Different Ways to Compare Dates in PHP
-
Compare Dates With
strtotime()
andtime()
-
Compare Dates With
Date()
Function and Conditional Check - Compare Dates With a Custom Function
-
Compare Dates With PHP
Datetime
Object -
Multiple Date Comparisons With
Arsort()
andstrtotime()
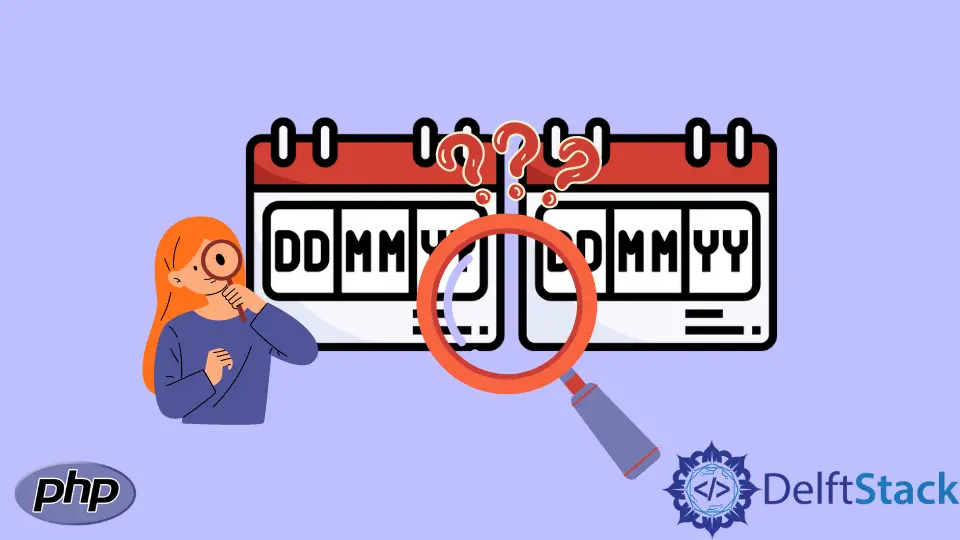
This article will teach you how to compare dates in PHP using 5 different techniques. Of these techniques, 4 will use built-in PHP functions like strtotime()
, time()
and date()
in one form or the other. The last technique will use PHP DateTime
object.
Compare Dates With strtotime()
and time()
The strtotime()
is a built-in time function in PHP. Its main use is to convert a string of human-readable dates into an equal UNIX timestamp. It can parse varieties of strings and convert them to their appropriate timestamp. Examples of such strings are 2 weeks ago
and next week
.
PHP time()
function returns the current time. This time is the number of seconds since the UNIX epoch. If you need to convert these seconds to the current date, you’ll need PHP’s built-in date()
function.
When combining these 2 functions, you can compare dates using the following steps.
First, you supply a date string to strtotime()
. It will convert it to its UNIX timestamp.
Subtract this UNIX timestamp from the current time using the time()
function.
You can use the result of this calculation and a conditional check to compare dates.
<?php
$dateString = '2021-12-12 21:00:00';
// This will check if the time
// is in the last day
if ((time() - (60 * 60 * 24)) < strtotime($dateString)) {
echo "Date in the last 24hrs";
} else {
echo "Date is <b>NOT</b> in the last 24hrs";
}
Output:
Date is <b>NOT</b> in the last 24hrs
Compare Dates With Date()
Function and Conditional Check
PHP’s date()
function allows you to format a timestamp into your desired format. The timestamp in question the seconds between the current time and the UNIX epoch. The UNIX epoch is the time since midnight of January 1st, 1970.
The value of the date() function depends on the time zone set in your php.ini
file.
If you want to compare a given date using the date()
function, do the following.
- Convert the current timestamp into your desired format
- Write the date you’d like to compare in an appropriate string
- Perform a conditional check between the date string and the current timestamp
<?php
$todaysDate = date("Y-m-d H:i:s");
$dateString = "2021-10-12 10:00:00";
if ($dateString < $todaysDate) {
echo "The date you supplied is LESS than the current date";
} else {
echo "The date you supplied is NOT LESS than the current date";
}
Output:
The date you supplied is LESS than the current date
Compare Dates With a Custom Function
You use a function to avoid code repetition. The first example in this tutorial showed how you can compare dates with strtotime()
and time()
.
In this case, you’ll create a function. This function will accept a date string as a parameter, which in turn, it will pass to strtotime()
. You pass the string "today"
to another strtotime()
inside the function.
Now, you have two things you can compare, which are,
- The time you want to compare
- The string
today
passed to anotherstrtotime()
You can use a strict comparison to compare the dates with these criteria. You can create a comparison function to check for the following.
- Today’s date
- A past date
- A future date
The following will check if the supplied date string is a current date:
<?php
function checkToday($time)
{
$convertToUNIXtime = strtotime($time);
$todayUNIXtime = strtotime('today');
return $convertToUNIXtime === $todayUNIXtime;
}
if (checkToday('2021-12-13')) {
echo "Yeah it's today";
} else {
echo "No, it's not today";
}
Output:
No, it's not today
Check for a past date.
<?php
function checkThePast($time)
{
$convertToUNIXtime = strtotime($time);
return $convertToUNIXtime < time();
}
if (checkThePast('2021-12-13 22:00:00')) {
echo "The date is in the past";
} else {
echo "No, it's not in the past";
}
Output:
The date is in the past
You can check a future date with the following.
<?php
function checkTheFuture($time)
{
$convertToUNIXtime = strtotime($time);
return $convertToUNIXtime > time();
}
if (checkTheFuture('2021-12-13 22:00:00')) {
echo "The date is in the future";
} else {
echo "No, it's not in the future";
}
Output:
No, it's not in the future
Compare Dates With PHP Datetime
Object
PHP DateTime
class gives you an object-oriented way to work with date strings in PHP. It has a suite of methods you can work with. It encapsulates some logic behind the scene and gives you a clean interface to work with.
Compared to strtotime()
and date()
, DateTime
has the following advantages:
- It can work with more date strings
- It is easier to work with
- It provides a direct comparison of dates
When you intend to compare dates with DateTime
, do the following.
- Parse the date string into a
DateTime
object - Compare the dates with operators like less than ( < ), greater than ( > ), or equal to ( == )
<?php
$firstDate = new DateTime("2020-12-13");
$secondDate = new DateTime("2021-12-13");
// Compare the date using operators
if ($firstDate > $secondDate) {
echo "First date is GREATER than the second date";
} elseif ($firstDate < $secondDate) {
echo "First date is LESS than the second date";
} else {
echo "First and second dates are EQUAL";
}
Output:
First date is LESS than the second date
Multiple Date Comparisons With Arsort()
and strtotime()
If you want to compare many date strings, you can store them in an array.
With the dates in the array, you can use a suitable loop method to work with the array. For example, foreach
.
In the loop within foreach
, you can convert each date to their UNIX timestamp using strtotime()
. Afterward, you can sort the dates with arsort()
.
<?php
// Store the dates
$dateArray = ["2020-09-30", "2021-12-13", "2021-08-05"];
// Convert each date to a UNIX timestamp
// and store them in another array
$dateArrayTimestamp = [];
foreach ($dateArray as $date) {
$dateArrayTimestamp[] = strtotime($date);
}
// Sort the dates,
arsort($dateArrayTimestamp);
// Print the date starting with the
// current date
foreach ($dateArrayTimestamp as $key => $value) {
echo "$key - " . date("Y - m - d", $value) . "<br>";
}
Output:
1 - 2021 - 12 - 13
2 - 2021 - 08 - 05
0 - 2020 - 09 - 30
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn