How to Convert Tensor to NumPy Array in Python
-
Convert a Tensor to a NumPy Array With the
Tensor.numpy()
Function in Python -
Convert a Tensor to a NumPy Array With the
Tensor.eval()
Function in Python -
Convert a Tensor to a NumPy Array With the
TensorFlow.Session()
Function in Python
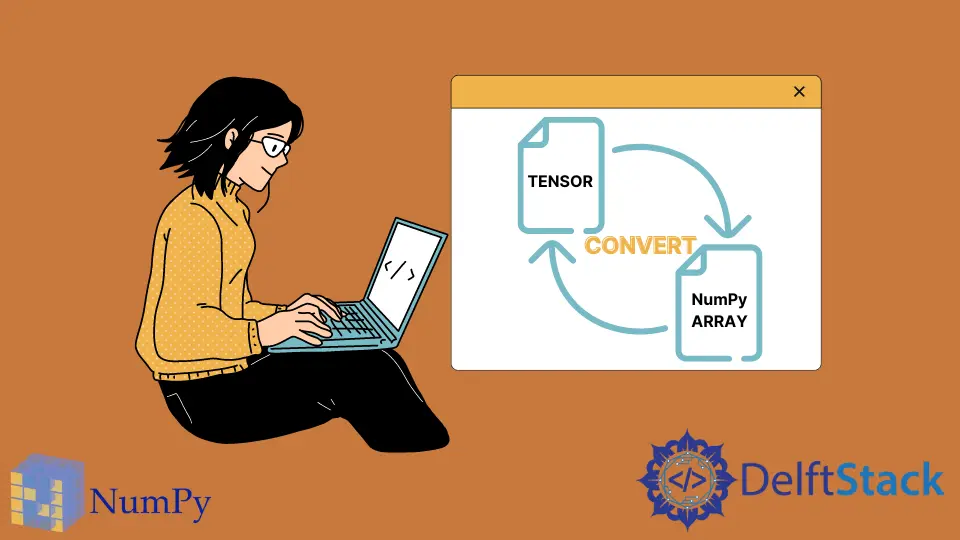
This tutorial will introduce the methods to convert a Tensor to a NumPy array in Python.
Convert a Tensor to a NumPy Array With the Tensor.numpy()
Function in Python
The Eager Execution
of the TensorFlow library can be used to convert a tensor to a NumPy array in Python. With Eager Execution
, the behavior of the operations of TensorFlow library changes, and the operations execute immediately. We can also perform NumPy operations on Tensor objects with Eager Execution
. The Tensor.numpy()
function converts the Tensor to a NumPy array in Python. In TensorFlow 2.0, the Eager Execution
is enabled by default. So, this approach works best for the TensorFlow version 2.0. See the following code example.
import tensorflow as tf
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tensor.numpy()
print("Array = ", array)
Output:
Tensor = tf.Tensor(
[[1 2 3]
[4 5 6]
[7 8 9]], shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
In the above code, we first created and initialized the Tensor object tensor
with the tf.constant()
function in Python. We printed the tensor
and converted it to a NumPy array array
with the tensor.numpy()
function in Python. In the end, we printed the array
.
Convert a Tensor to a NumPy Array With the Tensor.eval()
Function in Python
We can also use the Tensor.eval()
function to convert a Tensor to a NumPy array in Python. This method is not supported in the TensorFlow version 2.0. So, we have to either keep the previous version 1.0 of the TensorFlow or disable all the behavior of version 2.0 of the TensorFlow library. See the following code example.
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tensor.eval(session=tf.Session())
print("Array = ", array)
Output:
Tensor = Tensor("Const_1:0", shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
In the above code, we converted the Tensor object tensor
to the NumPy array array
with the tensor.eval()
function in Python. We first imported version 1.0 of the TensorFlow library and disabled all the behavior of version 2.0. We then created and initialized the tensor
with the tf.constant()
function and printed the values in tensor
. We then executed the tensor.eval()
function and saved the returned value inside the array
, and printed the values in array
.
Convert a Tensor to a NumPy Array With the TensorFlow.Session()
Function in Python
The TensorFlow.Session()
is another method that can be used to convert a Tensor to a NumPy array in Python. This method is very similar to the previous approach with the Tensor.eval()
function. This approach is also not supported by version 2.0 of the TensorFlow library. We either have to install version 1.0 of the TensorFlow library or disable all the behavior of version 2.0 of the TensorFlow library. We can pass our Tensor object to the TensorFlow.Session().run()
function to convert that Tensor object to a NumPy array in Python. See the following code example.
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tf.Session().run(tensor)
print("Array = ", array)
Output:
Tensor = Tensor("Const_6:0", shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
In the above code, we converted the Tensor object tensor
to the NumPy array array
with the tf.Session.run(tensor)
function in Python. We first imported the version 1.0 compatible TensorFlow library and disabled all the behavior of version 2.0. We then created the Tensor object tensor
and printed the values of tensor
. We then converted the tensor
Tensor to the array
NumPy array with the tf.Session.run(tensor)
function and printed the values in array
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn