numpy.where() 多個條件
-
在 Python 中使用
&
運算子實現numpy.where()
多個條件 -
使用
|
實現numpy.where()
多個條件 Python 中的運算子 -
使用
numpy.logical_and()
函式實現numpy.where()
多個條件 -
在 Python 中使用
numpy.logical_or()
函式實現numpy.where()
多個條件
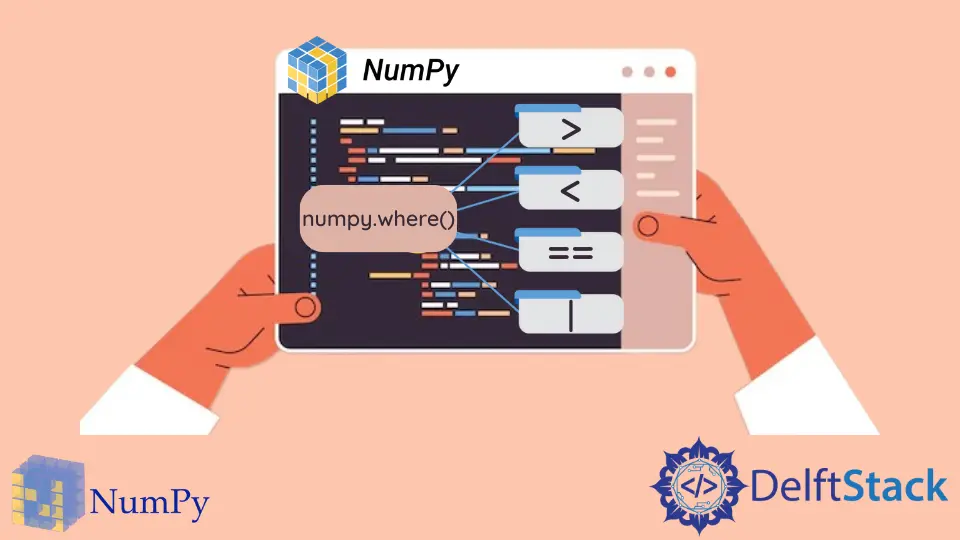
本教程將介紹在 Python 中的 numpy.where()
函式中指定多個條件的方法。
在 Python 中使用 &
運算子實現 numpy.where()
多個條件
numpy.where()
函式用於在應用指定條件後從陣列中選擇一些元素。假設我們有一個場景,我們必須在單個 numpy.where()
函式中指定多個條件。為此,我們可以使用 &
運算子。我們可以在 numpy.where()
函式中指定多個條件,方法是將每個條件括在一對括號內並在它們之間使用 &
運算子。
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where((values > 2) & (values < 4))]
print(result)
輸出:
[3]
在上面的程式碼中,我們使用 np.where()
函式和&
運算子從大於 2
但小於 4
的整數 values
陣列中選擇值。我們首先使用 np.array()
函式建立了一個整數 values
陣列。然後,我們使用 np.where()
函式和 &
運算子對陣列元素應用多個條件,並將選定的值儲存在 result
變數中。本節討論在 np.where()
函式中使用邏輯 AND 運算子。以下部分討論了 np.where()
函式中邏輯 OR 運算子的使用。
使用 |
實現 numpy.where()
多個條件 Python 中的運算子
我們也可以使用|
運算子在 numpy.where()
函式內指定多個條件。|
運算子代表 Python 中的邏輯 OR 門。我們可以在 numpy.where()
函式中指定多個條件,方法是將每個條件括在一對括號內並使用 |
他們之間的運營商。
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where((values > 2) | (values % 2 == 0))]
print(result)
輸出:
[2 3 4 5]
在上面的程式碼中,我們使用 np.where()
函式和 |
從整數 values
陣列中選擇大於 2
或可被 2
整除的值運算子。我們首先使用 np.array()
函式建立了一個整數 values
陣列。然後我們使用 np.where()
函式和 |
對陣列元素應用多個條件運算子並將選定的值儲存在 result
變數中。
使用 numpy.logical_and()
函式實現 numpy.where()
多個條件
numpy.logical_and()
函式用於計算 Python 中 AND 門的逐元素真值。我們可以在 numpy.where()
函式中使用 numpy.logical_and()
函式來指定多個條件。
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where(np.logical_and(values > 2, values < 4))]
print(result)
輸出:
[3]
在上面的程式碼中,我們使用 np.where()
函式和 np.logical_and()
函式從大於 2
但小於 4
的整數陣列 values
中選擇值 Python。我們首先使用 np.array()
函式建立了一個整數 values
陣列。然後我們使用 np.where()
函式和 np.logical_and()
函式對陣列元素應用多個條件,並將選定的值儲存在 result
變數中。
在 Python 中使用 numpy.logical_or()
函式實現 numpy.where()
多個條件
numpy.logical_or()
函式用於計算 Python 中 OR 門的元素真值。我們可以在 numpy.where()
函式中使用 numpy.logical_or()
函式來指定多個條件。
import numpy as np
values = np.array([1, 2, 3, 4, 5])
result = values[np.where(np.logical_or(values > 2, values % 2 == 0))]
print(result)
輸出:
[2 3 4 5]
在上面的程式碼中,我們使用 np.where()
和 numpy.logical_or()
函式從整數 values
陣列中選擇大於 2
或完全可以被 2
整除的值。我們首先使用 np.array()
函式建立了一個整數 values
陣列。然後,我們使用 np.where()
函式和 numpy.logical_or()
函式對陣列元素應用多個條件,並將選定的值儲存在 result
變數中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn