How to Generate ObjectId in MongoDB Using Java
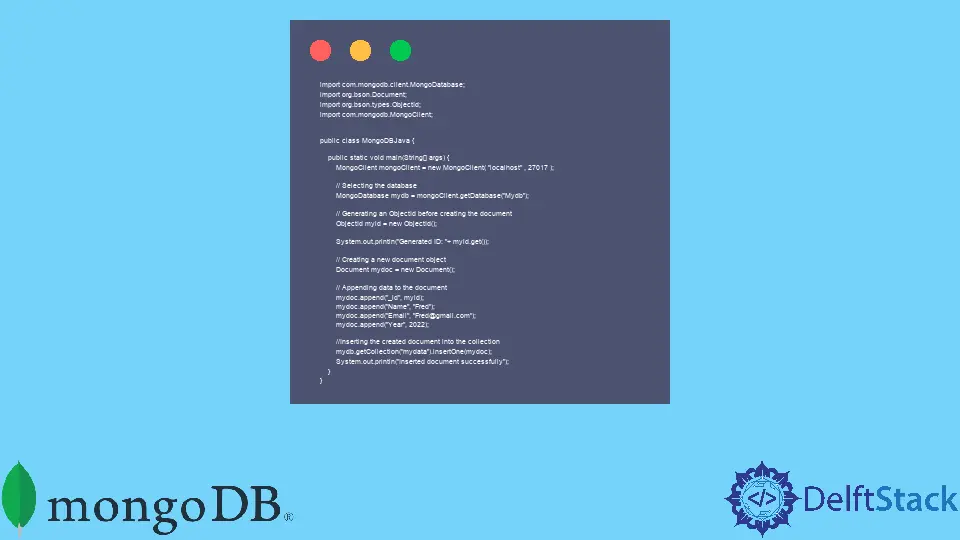
In any database, we need to organize data in a way through which we can easily find that data when necessary. Creating a unique identity is very important for this purpose.
In MongoDB, there is a built-in unique identity system using a field named the ObjectId
.
This article will discuss the ObjectId
and how we can generate this using a Java program. To make the topic easier we will see an example with an explanation to make the topic easier.
ObjectId
in MongoDB
The ObjectId
is a value used to identify a document uniquely through the field _id
in MongoDB. The system automatically generates this field when a user inserts a new document.
But sometimes, we must create our own ObjectId
based on our preferences to provide each document with a unique identity. If you are creating a Java program that can manipulate your MongoDB database, then you can look at the next section on generating an ObjectId
using Java.
Generate ObjectId
Using Java
In our below example, we will demonstrate how we can create a Java program that can insert a document using a manually created ObjectId
. To do this, you can take a look at the below example.
import com.mongodb.MongoClient;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
import org.bson.types.ObjectId;
public class MongoDBJava {
public static void main(String[] args) {
MongoClient mongoClient = new MongoClient("localhost", 27017);
// Selecting the database
MongoDatabase mydb = mongoClient.getDatabase("Mydb");
// Generating an ObjectId before creating the document
ObjectId myid = new ObjectId();
System.out.println("Generated ID: " + myid.get());
// Creating a new document object
Document mydoc = new Document();
// Appending data to the document
mydoc.append("_id", myid);
mydoc.append("Name", "Fred");
mydoc.append("Email", "Fred@gmail.com");
mydoc.append("Year", 2022);
// Inserting the created document into the collection
mydb.getCollection("mydata").insertOne(mydoc);
System.out.println("Inserted document successfully");
}
}
The purpose of each line is commented-out. In the example, we first created a connection with the MongoDB database.
Afterward, we selected the database using the method getDatabase()
and then generated a new ObjectId
with the name myid
.
Then we created a Document
object and append data to it using the append()
method. Lastly, we just inserted the document in our collection using the method insertOne()
.
After executing the above example, you will get the below output.
Generated ID: 6383c46f57dfc2483d89093c
Inserted document successfully
And you will see that the below document has been added to your MongoDB collection.
{ _id: ObjectId("6383c46f57dfc2483d89093c"),
Name: 'Fred',
Email: 'Fred@gmail.com',
Year: 2022 }
Remember that
ObjectId
only supports Hexadecimal format. Also, check if thewrite collection
is selected before running the program. Otherwise, it will show you an error.
Some important notes:
- The program shared in this article is written in Java. So make sure it is installed in your system.
- Install and include the MongoDB
jar
file in your Java program first. To download the MongoDBjar
, use this link.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn