How to Create Reverse Colormap in Python Matplotlib
- Colormaps in Matplotlib Python
-
Reverse Colormaps in Matplotlib Python With
_r
-
Reverse Colormaps in Matplotlib Python With
matplotlib.colors.Colormap.reversed()
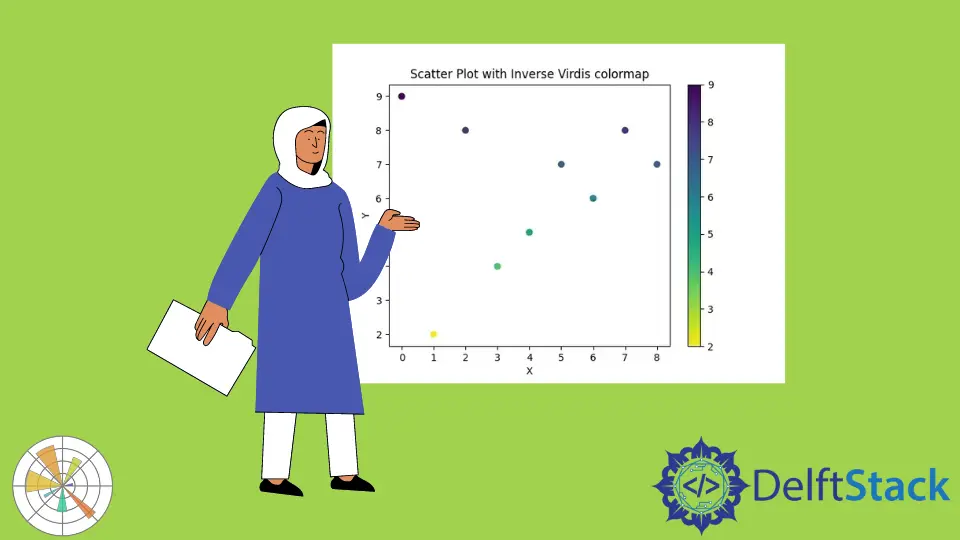
The Colormap is a simple way to map data values to colors. Reversing the color map means reversing the color map of each value. Suppose we have a colormap in which lower values are mapped to yellow
color, and higher values are mapped to red
color. By reversing the colormap, the lower values are now mapped to red
color and higher values to yellow
color.
This tutorial explains various ways of reversing colormaps in Python Matplotlib.
Colormaps in Matplotlib Python
import numpy as np
import matplotlib.pyplot as plt
x = np.arange(9)
y = [9, 2, 8, 4, 5, 7, 6, 8, 7]
plt.scatter(x, y, c=y, cmap="viridis")
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Scatter Plot with Virdis colormap")
plt.colorbar()
plt.show()
Output:
It creates a scatter plot whose y values are mapped to viridis
colormap. It means the points with lower values of y will have the dark blue color, the points with higher values of y will have the yellow color, and intermediate points will have blue
and green
colors.
We can also see a color bar on the right side of the figure, which shows the color mapping for the different values of y.
Reverse Colormaps in Matplotlib Python With _r
We can reverse a colormap by adding _r
at the end of the colormap’s name in Matplotlib. e.g. cmap='viridis_r'
will simply reverse the viridis
colormap.
import numpy as np
import matplotlib.pyplot as plt
x = np.arange(9)
y = [9, 2, 8, 4, 5, 7, 6, 8, 7]
plt.scatter(x, y, c=y, cmap="viridis_r")
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Scatter Plot with Inverse Virdis colormap")
plt.colorbar()
plt.show()
Output:
It creates a scatter plot whose y values are mapped to the inversed viridis
colormap. Hence, the points with higher values in the figure get the dark blue color, and the points with lower values get the yellow color.
We can also see that the color bar at the right has reversed the color in this example.
Reverse Colormaps in Matplotlib Python With matplotlib.colors.Colormap.reversed()
Another way to reverse colormap in Python is to use the matplotlib.colors.Colormap.reversed()
method to create the reversed colormap.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib import cm
x = np.arange(9)
y = [9, 2, 8, 4, 5, 7, 6, 8, 7]
initial_cmap = cm.get_cmap("viridis")
reversed_cmap = initial_cmap.reversed()
plt.scatter(x, y, c=y, cmap=reversed_cmap)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Scatter Plot with Inverse Virdis colormap")
plt.colorbar()
plt.show()
Output:
It also creates a scatter plot whose y values are mapped to the reversed viridis
colormap. The reversed()
method reverses the colormap object to which the method is applied.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib import cm
from matplotlib.colors import ListedColormap
x = np.arange(9)
y = [9, 2, 8, 4, 5, 7, 6, 8, 7]
initial_cmap = cm.get_cmap("viridis")
reversed_cmap = ListedColormap(initial_cmap.colors[::-1])
plt.scatter(x, y, c=y, cmap=reversed_cmap)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Scatter Plot with Inverse Virdis colormap")
plt.colorbar()
plt.show()
Output:
It also creates a scatter plot whose y values are mapped to the reversed viridis
colormap. Any colormap is just a list of colors. We can access the list of colors of a colormap cmap
using cmap.colors
. We then reverse the list and finally convert the reversed list back into a color map using the ListedColormap()
function from the matplotlib.colors
package.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn