How to Write Files in Node JS
-
Using the
fs.writeFile()
to Write Data in NodeJS -
Using the
fs.appendFile()
to Write Content to a File in NodeJS
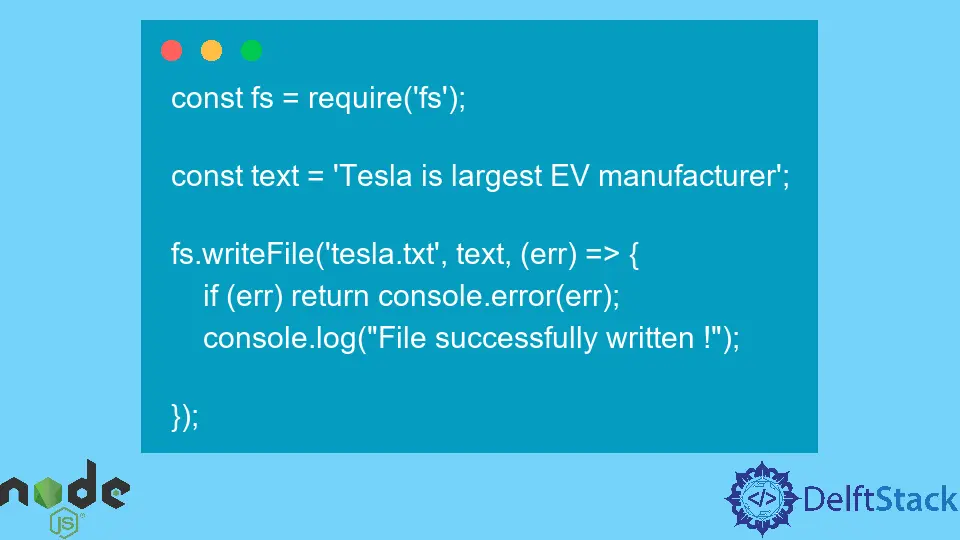
Working with files is an essential skill for every developer, regardless of the programming language, you’re using. In Node js, working with files is mainly through the fs module, which performs various operations on files.
Since the fs module is present in the standard library, we can use it in our programs by simply requiring it.
const fs = require('fs')
One of the asynchronous by default methods provided by the fs module is the fs.writeFile()
way to write data of any type to a file. This method accepts four parameters, as shown here.
Using the fs.writeFile()
to Write Data in NodeJS
var fs = require('fs');
fs.writeFile(filepath, data, [encoding], flag, [callback])
The first parameter is used to specify the location of the file we are writing to. The second parameter is the data we write to a file in the specified location.
The encoding parameters are optional.
However, there are various encoding formats such as base64
, ASCII
, and utf8
.The default encoding format is utf8
when this parameter is not specified.
The flag parameter is also an optional parameter that specifies the kind of writing operation we want to be performed on the target file.
The default flag is w
, which is stands for writing. Some of the other flags that we may use are r+
for reading and writing, w+
for positioning the stream at the beginning of a file, and reading and writing.
Finally, we also have the callback
parameter. Once the fs.writeFile()
method has been executed, this function is called.
It is also responsible for throwing an error if the method fails. In the example below, we write some text to a text file named tesla.txt
.
Please note that we have not created the file tesla.txt
; however, when writing to a non-existent file using the fs.writeFile()
API, the file will automatically be created.
const fs = require('fs');
const text = 'Tesla is largest EV manufacturer';
fs.writeFile('tesla.txt', text, (err) => {
if (err) return console.error(err);
console.log('File successfully written !');
});
The code sample was created since the file tesla.txt
did not exist.
However, when writing to a file that exists, the new contents that we are writing will overwrite the pre-existing content.
In the example below, we will add new text content to the tesla.txt
file that we created.
const fs = require('fs');
const text = 'Lucid Motors is a top EV manufacturer !';
fs.writeFile('tesla.txt', text, (err) => {
if (err) return console.error(err);
console.log('File successfully written !');
});
Output:
Lucid Motors is a top EV manufacturer !
As evident in the test.txt
file, the initial content has been overwritten by the new content. We can specify the append flag a
if we write a file with some content.
This flag allows us to write content at the end of a file with some content already.
const fs = require('fs');
const text = 'Tesla is a giant though!';
fs.writeFile('tesla.txt', text, {flag: 'a'}, (err) => {
if (err) return console.error(err);
console.log('File successfully written !');
});
Output:
Lucid Motors is a top EV manufacturer !Tesla is a giant though!
Alternatively, we can also use the fs.appendFile()
to write content to a file that already contains some content.
Using the fs.appendFile()
to Write Content to a File in NodeJS
const fs = require('fs');
const text = 'Tesla is a giant though!';
fs.appendFile('tesla.txt', text, (err) => {
if (err) return console.error(err);
console.log('File successfully written !');
});
Output:
Lucid Motors is a top EV manufacturer !Tesla is a giant though!
The fs module
also offers an asynchronous method fs.writeFileSync()
, that we can use to also write to files in Node js. This method will create a new file if we are writing to do not exist.
When writing to a file containing some content without adding the append flag, the unique contents will also overwrite the old content.
const fs = require('fs')
const content = 'Tesla is the largest EV manufacturer!';
try {
fs.writeFileSync('tesla.txt', content)
} catch (err) {
console.error(err)
}
Output:
Tesla is the largest EV manufacturer!
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn