How to Read File in Node.js
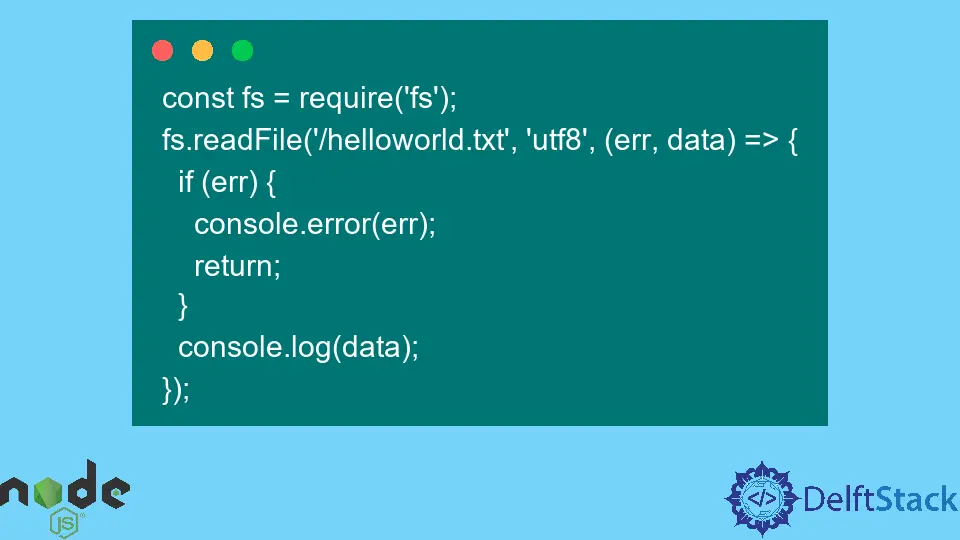
In this short article, we’ll learn how to read files in Node.js.
Read file in Node.js
The fs
module provides many useful functions for accessing and interacting with the file system. A special feature of the fs
module is that all methods are asynchronous by default but can also work synchronously by adding sync.
We will use fs.readFile()
to read a file in Node.js. You need to pass the file path, encoding, and a callback function to invoke the file data or error.
The fs.readFile()
method is a built-in method used to read the file. It reads the whole file into the buffer. require()
method is used to load the module like const fs = require('fs')
.
Syntax:
fs.readFile(filename, encoding, callbackFn)
This method accepts the three parameters.
filename
- This mandatory parameter holds the file’s name to read or the entire path if stored at another location.encoding
- This parameter is a mandatory parameter that holds the encoding of the file. Its default value isutf8
.callbackFn
- This callback function will be called after the file has been read. It takes two parameters,err
anddata
.
callbackFn Parameters |
Description |
---|---|
err |
If any error occurred while reading the file. |
data |
Contents of the file that is being read. |
It returns the contents, data stored in the file, or an error is encountered while reading the file. Let’s understand it with an example.
Code:
const fs = require('fs');
fs.readFile('/helloworld.txt', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
Output:
Hello DelftStack readers!
Another option is using the synchronous version fs.readFileSync()
.
Code:
const fs = require('fs');
try {
const fileContent = fs.readFileSync('/helloworld.txt', 'utf8');
console.log(fileContent);
} catch (err) {
console.error(err);
}
Output:
Hello DelftStack readers!
We can also use the fsPromises.readFile()
method offered by the fs/promises
module.
Code:
const fsPromises = require('fs/promises');
async function FileReadFn() {
try {
const fileContent =
await fsPromises.readFile('/helloworld.txt', {encoding: 'utf8'});
console.log(fileContent);
} catch (err) {
console.log(err);
}
}
FileReadFn();
Output:
Hello DelftStack readers!
All three of fs.readFile()
, fsPromises.readFile()
and fs.readFileSync()
reads the entire content of the file into memory before returning the data. This means that large files will greatly impact memory usage and program execution speed.
Instead of waiting for the file to finish reading, we start streaming to the HTTP client as soon as we have a piece of data ready to send. Streams essentially offer two main advantages over using other data processing methods.
Memory efficiency
- You don’t have to load large amounts of data into memory before processing it.Time efficiency
- It takes much less time to start processing the data since you can start processing immediately instead of waiting until the entire payload is available.
On the file stream, the pipe()
method is called, and it takes the source and directs it to a destination. The target stream is the return value of the pipe()
method, which is a very handy thing that allows us to chain multiple calls to pipe()
together.
Code:
const fs = require('fs');
const http = require('http');
const nodeServer = http.createServer((req, res) => {
const fileStream = fs.createReadStream(`${__dirname}/helloworld.txt`);
fileStream.pipe(res);
});
nodeServer.listen(3000);
Output:
Hello DelftStack readers!
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn