How to Check if a String Starts With a Specified String in PHP
-
Use
substr()
Function to Check if a String Starts With a Specified String in PHP -
Use
strpos()
Function to Check if a String Starts With a Specified String in PHP -
Use
strncmp()
Function to Check if a String Starts With a Specified String in PHP
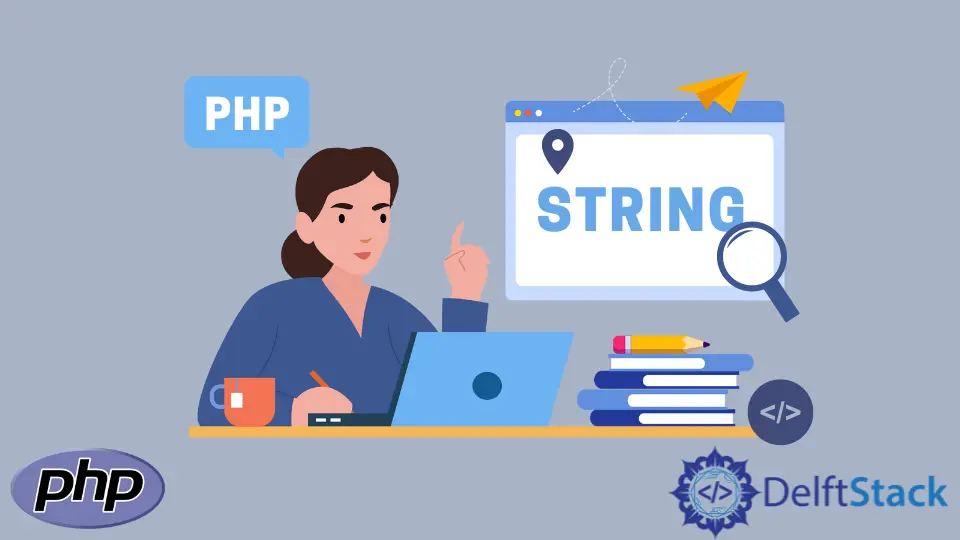
In this article, we will introduce methods to check if a string
starts with a specified string
in PHP.
- Using
substr()
function - Using
strpos()
function - Using
strncmp()
function
Use substr()
Function to Check if a String Starts With a Specified String in PHP
The built-in function substr()
is used to access a substring. The string
is passed as an input, and the substring that we want to access is returned. We can use this function to check if a string
starts with a specific string. The correct syntax to use this function is as follows
substr($string, $startPosition, $lengthOfSubstring);
This function has three parameters. The details of its parameters are as follows.
Parameters | Description | |
---|---|---|
$string |
mandatory | The original string whose substring we wish to access. |
$startPosition |
mandatory | An integer variable. It tells the position where our substring will start. If it is positive, then our substring starts from the left side of the string i.e from the beginning. If it is negative, then our substring starts from the end. |
$lengthOfSubstring |
optional | An integer variable. It specifies the total length of the string from the start position. If it is omitted, then the substring from the start position to the end of the string is returned. If it is negative, then the string from the end is removed according to its value. If it is zero, then an empty string is returned. |
<?php
$string = "Mr. Peter";
if(substr($string, 0, 3) === "Mr."){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
In the above code, we want to check if our string
starts with Mr.
.
substr($string, 0, 3)
0
is the start index of the substring, or in other words, the substring starts from the first character of the given string.
3
means the length of the returned substring is 3.
If the start of the string
is the same as Mr.
then it will display The string starts with the desired substring.
.
Output:
The string starts with the desired substring.
Use strpos()
Function to Check if a String Starts With a Specified String in PHP
The function strpos()
returns the position of the first occurrence of a substring in the given string
. We could use it to check if a string
starts with a specified string.
If the returned value is 0
, it means the given string starts with the specified substring. Otherwise, the string doesn’t start with the checked substring.
strpos()
is a case sensitive function. The correct syntax to use this function is as follows.
strpos($string, $searchString, $startPosition);
It has three parameters. The details of its parameters are as follows.
Parameter | Description | |
---|---|---|
$string |
mandatory | It is the string whose substring we wish to find. |
$searchString |
mandatory | It is the substring that will be searched in a string. |
$startPosition |
optional | It is the position in the string from where the search will start. |
<?php
$string = "Mr. Peter";
if(strpos( $string, "Mr." ) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
Here, we have checked if our string
starts with Mr.
by finding the first occurrence of Mr.
.
Output:
The string starts with the desired substring.
Use strncmp()
Function to Check if a String Starts With a Specified String in PHP
The built-in function strncmp()
compares two given strings
. This function is also case sensitive. The correct syntax to use this function is as follows.
strncmp($string1, $string2, $length);
It has three parameters. The details of its parameters are as follows.
Parameters | Description | |
---|---|---|
$string1 |
mandatory | It is the first string to be compared. |
$string2 |
mandatory | It is the second string to be compared. |
$length |
mandatory | It is the length of the string to be compared. |
It returns zero if both the strings
are equal. This is a case sensitive function.
<?php
$string = "Mr. Peter";
if(strncmp($string, "Mr.", 3) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
Here, the two strings are compared. The length of the strings to be compared is three.
Output:
The string starts with the desired substring.
The case insensitive version of strncmp
is strncasecmp
. It compares the first n characters of the given two strings regardless of their cases.
<?php
$string = "mr. Peter";
if(strncasecmp($string, "Mr.", 3) === 0){
echo "The string starts with the desired substring.";
}else
echo "The string does not start with the desired substring.";
?>
Output:
The string starts with the desired substring.