How to Get Parameters From a URL String in PHP
-
Use
parse_url()
andparse_str()
Functions to Get Parameters From a URL String in PHP -
Use
$_GET
Variable to Get Parameters From a URL String in PHP
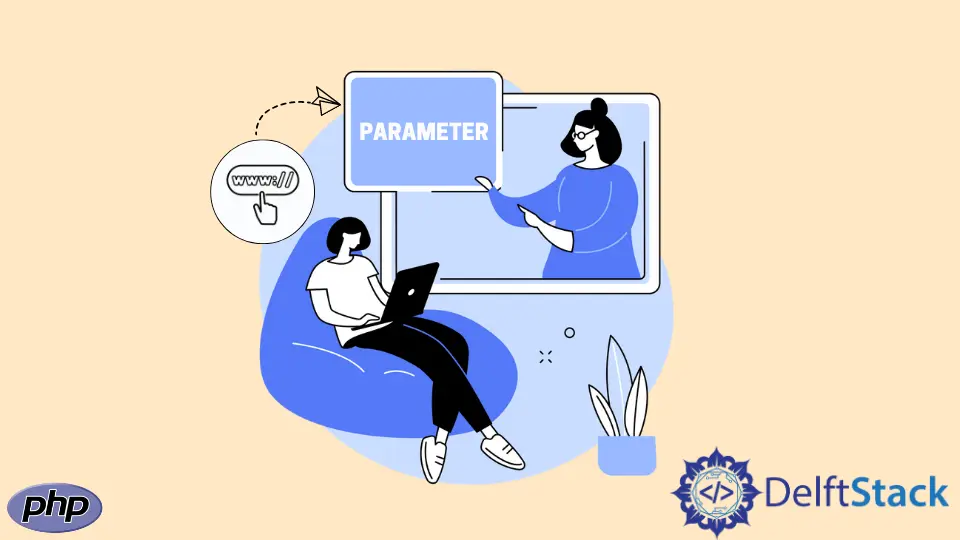
This article will introduce methods to get parameters from a URL string
in PHP.
- Using
parse_url()
function - Using
parse_str()
function - Using
$_GET
variable
Use parse_url()
and parse_str()
Functions to Get Parameters From a URL String in PHP
We can use the built-in functions parse_url()
and parse_str()
functions to get parameters from a URL string
. These functions are used together to get the parameters. The function parse_url
will divide the URL into different parameters. After that parse_string
will get the required parameter.
The correct syntax to use parse_url()
functions is as follows
parse_url( $url, $component);
The built-in function parse_url
has two parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$url |
mandatory | It is the URL we want to get parameter from. |
$component |
optional | It is used to get a specific URL component. |
This function returns components of a URL. If our URL is not correctly formatted, the function may return False
.
The correct syntax to use the parse_str()
function is as follows
parse_str( $string, $result);
The built-in function parse_str
has two parameters. The details of its parameters are as follows.
Parameters | Description | |
---|---|---|
$string |
mandatory | It is the input string that is converted to the variables. |
$result |
mandatory | If this parameter is passed, the variables are stored in it. |
This function returns nothing.
The program below shows how we can use these functions to get parameters from a URL string
.
<?php
$url = "https://testurl.com/test/1234?email=abc@test.com&name=sarah";
$components = parse_url($url);
parse_str($components['query'], $results);
print_r($results);
?>
Output:
Array
(
[email] => abc@test.com
[name] => sarah
)
Now, if we want to get the email parameter only, we will do the following changes in our program:
<?php
$url = "https://testurl.com/test/1234?email=abc@test.com&name=sarah";
$components = parse_url($url);
parse_str($components['query'], $results);
echo($results['email']);
?>
Output:
abc@test.com
We can also pass $component
parameter to parse_url()
function.
<?php
$url = "https://testurl.com/test/1234?email=abc@test.com&name=sarah";
$components = parse_url($url, PHP_URL_QUERY);
//$component parameter is PHP_URL_QUERY
parse_str($components, $results);
print_r($results);
?>
Output:
Array
(
[email] => abc@test.com
[name] => sarah
)
Use $_GET
Variable to Get Parameters From a URL String in PHP
In PHP, we can also use the $_GET
variable to get parameters from a URL string. It is a superglobals
variable, which means that it is available in all scopes. This variable gets the data i.e URL, from an HTML form.
Assuming that the URL from form is https://testurl.com/test/1234?email=abc@test.com&name=sarah
. The program that uses this variable to get parameters from a URL string
is as follows.
<?php
echo $_GET['email'] . $_GET['name']
?>
Output:
abc@test.com
sarah