How to Bulk Update of Documents in MongoDB Using Java
- Prerequisites
- Bulk Update of Documents in MongoDB Using Java
-
Use
updateMany()
to Perform Bulk Update by Adding a New Field to the Existing Documents -
Use
updateMany()
to Perform Bulk Update of Existing Field in Multiple Documents Matching the Filter -
Use
updateMany()
to Perform Bulk Update on Embedded Documents Matching the Filter -
Use
bulkWrite()
API to Update Existing Documents Matching the Filter Query
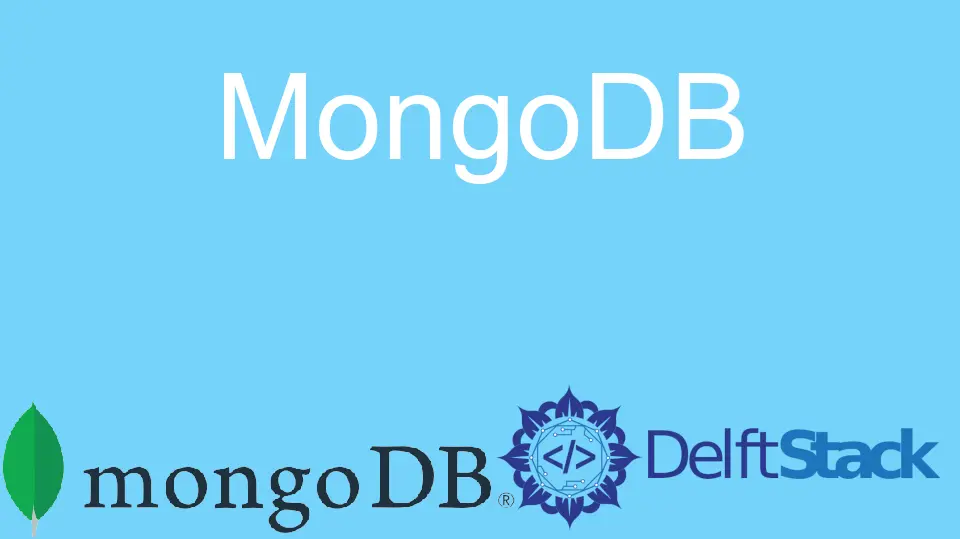
This tutorial is about performing a bulk update of documents in MongoDB using the Java driver. We will also learn how to update by adding a new field, changing the value of the existing field or array, or deleting documents.
Prerequisites
For this tutorial, we are using the following tools that you must have to follow along.
- Java (we are using Java 18.0.1.1)
- MongoDB Server (we are using MongoDB 5.0.8)
- Code Editor or any Java IDE (we are using Apache NetBeans IDE 13)
- Mongo Java Driver Dependencies using Maven or Gradle; we are using it with Maven.
Bulk Update of Documents in MongoDB Using Java
The bulk update is beneficial when we are required to update multiple documents within the database. Now, the question is, how do we want to update the documents?
Do we want to update by adding a new field, updating the value of an existing field or an array, or deleting documents? To learn all these scenarios, let’s create a collection and insert two documents.
Create Collection:
db.createCollection('supervisor');
Insert First Document:
db.supervisor.insertOne(
{
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "Female",
"contact" : {
"email":"mehvishofficial@gmail.com",
"phone" : [
{
"type" : "official",
"number" : "123456789"
},
{
"type" : "personal",
"number" : "124578369"
},
]
},
"entries" : [
{ "_id" : 1, "itemsperday" : [ 1,3,4,5,6,7 ] },
{ "_id" : 2, "itemperday" : [ 2,3,4,5,2,7 ] },
{ "_id" : 3, "itemperday" : [ 5,0,0,4,0,1 ] }
]
}
);
Insert Second Document:
db.supervisor.insertOne(
{
"firstname": "Tahir",
"lastname": "Raza",
"gender": "Male",
"contact" : {
"email":"tahirraza@gmail.com",
"phone" : [
{
"type" : "official",
"number" : "123478789"
},
{
"type" : "personal",
"number" : "122378369"
},
]
},
"entries" : [
{ "_id" : 1, "itemsperday" : [ 4,5,6,7,4,6 ] },
{ "_id" : 2, "itemperday" : [ 2,3,2,7,5,2 ] },
{ "_id" : 3, "itemperday" : [ 5,0,0,1,0,0 ] }
]
}
);
Once you have populated the collection, you can use db.supervisor.find().pretty();
to look at the inserted documents. We will start with easy solutions and move towards a bit tricky but interesting ones.
Use updateMany()
to Perform Bulk Update by Adding a New Field to the Existing Documents
Example Code:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.BasicDBObject;
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
// Example_1
public class Example_1 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// update documents by adding a new field and its value
collection.updateMany(
new BasicDBObject(), new BasicDBObject("$set", new BasicDBObject("status", "Good")));
// print a new line
System.out.println();
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_1
Output:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "Female",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "official", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "Male",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "official", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
We have formatted the output for you (given above), but you will see one document per line on IDE. We can also use the db.supervisor.find().pretty();
on mongo shell to see the updated documents there.
For this example code, we use the updateMany()
method of a mongo collection object that takes the filter
query and an update
statement to update the matched documents. Here, we are not filtering the documents but adding a new field named status
to all existing documents (see the output given above).
Use updateMany()
to Perform Bulk Update of Existing Field in Multiple Documents Matching the Filter
Example Code:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import static com.mongodb.client.model.Filters.eq;
import static com.mongodb.client.model.Updates.combine;
import static com.mongodb.client.model.Updates.set;
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
// Example_2
public class Example_2 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// update the filtered documents by updating the existing field
collection.updateMany(eq("gender", "Female"), combine(set("gender", "F")));
collection.updateMany(eq("gender", "Male"), combine(set("gender", "M")));
System.out.println();
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_2
Output:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "official", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "M",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "official", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
This code example is similar to the previous one, but we are updating a specific field’s value in all documents that match the filter.
We are using the updateMany()
to perform bulk updates for all the documents where the gender
is equal to Female
and gender
is equal to Male
. We are changing the "gender": "Female"
and "gender": "Male"
to "gender":"F"
and "gender":"M"
respectively.
Until now, we have seen how to use the updateMany()
method to add a new field or update the value of an existing field at the first level. Next, we will learn to use updateMany()
to do a bulk update on embedded documents that satisfy the filter query.
Use updateMany()
to Perform Bulk Update on Embedded Documents Matching the Filter
Example Code:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.UpdateOptions;
import org.bson.Document;
import org.bson.conversions.Bson;
// Example_3
public class Example_3 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// filter
Bson where = new Document().append("contact.phone.type", "official");
// update
Bson update = new Document().append("contact.phone.$.type", "assistant");
// set
Bson set = new Document().append("$set", update);
// update collection
collection.updateMany(where, set, new UpdateOptions());
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_3
Output:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "assistant", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "M",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "assistant", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
In this Java program, we use the updateMany()
method to perform a bulk update on embedded documents that match the filter query.
Here, we update the value of the contact.phone.type
from official
to assistant
. To access an array of documents, we use the positional operator ($
) to update the first array value that meets the filter query.
While using the positional operator, we can also specify which array elements are supposed to be updated. We can specify the first, all, or particular array elements to update.
To specify the array elements via positional operator, we use the dot notation
, a property access syntax for navigating BSON
objects. We use the positional operator in the following ways to update first, all or specified array elements.
-
We use the positional operator (
$
) to update the first element of the array that satisfies the filter query, while the array field must be part of the filter query to use the positional operator. -
We use all positional operators (
$[]
) to update all items (elements) of an array. -
The filtered positional operator (
$[<identifier>]
) is used to update those elements of the array that match the filter query. Here, we have to include the array filter in our update operation to tell which array elements need to be updated.Remember that the
<identifier>
is a name we give to our array filter. This value has to start with a lowercase letter and hold alphanumeric characters only.
Use bulkWrite()
API to Update Existing Documents Matching the Filter Query
Example Code:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.DeleteOneModel;
import com.mongodb.client.model.UpdateManyModel;
import com.mongodb.client.model.WriteModel;
import java.util.ArrayList;
import java.util.List;
import org.bson.Document;
// Example_4
public class Example_4 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// create object
List<WriteModel<Document>> writes = new ArrayList<>();
// delete the document matching the filter
writes.add(new DeleteOneModel<>(new Document("firstname", "Tahir")));
// update document matching the filter
writes.add(new UpdateManyModel<>(new Document("status", "Good"), // filter
new Document("$set", new Document("status", "Excellent")) // update
));
// bulk write
collection.bulkWrite(writes);
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
catch (MongoException me) {
System.err.println("An error occurred while running a command: " + me);
} // end catch block
} // end main method
} // end Example_4
Output:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{"type": "assistant", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Excellent"
}
In this example, we are performing multiple operations (delete and update) at once using bulkWrite()
API. The DeleteOneModel()
is used to delete a document that matches the filter, while we are using UpdateManyModel()
to update the value of an already existing field in all those documents that match the filter.