How to Get Time Difference in Minutes in PHP
-
Use
date_diff()
Function to Get Time Difference in Minutes in PHP - Use Mathematical Formulas to Get Time Difference in Minutes in PHP
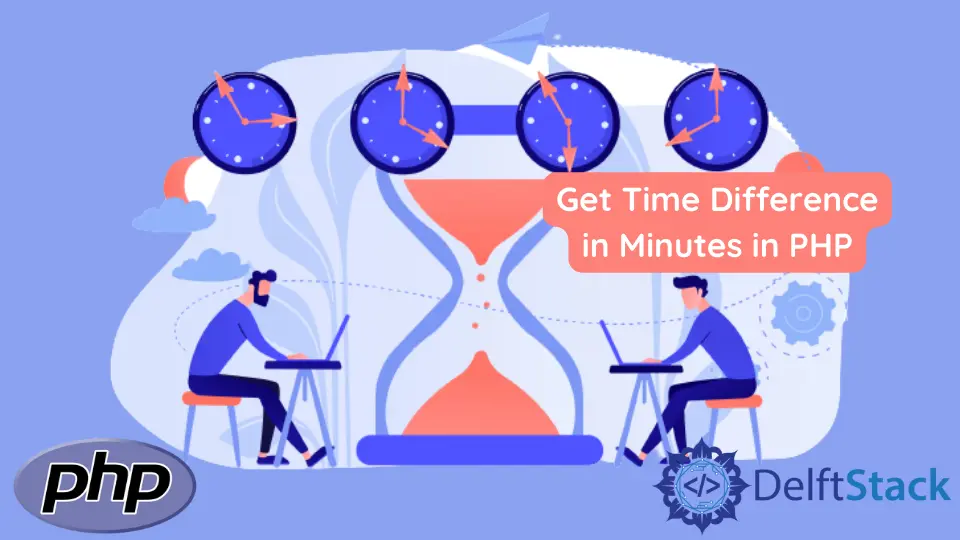
In this article, we will introduce methods to get time difference in minutes in PHP.
- Using
date_diff()
function - Using mathematical formula
Use date_diff()
Function to Get Time Difference in Minutes in PHP
We will use the built-in function date_diff()
to get time difference in minutes.
For this, we need a start date and an end date. We will calculate their time difference in minutes using the date_diff()
function. The correct syntax to use this function is as follows.
date_diff($DateTimeObject1, $DateTimeObject2);
The built-in function date_diff()
has two parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$DateTimeObject1 |
mandatory | It is a DateTime object. It represents the start date. |
$DateTimeObject2 |
mandatory | It is also a DateTime object, It represents the end date. |
This function returns the difference between the start date and end date on success. It returns FALSE on failure.
The program below shows how we can use the date_diff()
function to get time difference in minutes.
<?php
$dateTimeObject1 = date_create('2019-06-16');
$dateTimeObject2 = date_create('2020-06-16');
$difference = date_diff($dateTimeObject1, $dateTimeObject2);
echo ("The difference in days is:");
echo $difference->format('%R%a days');
echo "\n";
$minutes = $difference->days * 24 * 60;
$minutes += $difference->h * 60;
$minutes += $difference->i;
echo("The difference in minutes is:");
echo $minutes.' minutes';
?>
The function date_diff()
has returned an object that represents the difference between two dates.
Output:
The difference in days is:+366 days
The difference in minutes is:527040 minutes
Now we will find the difference between time.
<?php
$dateTimeObject1 = date_create('17:13:00');
$dateTimeObject2 = date_create('12:13:00');
$difference = date_diff($dateTimeObject1, $dateTimeObject2);
echo ("The difference in hours is:");
echo $difference->h;
echo "\n";
$minutes = $difference->days * 24 * 60;
$minutes += $difference->h * 60;
$minutes += $difference->i;
echo("The difference in minutes is:");
echo $minutes.' minutes';
?>
Output:
The difference in hours is:5
The difference in minutes is:300 minutes
Use Mathematical Formulas to Get Time Difference in Minutes in PHP
In PHP, we can also use different mathematical formulas to get time difference in minutes. The program that gets time difference in minutes is as follows:
<?php
$to_time = strtotime("10:42:00");
$from_time = strtotime("10:21:00");
$minutes = round(abs($to_time - $from_time) / 60,2);
echo("The difference in minutes is: $minutes minutes.");
?>
Output:
The difference in minutes is: 21 minutes
We can also find the time difference in minutes using the following method.
<?php
$start = strtotime('12:01:00');
$end = strtotime('13:16:00');
$minutes = ($end - $start) / 60;
echo "The difference in minutes is $minutes minutes.";
?>
Output:
The difference in minutes is 75 minutes.