numpy.where() Multiple Conditions
-
Implement
numpy.where()
Multiple Conditions With the&
Operator in Python -
Implement
numpy.where()
Multiple Conditions With the|
Operator in Python -
Implement
numpy.where()
Multiple Conditions With thenumpy.logical_and()
Function -
Implement
numpy.where()
Multiple Conditions With thenumpy.logical_or()
Function in Python
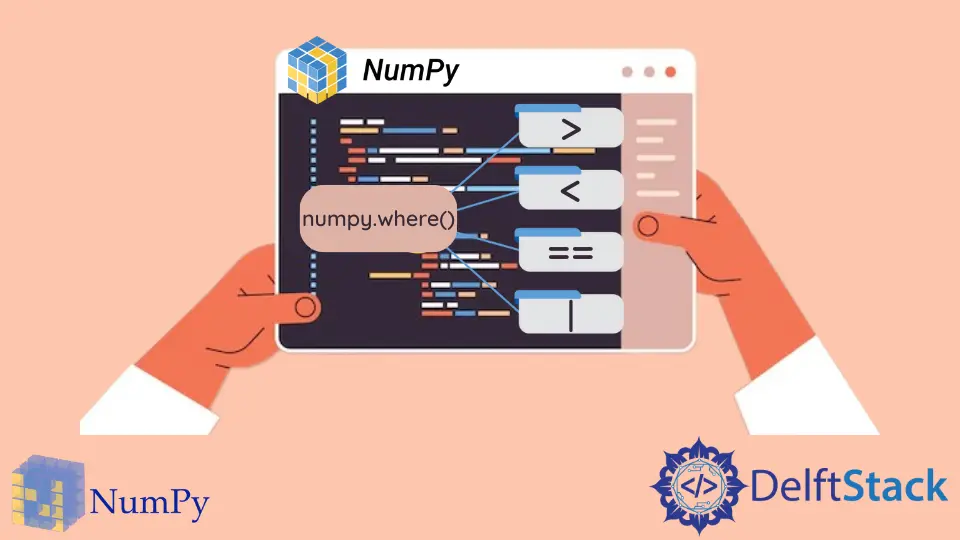
This tutorial will introduce the methods to specify multiple conditions in the numpy.where()
function in Python.
Implement numpy.where()
Multiple Conditions With the &
Operator in Python
The numpy.where()
function is used to select some elements from an array after applying a specified condition. Suppose we have a scenario where we have to specify multiple conditions inside a single numpy.where()
function. We can use the &
operator for this purpose. We can specify multiple conditions inside the numpy.where()
function by enclosing each condition inside a pair of parenthesis and using a &
operator between them.
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where((values > 2) & (values < 4))]
print(result)
Output:
[3]
In the above code, we selected the values from the array of integers values
greater than 2
but less than 4
with the np.where()
function along with the &
operator. We first created an array of integers values
with the np.array()
function. We then applied multiple conditions on the array elements with the np.where()
function and &
operator and stored the selected value inside the result
variable. This section discusses the use of the logical AND operator inside the np.where()
function. The following section discusses the use of the logical OR operator inside the np.where()
function.
Implement numpy.where()
Multiple Conditions With the |
Operator in Python
We can also use the |
operator to specify multiple conditions inside the numpy.where()
function. The |
operator represents a logical OR gate in Python. We can specify multiple conditions inside the numpy.where()
function by enclosing each condition inside a pair of parenthesis and using a |
operator between them.
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where((values > 2) | (values % 2 == 0))]
print(result)
Output:
[2 3 4 5]
In the above code, we selected the values from the array of integers values
that are either greater than 2
or completely divisible by 2
with the np.where()
function along with the |
operator. We first created an array of integers values
with the np.array()
function. We then applied multiple conditions on the array elements with the np.where()
function and |
operator and stored the selected values inside the result
variable.
Implement numpy.where()
Multiple Conditions With the numpy.logical_and()
Function
The numpy.logical_and()
function is used to calculate the element-wise truth value of AND gate in Python. We can use the numpy.logical_and()
function inside the numpy.where()
function to specify multiple conditions.
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where(np.logical_and(values > 2, values < 4))]
print(result)
Output:
[3]
In the above code, we selected the values from the array of integers values
greater than 2
but less than 4
with the np.where()
function along with the np.logical_and()
function in Python. We first created an array of integers values
with the np.array()
function. We then applied multiple conditions on the array elements with the np.where()
function and np.logical_and()
function, and stored the selected value inside the result
variable.
Implement numpy.where()
Multiple Conditions With the numpy.logical_or()
Function in Python
The numpy.logical_or()
function is used to calculate the element-wise truth value of OR gate in Python. We can use the numpy.logical_or()
function inside the numpy.where()
function to specify multiple conditions.
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where(np.logical_or(values > 2, values % 2 == 0))]
print(result)
Output:
[2 3 4 5]
In the above code, we selected the values from the array of integers values
that are either greater than 2
or completely divisible by 2
with the np.where()
function along with the numpy.logical_or()
function in Python. We first created an array of integers values
with the np.array()
function. We then applied multiple conditions on the array elements with the np.where()
function and the numpy.logical_or()
function and stored the selected values inside the result
variable.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn