Element-Wise Multiplication in NumPy
-
Element-Wise Multiplication of Matrices in Python Using the
np.multiply()
Method -
Element-Wise Multiplication of Matrices in Python Using the
*
Operator
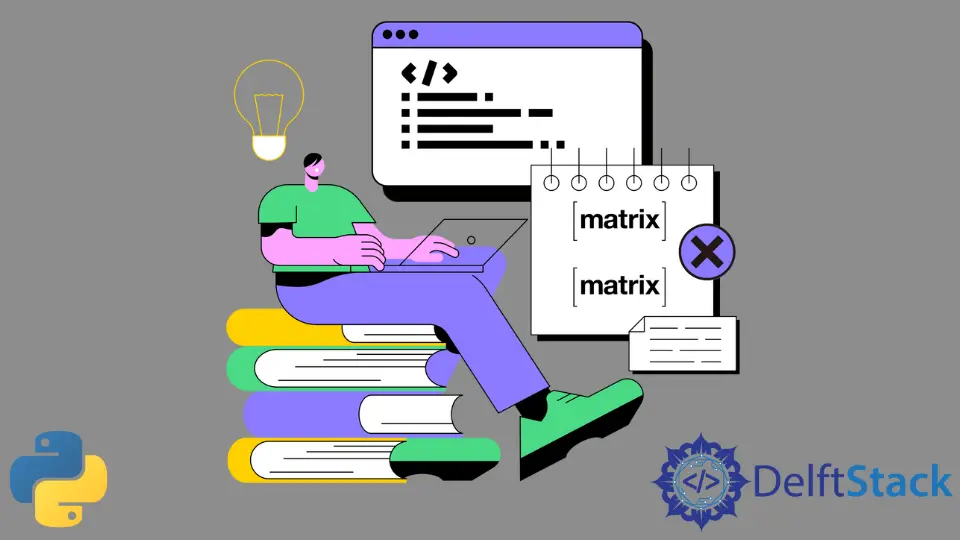
This tutorial will explain various methods to perform element-wise matrix multiplication in Python. In element-wise matrix multiplication (also known as Hadamard Product), every element of the first matrix is multiplied by the second matrix’s corresponding element.
When performing the element-wise matrix multiplication, both matrices should be of the same dimensions. The resultant matrix c
of the element-wise matrix multiplication a*b = c
always has the same dimension as that in a
and b
.
We can perform the element-wise multiplication in Python using the following methods:
Element-Wise Multiplication of Matrices in Python Using the np.multiply()
Method
The np.multiply(x1, x2)
method of the NumPy
library of Python takes two matrices x1
and x2
as input, performs element-wise multiplication on input, and returns the resultant matrix as input.
Therefore, we need to pass the two matrices as input to the np.multiply()
method to perform element-wise input. The below example code demonstrates how to use the np.multiply()
to perform element-wise multiplication of two matrices in Python.
import numpy as np
a1 = np.array([[12, 46, 23, 7, 2], [3, 5, 8, 3, 6]])
a2 = np.array([[15, 26, 2, 17, 22], [13, 8, 9, 3, 4]])
print(np.multiply(a1, a2))
Output:
[[ 180 1196 46 119 44]
[ 39 40 72 9 24]]
We can also perform the element-wise multiplication of specific rows, columns, or submatrices of the matrices using the np.multiply()
method. We need to pass the specific rows, columns, or submatrices of the matrices to the np.multiply()
method. Like in the element-wise matrix multiplication, the size of the rows, columns, or submatrices passed as first and second operand for multiplication should also be the same.
The example code below demonstrates how to implement element-wise multiplication of rows, columns, or submatrices of two matrices in Python.
import numpy as np
a1 = np.array([[12, 46, 23, 7, 2], [3, 5, 8, 3, 6]])
a2 = np.array([[15, 26, 2, 17, 22], [13, 8, 9, 3, 4]])
print(np.multiply(a1[0, :], a2[1, :]))
print(np.multiply(a1[1, :], a2[0, :]))
print(np.multiply(a1[:, 3], a2[:, 1]))
Output:
[156 368 207 21 8]
[ 45 130 16 51 132]
[182 24]
Element-Wise Multiplication of Matrices in Python Using the *
Operator
We can also use the *
operator with the matrices to perform element-wise matrix multiplication. The *
operator, when used with the matrices in Python, returns a resultant matrix of the element-wise matrix multiplication.
The below example code demonstrates how to use the *
operator to perform the element-wise matric multiplication in Python:
a1 = np.array([[12, 46, 23, 7, 2], [3, 5, 8, 3, 6]])
a2 = np.array([[15, 26, 2, 17, 22], [13, 8, 9, 3, 4]])
print(a1 * a2)
Output:
[[ 180 1196 46 119 44]
[ 39 40 72 9 24]]
We can also use the *
operator to perform the element-wise multiplication of rows, columns, and submatrices of the matrices in the following way in Python.
import numpy as np
a1 = np.array([[12, 46, 23, 7, 2], [3, 5, 8, 3, 6]])
a2 = np.array([[15, 26, 2, 17, 22], [13, 8, 9, 3, 4]])
print(a1[0, :] * a2[1, :])
print(a1[1, :] * a2[0, :])
print(a1[:, 3] * a2[:, 1])
Output:
[156 368 207 21 8]
[ 45 130 16 51 132]
[182 24]