在 Python 中將 Tensor 轉換為 NumPy 陣列
-
在 Python 中使用
Tensor.numpy()
函式將 tensor 轉換為 NumPy 陣列 -
在 Python 中使用
Tensor.eval()
函式將 tensor 轉換為 NumPy 陣列 -
在 Python 中使用
TensorFlow.Session()
函式將 tensor 轉換為 NumPy 陣列
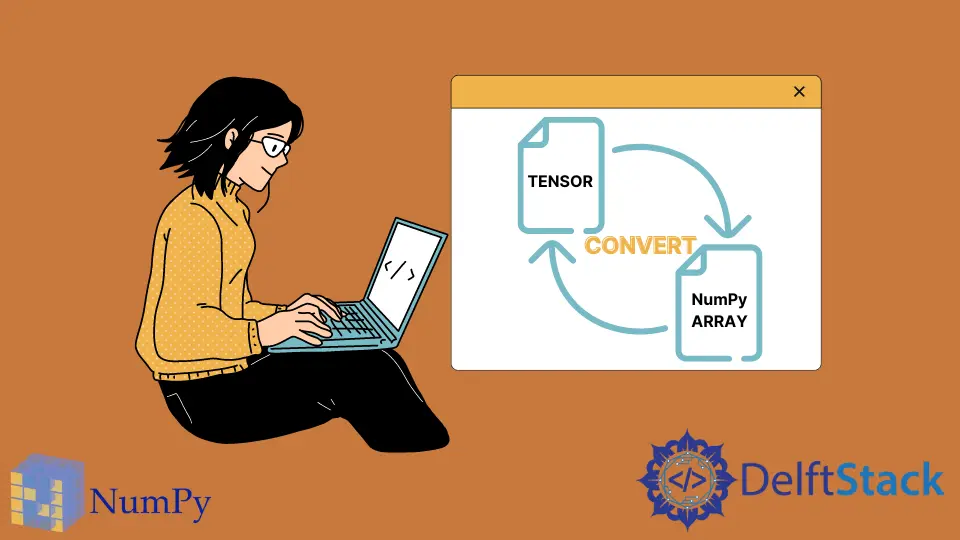
本教程將介紹在 Python 中將 Tensor 轉換為 NumPy 陣列的方法。
在 Python 中使用 Tensor.numpy()
函式將 tensor 轉換為 NumPy 陣列
TensorFlow 庫的 Eager Execution
可用於在 Python 中將 tensor 轉換為 NumPy 陣列。使用 Eager Execution
,TensorFlow 庫操作的行為會更改,並且這些操作會立即執行。我們還可以使用 Eager Execution
在 Tensor 物件上執行 NumPy 操作。Tensor.numpy()
函式將 Tensor 轉換為 Python 中的 NumPy 陣列。在 TensorFlow 2.0 中,預設情況下啟用了 Eager Execution
。因此,此方法最適合 TensorFlow 2.0 版。請參見以下程式碼示例。
import tensorflow as tf
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tensor.numpy()
print("Array = ", array)
輸出:
Tensor = tf.Tensor(
[[1 2 3]
[4 5 6]
[7 8 9]], shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
在上面的程式碼中,我們首先在 Python 中使用 tf.constant()
函式建立並初始化了 Tensor 物件 tensor
。我們在 Python 中使用 tensor.numpy()函式列印了 tensor
,並將其轉換為 NumPy 陣列 array
。最後,我們列印了陣列
。
在 Python 中使用 Tensor.eval()
函式將 tensor 轉換為 NumPy 陣列
我們還可以使用 Tensor.eval()
函式在 Python 中將 Tensor 轉換為 NumPy 陣列。TensorFlow 2.0 版不支援此方法。因此,我們必須保留 TensorFlow 的先前版本 1.0 或禁用 TensorFlow 庫的 2.0 版的所有行為。請參見以下程式碼示例。
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tensor.eval(session=tf.Session())
print("Array = ", array)
輸出:
Tensor = Tensor("Const_1:0", shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
在上面的程式碼中,我們使用 Python 中的 tensor.eval()
函式將 Tensor 物件 tensor
轉換為 NumPy 陣列 array
。我們首先匯入了 TensorFlow 庫的 1.0 版並禁用了 2.0 版的所有行為。然後,我們使用 tf.constant()
函式建立並初始化 tensor
,並在 tensor
中列印值。然後,我們執行 tensor.eval()
函式,並將返回的值儲存在 array
內,並將值列印在 array
中。
在 Python 中使用 TensorFlow.Session()
函式將 tensor 轉換為 NumPy 陣列
TensorFlow.Session()
是另一個可用於在 Python 中將 Tensor 轉換為 NumPy 陣列的方法。該方法與以前的帶有 Tensor.eval()
函式的方法非常相似。TensorFlow 庫的 2.0 版也不支援此方法。我們要麼必須安裝 TensorFlow 庫的 1.0 版本,要麼禁用 TensorFlow 庫的 2.0 版的所有行為。我們可以將 Tensor 物件傳遞給 TensorFlow.Session().run()
函式,以將該 Tensor 物件轉換為 Python 中的 NumPy 陣列。請參見以下程式碼示例。
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tf.Session().run(tensor)
print("Array = ", array)
輸出:
Tensor = Tensor("Const_6:0", shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
在上面的程式碼中,我們使用 Python 中的 tf.Session.run(tensor)
函式將 Tensor 物件 tensor
轉換為 NumPy 陣列 array
。我們首先匯入了 1.0 版相容的 TensorFlow 庫並禁用了 2.0 版的所有行為。然後,我們建立了 tensor 物件 tensor
,並列印了 tensor
的值。然後,使用 tf.Session.run(tensor)
函式將 tensor
tensor 轉換為 array
NumPy 陣列,並將值列印在 array
中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn