the time in Guatemala
// and what it'll be in UTC.
echo "The time in Guatemala is: " . $time_in_Guatemala . PHP_EOL;
echo "The UTC is: " . $utc_time;
?>
出力 (異なる結果が得られます):
The time in Guatemala is: 2022-07-27 15:48:01
The UTC is: 2022-07-27 21:48:01
date()
を使用して UTC 時刻を取得する
date()
関数を使用して日付をフォーマットしますが、いくつかの計算を行うと、date()
は UTC を返します。 この計算では、エポック タイムからタイム ゾーン オフセットを減算し、その結果を date()
関数の 2 番目のパラメーターとして渡します。
比較のために、タイム ゾーンをオーストラリアのアデレードに変更しました。 以下のコードを実行すると、UTC とアデレードでの様子が表示されます。
コード例:
<?php
// Use the time in Adelaide, Australia, as the default
// time.
date_default_timezone_set("Australia/Adelaide");
// We get the current time in Adelaide
$time_in_Adelaide = date('Y-m-d H:i:s', time());
// We get the UTC time using the date() function.
$utc_time = date("Y-m-d H:i:s", time() - date("Z"));
// For comparison, we show the time in Adelaide
// and what it'll be in UTC.
echo "The time in Adelaide is: " . $time_in_Adelaide . PHP_EOL;
echo "The UTC is: " . $utc_time;
?>
出力 (異なる結果が得られます):
The time in Adelaide is: 2022-07-28 07:56:31
The UTC is: 2022-07-27 22:26:31
date_default_timezone_set()
を使用して UTC 時刻を取得する
date_default_timezone_set()
関数は、タイム ゾーンを変更するために使用されます。 パラメータをUTC
に変更することで、UTCを返すために使用することもできます。
その結果、PHP スクリプトの時刻に関係なく、常に UTC になります。
<?php
// Set the default time zone to UTC and all
// date and time of the current PHP script will
// be in UTC.
date_default_timezone_set("UTC");
// As usual, get the date, but this time, it'll
// return the time in UTC. That's because this script
// has its time zone set to UTC.
$utc_time = date('Y-m-d H:i:s', time());
echo "The UTC is: " . $utc_time;
?>
出力 (異なる結果が得られます):
The UTC is: 2022-07-27 22:36:02
DateTime
オブジェクトを使用して UTC 時刻を取得する
DateTime
オブジェクトと DateTimeZone
オブジェクトの組み合わせは、UTC を返すことができます。 UTC を返すには、DateTime
の 1 番目と 2 番目のパラメーターを now
と new \DateTimeZone("UTC")
に設定すると、結果を RFC 850 形式で表示できます。
コード例:
<?php
// Get the UTC using the DateTime object.
// Its first parameter should be the
// string "now". And its second parameter should
// be a new DateTimeZone object and its parameter
// should be the string "UTC".
$utc_time = new \DateTime("now", new \DateTimeZone("UTC"));
// Format the date to show it's a UTC date
// A sample output is: Wednesday, 27-Jul-22 15:02:41 UTC
echo $utc_time->format(\DateTime::RFC850);
?>
出力 (異なる結果が得られます):
Wednesday, 27-Jul-22 23:00:42 UTC
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn