Java를 사용하여 MongoDB에서 문서 대량 업데이트
- 전제 조건
- Java를 사용하여 MongoDB에서 문서 대량 업데이트
-
updateMany()
를 사용하여 기존 문서에 새 필드를 추가하여 대량 업데이트 수행 -
updateMany()
를 사용하여 필터와 일치하는 여러 문서에서 기존 필드의 대량 업데이트 수행 -
updateMany()
를 사용하여 필터와 일치하는 포함된 문서에 대량 업데이트 수행 -
bulkWrite()
API를 사용하여 필터 쿼리와 일치하는 기존 문서 업데이트
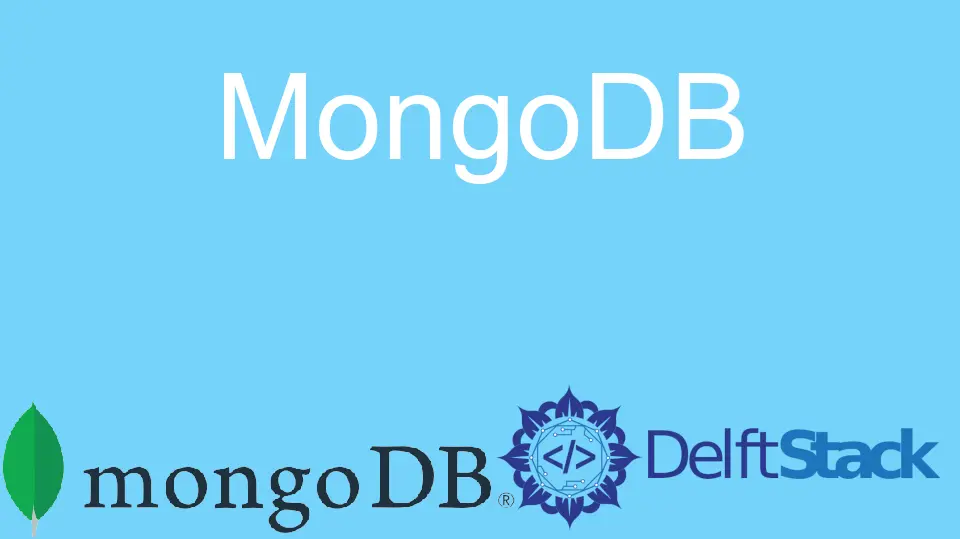
이 튜토리얼은 Java 드라이버를 사용하여 MongoDB에서 문서의 대량 업데이트를 수행하는 방법에 관한 것입니다. 또한 새 필드를 추가하거나 기존 필드 또는 배열의 값을 변경하거나 문서를 삭제하여 업데이트하는 방법을 배웁니다.
전제 조건
이 자습서에서는 따라야 하는 다음 도구를 사용합니다.
- 자바(자바 18.0.1.1 사용)
- MongoDB 서버(MongoDB 5.0.8 사용)
- 코드 편집기 또는 모든 Java IDE(Apache NetBeans IDE 13 사용)
- Maven 또는 Gradle을 사용하는 Mongo Java 드라이버 종속성 우리는 Maven과 함께 사용하고 있습니다.
Java를 사용하여 MongoDB에서 문서 대량 업데이트
대량 업데이트는 데이터베이스 내에서 여러 문서를 업데이트해야 할 때 유용합니다. 이제 문제는 문서를 어떻게 업데이트할까요?
새 필드를 추가하거나 기존 필드 또는 배열의 값을 업데이트하거나 문서를 삭제하여 업데이트하시겠습니까? 이 모든 시나리오를 배우기 위해 컬렉션을 만들고 두 개의 문서를 삽입해 보겠습니다.
컬렉션 만들기:
db.createCollection('supervisor');
첫 번째 문서 삽입:
db.supervisor.insertOne(
{
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "Female",
"contact" : {
"email":"mehvishofficial@gmail.com",
"phone" : [
{
"type" : "official",
"number" : "123456789"
},
{
"type" : "personal",
"number" : "124578369"
},
]
},
"entries" : [
{ "_id" : 1, "itemsperday" : [ 1,3,4,5,6,7 ] },
{ "_id" : 2, "itemperday" : [ 2,3,4,5,2,7 ] },
{ "_id" : 3, "itemperday" : [ 5,0,0,4,0,1 ] }
]
}
);
두 번째 문서 삽입:
db.supervisor.insertOne(
{
"firstname": "Tahir",
"lastname": "Raza",
"gender": "Male",
"contact" : {
"email":"tahirraza@gmail.com",
"phone" : [
{
"type" : "official",
"number" : "123478789"
},
{
"type" : "personal",
"number" : "122378369"
},
]
},
"entries" : [
{ "_id" : 1, "itemsperday" : [ 4,5,6,7,4,6 ] },
{ "_id" : 2, "itemperday" : [ 2,3,2,7,5,2 ] },
{ "_id" : 3, "itemperday" : [ 5,0,0,1,0,0 ] }
]
}
);
컬렉션을 채우고 나면 db.supervisor.find().pretty();
를 사용할 수 있습니다. 삽입된 문서를 볼 수 있습니다. 우리는 쉬운 해결책부터 시작하여 조금 까다롭지만 흥미로운 해결책으로 나아갈 것입니다.
updateMany()
를 사용하여 기존 문서에 새 필드를 추가하여 대량 업데이트 수행
예제 코드:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.BasicDBObject;
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
// Example_1
public class Example_1 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// update documents by adding a new field and its value
collection.updateMany(
new BasicDBObject(), new BasicDBObject("$set", new BasicDBObject("status", "Good")));
// print a new line
System.out.println();
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_1
출력:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "Female",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "official", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "Male",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "official", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
우리는 당신을 위해 출력을 포맷했지만(위에 주어진), IDE에서 한 줄에 하나의 문서를 보게 될 것입니다. db.supervisor.find().pretty();
를 사용할 수도 있습니다. mongo 셸에서 업데이트된 문서를 확인하세요.
이 예제 코드에서는 filter
쿼리와 update
문을 사용하여 일치하는 문서를 업데이트하는 mongo 컬렉션 개체의 updateMany()
메서드를 사용합니다. 여기에서 문서를 필터링하는 것이 아니라 status
라는 새 필드를 모든 기존 문서에 추가합니다(위에 제공된 출력 참조).
updateMany()
를 사용하여 필터와 일치하는 여러 문서에서 기존 필드의 대량 업데이트 수행
예제 코드:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import static com.mongodb.client.model.Filters.eq;
import static com.mongodb.client.model.Updates.combine;
import static com.mongodb.client.model.Updates.set;
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
// Example_2
public class Example_2 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// update the filtered documents by updating the existing field
collection.updateMany(eq("gender", "Female"), combine(set("gender", "F")));
collection.updateMany(eq("gender", "Male"), combine(set("gender", "M")));
System.out.println();
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_2
출력:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "official", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "M",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "official", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
이 코드 예제는 이전 예제와 유사하지만 필터와 일치하는 모든 문서에서 특정 필드 값을 업데이트하고 있습니다.
updateMany()
를 사용하여 gender
가 Female
과 같고 gender
가 Male
과 동일한 모든 문서에 대한 일괄 업데이트를 수행하고 있습니다. "gender": "Female"
및 "gender": "Male"
을 각각 "gender":"F"
및 "gender":"M"
으로 변경합니다.
지금까지 updateMany()
메서드를 사용하여 첫 번째 수준에서 새 필드를 추가하거나 기존 필드 값을 업데이트하는 방법을 살펴보았습니다. 다음으로 updateMany()
를 사용하여 필터 쿼리를 충족하는 포함된 문서에 대한 대량 업데이트를 수행하는 방법을 배웁니다.
updateMany()
를 사용하여 필터와 일치하는 포함된 문서에 대량 업데이트 수행
예제 코드:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.UpdateOptions;
import org.bson.Document;
import org.bson.conversions.Bson;
// Example_3
public class Example_3 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// filter
Bson where = new Document().append("contact.phone.type", "official");
// update
Bson update = new Document().append("contact.phone.$.type", "assistant");
// set
Bson set = new Document().append("$set", update);
// update collection
collection.updateMany(where, set, new UpdateOptions());
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_3
출력:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "assistant", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "M",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "assistant", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
이 Java 프로그램에서 updateMany()
메서드를 사용하여 필터 쿼리와 일치하는 포함된 문서에 대한 대량 업데이트를 수행합니다.
여기에서 contact.phone.type
의 값을 official
에서 assistant
로 업데이트합니다. 문서 배열에 액세스하기 위해 위치 연산자 ($
)를 사용하여 필터 쿼리를 충족하는 첫 번째 배열 값을 업데이트합니다.
위치 연산자를 사용하는 동안 업데이트해야 하는 배열 요소를 지정할 수도 있습니다. 업데이트할 첫 번째, 전체 또는 특정 배열 요소를 지정할 수 있습니다.
위치 연산자를 통해 배열 요소를 지정하기 위해 BSON
개체를 탐색하기 위한 속성 액세스 구문인 점 표기법
을 사용합니다. 다음과 같은 방법으로 위치 연산자를 사용하여 첫 번째, 전체 또는 지정된 배열 요소를 업데이트합니다.
-
위치 연산자(
$
)를 사용하여 필터 쿼리를 충족하는 배열의 첫 번째 요소를 업데이트하는 반면 배열 필드는 위치 연산자를 사용하기 위해 필터 쿼리의 일부여야 합니다. -
모든 위치 연산자(
$[]
)를 사용하여 배열의 모든 항목(요소)을 업데이트합니다. -
필터링된 위치 연산자(
$[<식별자>]
)는 필터 쿼리와 일치하는 배열 요소를 업데이트하는 데 사용됩니다. 여기서 업데이트 작업에 배열 필터를 포함하여 업데이트해야 하는 배열 요소를 알려야 합니다.<identifier>
는 배열 필터에 부여한 이름임을 기억하십시오. 이 값은 소문자로 시작해야 하며 영숫자 문자만 포함해야 합니다.
bulkWrite()
API를 사용하여 필터 쿼리와 일치하는 기존 문서 업데이트
예제 코드:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.DeleteOneModel;
import com.mongodb.client.model.UpdateManyModel;
import com.mongodb.client.model.WriteModel;
import java.util.ArrayList;
import java.util.List;
import org.bson.Document;
// Example_4
public class Example_4 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// create object
List<WriteModel<Document>> writes = new ArrayList<>();
// delete the document matching the filter
writes.add(new DeleteOneModel<>(new Document("firstname", "Tahir")));
// update document matching the filter
writes.add(new UpdateManyModel<>(new Document("status", "Good"), // filter
new Document("$set", new Document("status", "Excellent")) // update
));
// bulk write
collection.bulkWrite(writes);
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
catch (MongoException me) {
System.err.println("An error occurred while running a command: " + me);
} // end catch block
} // end main method
} // end Example_4
출력:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{"type": "assistant", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Excellent"
}
이 예에서는 [bulkWrite()
](https://mongodb.github.io/mongo-java-driver/4.6/apidocs/mongodb-driver-sync/com/mongodb/client/MongoCollection.html#bulkWrite(java.util.List,com.mongodb.client.model.BulkWriteOptions) API를 사용하여 여러 작업(삭제 및 업데이트)을 한 번에 수행합니다. DeleteOneModel()
은 필터와 일치하는 문서를 삭제하는 데 사용되는 반면 UpdateManyModel()
은 필터와 일치하는 모든 문서의 기존 필드 값을 업데이트하는 데 사용됩니다.