How to Extract Numbers From a String in PHP
-
Use
preg_match_all()
Function to Extract Numbers From a String in PHP -
Use
filter_var()
Function to Extract Numbers From a String in PHP -
Use
preg_replace()
Function to Extract Numbers From a String in PHP
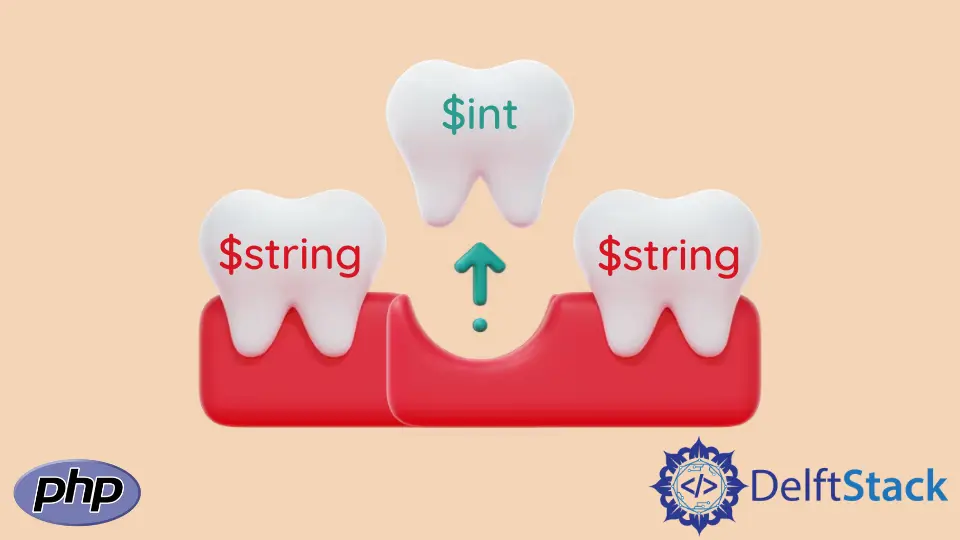
In this article, we will introduce methods to extract numbers from a string
in PHP.
- Using
preg_match_all()
function - Using
filter_variable()
function - Using
preg_replace()
function
Use preg_match_all()
Function to Extract Numbers From a String in PHP
We can use the built-in function preg_match_all()
to extract numbers from a string
. This function globally searches a specified pattern from a string
. The correct syntax to use this function is as follows:
preg_match_all($pattern, $inputString, $matches, $flag, $offset);
The built-in function preg_match_all()
has five parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$pattern |
mandatory | It is the pattern that we want to check in the given string . |
$inputString |
mandatory | It is the string for which we want to search the given pattern. |
$matches |
optional | If this parameter is given, then the function stores the result of the matching process in it. |
$flags |
optional | This parameter has two options: PREG_OFFSET_CAPTURE and PREG_UNMATCHED_AS_NULL . You can read its description here. |
$offset |
optional | It tells the function of where to start the matching process. Usually, the search starts from the beginning. |
This function returns a Boolean
variable. It returns true if the given pattern exists.
The program below shows how we can use the preg_match_all()
function to extract numbers from a given string
.
<?php
$string = 'Sarah has 4 dolls and 6 bunnies.';
preg_match_all('!\d+!', $string, $matches);
print_r($matches);
?>
We have used !\d+!
pattern to extract numbers from the string
.
Output:
Array
(
[0] => Array
(
[0] => 4
[1] => 6
)
)
Use filter_var()
Function to Extract Numbers From a String in PHP
We can also use the filter_var()
function to extract numbers from a string
. The correct syntax to use this function is as follows:
filter_var($variableName, $filterName, $options)
The function filter_var()
accepts three parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$variableName |
mandatory | It is the variable to filter. |
$filterName |
mandatory | It is the name of filter which will be applied to the variable. By default, it is FILTER_DEFAULT |
$options |
mandatory | This parameter tells about the options to use. |
We have used FILTER_SANITIZE_NUMBER_INT
filter. The program that extracts numbers from the string
is as follows:
<?php
$string = 'Sarah has 4 dolls and 6 bunnies.';
$int = (int) filter_var($string, FILTER_SANITIZE_NUMBER_INT);
echo("The extracted numbers are: $int \n");
?>
Output:
The extracted numbers are: 46
Use preg_replace()
Function to Extract Numbers From a String in PHP
In PHP, we can also use the preg_replace()
function to extract numbers from a string
. The correct syntax to use this function is as follows:
preg_replace($regexPattern, $replacementVar, $original, $limit, $count)
The function preg_replace()
accepts five parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$regexPattern |
mandatory | It is the pattern we will search in the original string or array. |
$replacementVar |
mandatory | It is the string or array which we use as a replacement for the searched value. |
$original |
mandatory | It is the string or an array from which we want to find value and replace it. |
$limit |
optional | This parameter limits the number of replacements. |
$count |
optional | This parameter tells about the number of total replacements made on our original string or array. |
We will use the pattern /[^0-9]/
for finding numbers in the string
. The program that extracts numbers from the string
is as follows:
<?php
$string = 'Sarah has 4 dolls and 6 bunnies.';
$outputString = preg_replace('/[^0-9]/', '', $string);
echo("The extracted numbers are: $outputString \n");
?>
Output:
The extracted numbers are: 46