Stack Bar Plots in Matplotlib
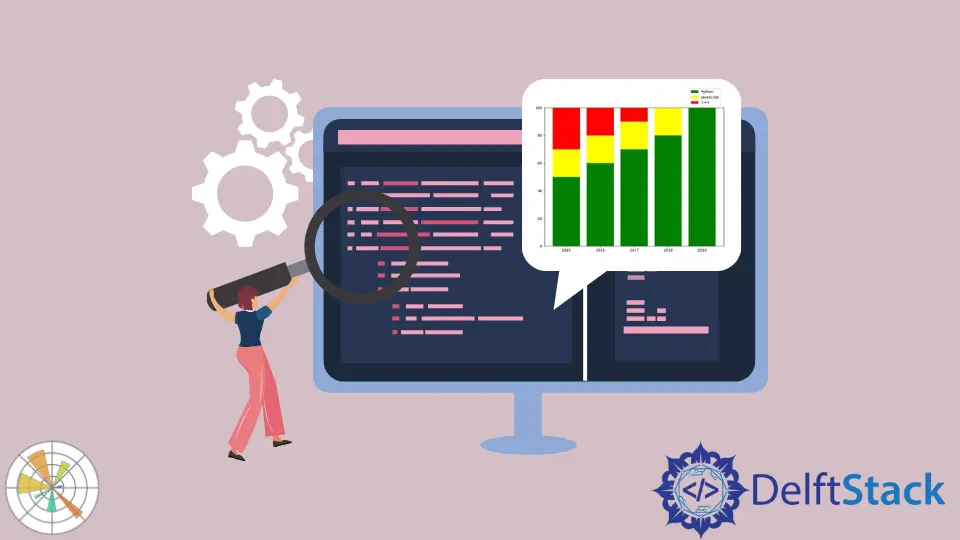
We generate bar plots in Matplotlib using the matplotlib.pyplot.bar()
method. To stack the bar plot of a certain dataset over another, we add all the datasets we need to stack and pass the sum as the bottom
parameter to the bar()
method.
import matplotlib.pyplot as plt
data1 = [30, 20, 10, 0, 0]
data2 = [20, 20, 20, 20, 0]
data3 = [50, 60, 70, 80, 100]
year = ["2015", "2016", "2017", "2018", "2019"]
fig, ax = plt.subplots(3, 1, figsize=(10, 8))
ax[0].bar(year, data1, color="red")
ax[0].legend(["C++"])
ax[1].bar(year, data2, color="yellow")
ax[1].legend(["JavaScript"])
ax[2].bar(year, data3, color="green")
ax[2].legend(["Python"])
plt.show()
Output:
Here, we have three separate bar plots that represent the preference of a Programming language for employees of a company over five years. We will discuss the ways to stack the bar plot of one language over another and study the overall choice of programming languages over the years with a single bar plot.
Stack Bar Plots Matplotlib
import numpy as np
import matplotlib.pyplot as plt
data1 = [30, 20, 10, 0, 0]
data2 = [20, 20, 20, 20, 0]
data3 = [50, 60, 70, 80, 100]
year = ["2015", "2016", "2017", "2018", "2019"]
plt.figure(figsize=(9, 7))
plt.bar(year, data3, color="green", label="Python")
plt.bar(year, data2, color="yellow", bottom=np.array(data3), label="JavaScript")
plt.bar(year, data1, color="red", bottom=np.array(data3) + np.array(data2), label="C++")
plt.legend(loc="lower left", bbox_to_anchor=(0.8, 1.0))
plt.show()
Output:
It stacks the one bar plot on the top of another. In the plot, we first plot the data3
as Python data, which serves as a base for other bars, and then we plot the bar of data2
, and we base bar of data3
as a base for the bar of data2
. To stack bar of data2
upon data3
, we set bottom=np.array(data3)
.
Similarly, while plotting the bar for data1
, we use the bar plot of data2
and data3
as a base. To do so , we set bottom=np.array(data3)+np.array(data2)
while plotting bar of data1
.
An important point to be noted that we must use NumPy arrays to add the data for the bottom
parameter. If we set bottom=data3+data2
, it will create a list by appending the elements of data2
at the end of the list data3
.
If we do not wish to use NumPy arrays, we can use list comprehension to add lists’ corresponding elements.
import numpy as np
import matplotlib.pyplot as plt
data1 = [30, 20, 10, 0, 0]
data2 = [20, 20, 20, 20, 0]
data3 = [50, 60, 70, 80, 100]
year = ["2015", "2016", "2017", "2018", "2019"]
plt.figure(figsize=(9, 7))
plt.bar(year, data3, color="green", label="Python")
plt.bar(year, data2, color="yellow", bottom=data3, label="JavaScript")
plt.bar(
year,
data1,
color="red",
bottom=[sum(data) for data in zip(data2, data3)],
label="C++",
)
plt.legend(loc="lower left", bbox_to_anchor=(0.8, 1.0))
plt.show()
Output:
Stack Bar Plots Matplotlib Using Pandas
We can also use the Pandas
library in Python to generate stacked bar plots in Python.
import pandas as pd
import matplotlib.pyplot as plt
years = ["2015", "2016", "2017", "2018", "2019"]
data = {
"Python": [50, 60, 70, 80, 100],
"JavaScript": [20, 20, 20, 20, 0],
"C++": [30, 20, 10, 0, 0],
}
df = pd.DataFrame(data, index=years)
df.plot(kind="bar", stacked=True, figsize=(10, 8))
plt.legend(loc="lower left", bbox_to_anchor=(0.8, 1.0))
plt.show()
Output:
It generates a stacked bar plot from a Pandas DataFrame where the bar plot of one column is stacked over another for each index in the DataFrame.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn