numpy.where() 多个条件
-
在 Python 中使用
&
运算符实现numpy.where()
多个条件 -
使用
|
实现numpy.where()
多个条件 Python 中的运算符 -
使用
numpy.logical_and()
函数实现numpy.where()
多个条件 -
在 Python 中使用
numpy.logical_or()
函数实现numpy.where()
多个条件
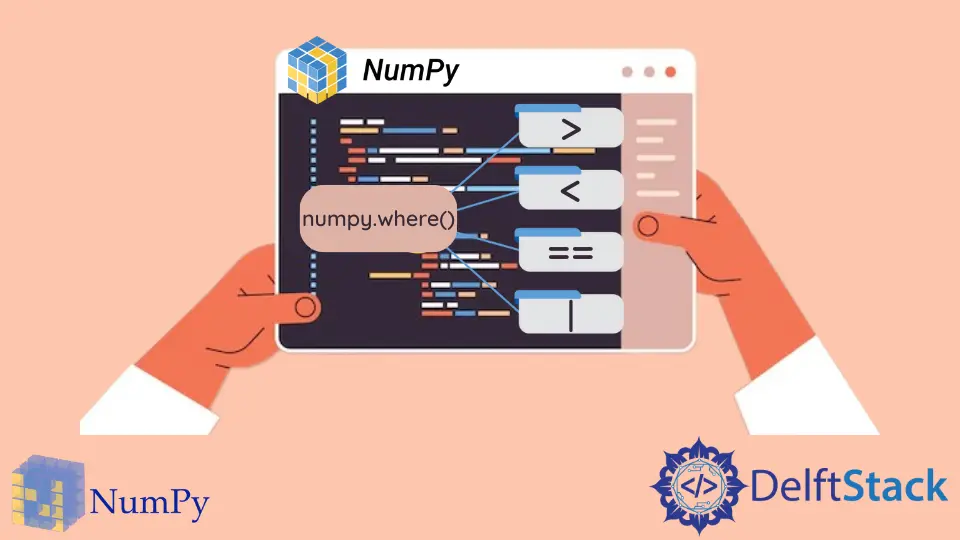
本教程将介绍在 Python 中的 numpy.where()
函数中指定多个条件的方法。
在 Python 中使用 &
运算符实现 numpy.where()
多个条件
numpy.where()
函数用于在应用指定条件后从数组中选择一些元素。假设我们有一个场景,我们必须在单个 numpy.where()
函数中指定多个条件。为此,我们可以使用 &
运算符。我们可以在 numpy.where()
函数中指定多个条件,方法是将每个条件括在一对括号内并在它们之间使用 &
运算符。
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where((values > 2) & (values < 4))]
print(result)
输出:
[3]
在上面的代码中,我们使用 np.where()
函数和&
运算符从大于 2
但小于 4
的整数 values
数组中选择值。我们首先使用 np.array()
函数创建了一个整数 values
数组。然后,我们使用 np.where()
函数和 &
运算符对数组元素应用多个条件,并将选定的值存储在 result
变量中。本节讨论在 np.where()
函数中使用逻辑 AND 运算符。以下部分讨论了 np.where()
函数中逻辑 OR 运算符的使用。
使用 |
实现 numpy.where()
多个条件 Python 中的运算符
我们也可以使用|
运算符在 numpy.where()
函数内指定多个条件。|
运算符代表 Python 中的逻辑 OR 门。我们可以在 numpy.where()
函数中指定多个条件,方法是将每个条件括在一对括号内并使用 |
他们之间的运营商。
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where((values > 2) | (values % 2 == 0))]
print(result)
输出:
[2 3 4 5]
在上面的代码中,我们使用 np.where()
函数和 |
从整数 values
数组中选择大于 2
或可被 2
整除的值运算符。我们首先使用 np.array()
函数创建了一个整数 values
数组。然后我们使用 np.where()
函数和 |
对数组元素应用多个条件运算符并将选定的值存储在 result
变量中。
使用 numpy.logical_and()
函数实现 numpy.where()
多个条件
numpy.logical_and()
函数用于计算 Python 中 AND 门的逐元素真值。我们可以在 numpy.where()
函数中使用 numpy.logical_and()
函数来指定多个条件。
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where(np.logical_and(values > 2, values < 4))]
print(result)
输出:
[3]
在上面的代码中,我们使用 np.where()
函数和 np.logical_and()
函数从大于 2
但小于 4
的整数数组 values
中选择值 Python。我们首先使用 np.array()
函数创建了一个整数 values
数组。然后我们使用 np.where()
函数和 np.logical_and()
函数对数组元素应用多个条件,并将选定的值存储在 result
变量中。
在 Python 中使用 numpy.logical_or()
函数实现 numpy.where()
多个条件
numpy.logical_or()
函数用于计算 Python 中 OR 门的元素真值。我们可以在 numpy.where()
函数中使用 numpy.logical_or()
函数来指定多个条件。
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where(np.logical_or(values > 2, values % 2 == 0))]
print(result)
输出:
[2 3 4 5]
在上面的代码中,我们使用 np.where()
和 numpy.logical_or()
函数从整数 values
数组中选择大于 2
或完全可以被 2
整除的值。我们首先使用 np.array()
函数创建了一个整数 values
数组。然后,我们使用 np.where()
函数和 numpy.logical_or()
函数对数组元素应用多个条件,并将选定的值存储在 result
变量中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn