在 Python 中将 Tensor 转换为 NumPy 数组
-
在 Python 中使用
Tensor.numpy()
函数将 tensor 转换为 NumPy 数组 -
在 Python 中使用
Tensor.eval()
函数将 tensor 转换为 NumPy 数组 -
在 Python 中使用
TensorFlow.Session()
函数将 tensor 转换为 NumPy 数组
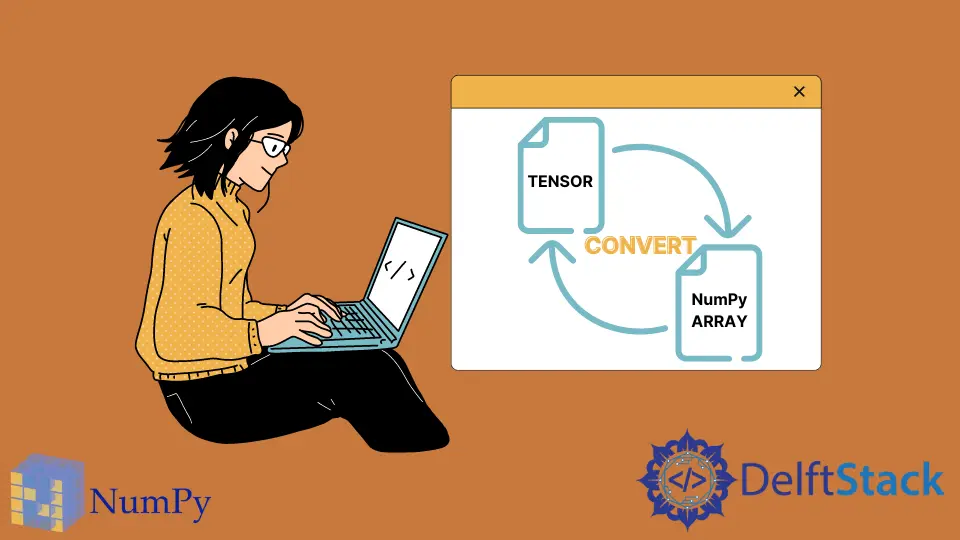
本教程将介绍在 Python 中将 Tensor 转换为 NumPy 数组的方法。
在 Python 中使用 Tensor.numpy()
函数将 tensor 转换为 NumPy 数组
TensorFlow 库的 Eager Execution
可用于在 Python 中将 tensor 转换为 NumPy 数组。使用 Eager Execution
,TensorFlow 库操作的行为会更改,并且这些操作会立即执行。我们还可以使用 Eager Execution
在 Tensor 对象上执行 NumPy 操作。Tensor.numpy()
函数将 Tensor 转换为 Python 中的 NumPy 数组。在 TensorFlow 2.0 中,默认情况下启用了 Eager Execution
。因此,此方法最适合 TensorFlow 2.0 版。请参见以下代码示例。
import tensorflow as tf
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tensor.numpy()
print("Array = ", array)
输出:
Tensor = tf.Tensor(
[[1 2 3]
[4 5 6]
[7 8 9]], shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
在上面的代码中,我们首先在 Python 中使用 tf.constant()
函数创建并初始化了 Tensor 对象 tensor
。我们在 Python 中使用 tensor.numpy()函数打印了 tensor
,并将其转换为 NumPy 数组 array
。最后,我们打印了数组
。
在 Python 中使用 Tensor.eval()
函数将 tensor 转换为 NumPy 数组
我们还可以使用 Tensor.eval()
函数在 Python 中将 Tensor 转换为 NumPy 数组。TensorFlow 2.0 版不支持此方法。因此,我们必须保留 TensorFlow 的先前版本 1.0 或禁用 TensorFlow 库的 2.0 版的所有行为。请参见以下代码示例。
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tensor.eval(session=tf.Session())
print("Array = ", array)
输出:
Tensor = Tensor("Const_1:0", shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
在上面的代码中,我们使用 Python 中的 tensor.eval()
函数将 Tensor 对象 tensor
转换为 NumPy 数组 array
。我们首先导入了 TensorFlow 库的 1.0 版并禁用了 2.0 版的所有行为。然后,我们使用 tf.constant()
函数创建并初始化 tensor
,并在 tensor
中打印值。然后,我们执行 tensor.eval()
函数,并将返回的值保存在 array
内,并将值打印在 array
中。
在 Python 中使用 TensorFlow.Session()
函数将 tensor 转换为 NumPy 数组
TensorFlow.Session()
是另一个可用于在 Python 中将 Tensor 转换为 NumPy 数组的方法。该方法与以前的带有 Tensor.eval()
函数的方法非常相似。TensorFlow 库的 2.0 版也不支持此方法。我们要么必须安装 TensorFlow 库的 1.0 版本,要么禁用 TensorFlow 库的 2.0 版的所有行为。我们可以将 Tensor 对象传递给 TensorFlow.Session().run()
函数,以将该 Tensor 对象转换为 Python 中的 NumPy 数组。请参见以下代码示例。
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
tensor = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print("Tensor = ", tensor)
array = tf.Session().run(tensor)
print("Array = ", array)
输出:
Tensor = Tensor("Const_6:0", shape=(3, 3), dtype=int32)
Array = [[1 2 3]
[4 5 6]
[7 8 9]]
在上面的代码中,我们使用 Python 中的 tf.Session.run(tensor)
函数将 Tensor 对象 tensor
转换为 NumPy 数组 array
。我们首先导入了 1.0 版兼容的 TensorFlow 库并禁用了 2.0 版的所有行为。然后,我们创建了 tensor 对象 tensor
,并打印了 tensor
的值。然后,使用 tf.Session.run(tensor)
函数将 tensor
tensor 转换为 array
NumPy 数组,并将值打印在 array
中。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn