How to Color Histogramm in Matplotlib
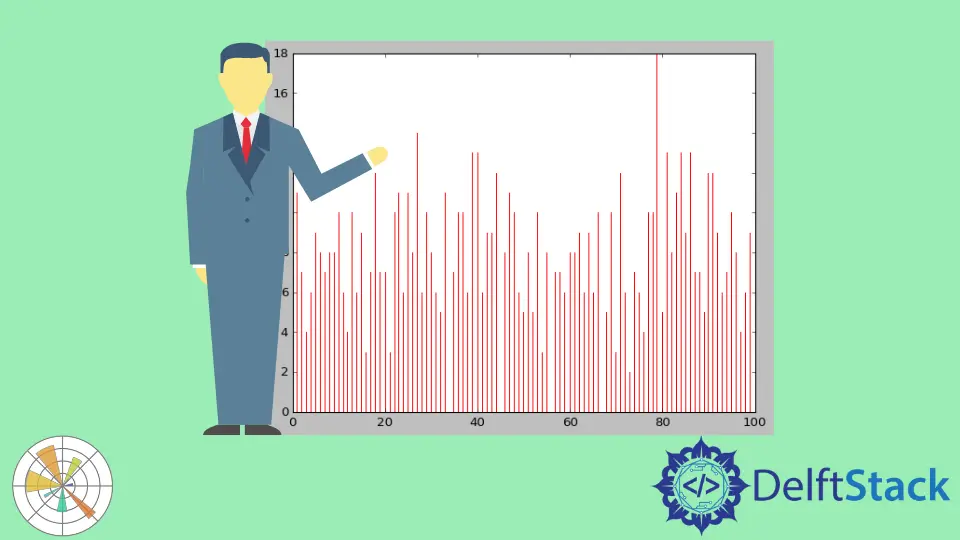
This Tutorial teaches you how to color matplotlib histograms and solve an issue where the bars appear black.
Before we color our histograms, we need to set up our script to work with the library. So we start by importing matplotlib. Furthermore, we load the randrange
function from the random
module to quickly generate some data.
import matplotlib.pyplot as plt
from random import randrange
We continue by setting a style, to see all the styles available check out plt.style.available
.
plt.style.use("classic")
Last but not least, we generate the data in a one-line loop. This won’t generate nice data that makes sense, but it’s good for testing purposes. Keep in mind that the data won’t be the same for you.
data = [randrange(0, 100) for _ in range(0, 800)]
Coloring the Histogram in Matplotlib
Now we make a colored histogram. We just pass the color
argument a color name for doing that. Here lists all named colors available in matplotlib. Don’t also forget to call the show()
function.
plt.hist(data, 3, color="red")
plt.show()
Possible result:
A problem arises when we set a higher amount of bins. As you see in the image, the color is no longer red but black.
This is because the style we used set the border color of the bars to black, and now it’s the only thing we see. To fix this, we either set the bar border width to 0 or its color also to red.
plt.hist(data, 500, color="red", ec="red")
# ec is the edge color
Or,
plt.hist(data, 500, color="red", lw=0)
# lw is the linewidth
Complete code:
import matplotlib.pyplot as plt
from random import randrange
plt.style.use("classic")
data = [randrange(0, 100) for _ in range(0, 800)]
plt.hist(data, 500, color="red", lw=0)
plt.show()
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub