How to Download a File in Node JS Without Using Third Party Libraries
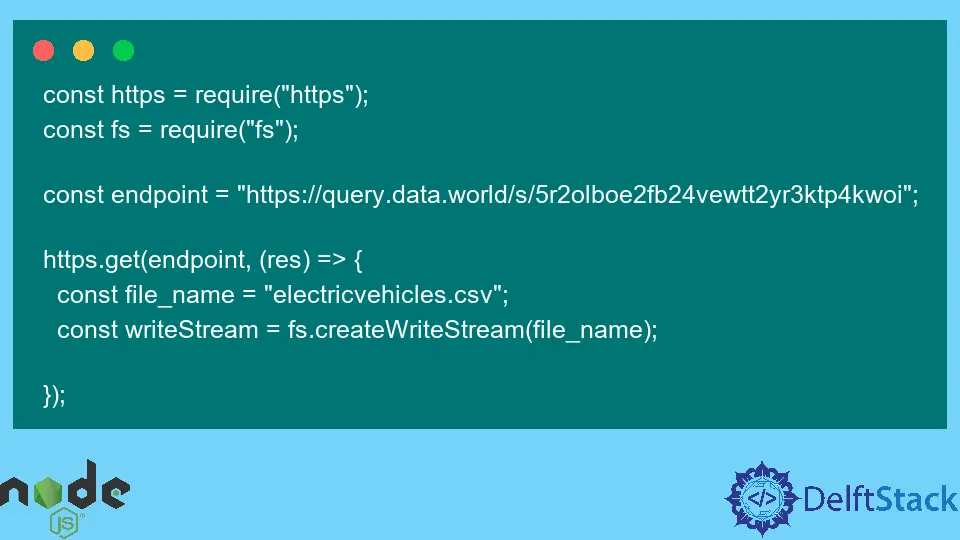
Node js makes heavy use of third-party libraries in most of its operations.
However, when performing operations such as downloading files, we can also use built-in packages or modules that are part of Node js core by requiring them in our programs.
Downloading a File in NodeJS
We can download any file in Node js using the fs
and HTTP
modules.
The fs
module provides us with the functionality to work with files on our system besides access to a range of asynchronous methods to perform other basic operations on files, such as renaming and deleting.
On the other hand, Nodejs provides the standard HTTP
and HTTPS
modules in its standard library. Using this method, we can request a server using the same module.
We can also create a server that listens to ports and responds. First, we will need to create a simple Node js application with the main file index.js
to get started.
Now under this file, using the require()
functions, we will require the fs
module and HTTP
module without any prior installations, as shown here.
const http = require('https');
const fs = require('fs');
Using the http.get()
method, we will then pass in the endpoint to the resource that we want to download as the first argument, followed by a callback
function whose work is to run once the file stream has been received and create the file in our local system using the name specified in the function.
Using streams
lets us download this file faster since we don’t have to wait until the whole data payload has been loaded. The streaming process begins once the data is available to respond to the HTTP
client.
Using the streams
powered Node js API fs.createWriteStream()
, we will create a writable stream to the file we want to make. This method accepts only one argument: the location where we want the file saved.
Using the fs
Module to Create a File in the Specified Location
const https = require('https');
const fs = require('fs');
const endpoint = 'https://query.data.world/s/5r2olboe2fb24vewtt2yr3ktp4kwoi';
https.get(endpoint, (res) => {
const file_name = 'electricvehicles.csv';
const writeStream = fs.createWriteStream(file_name);
});
Please note that since the fs
module will automatically save this current folder, we have instead specified the name we want the file we are downloading.
Finally, using the pipe()
method, usually called on the file stream, we will pipe the file stream to the HTTP
response and close the write stream using the writeStream.close()
method.
const https = require('https');
const fs = require('fs');
const endpoint = 'https://query.data.world/s/5r2olboe2fb24vewtt2yr3ktp4kwoi';
https.get(endpoint, (res) => {
const file_name = 'electricvehicles.csv';
const writeStream = fs.createWriteStream(file_name);
res.pipe(writeStream);
writeStream.on('end', function() {
writeStream.close();
console.log('Thed download is Completed');
});
});
Running the code above downloads the CSV
file in the current folder naming it after the name specified in the code as shown below.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn