How to Save Plots as PDF File in Matplotlib
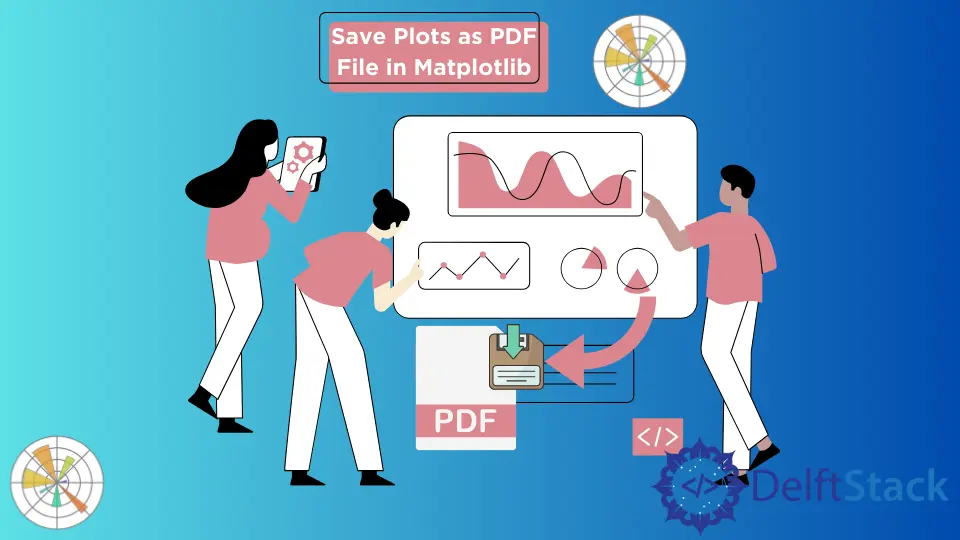
The plots generated from Matplotlib can be simply saved as a PDF file using the .pdf
extension of the filename in the savefig()
method. To save multiple plots in a single PDF file, we use the PdfPages
class.
savefig()
Method to Save Plots as PDF File
We can simply save a plot as an image file in Matplotlib using savefig()
method.
Syntax for savefig()
method:
matplotlib.pyplot.savefig(
fname,
dpi=None,
facecolor="w",
edgecolor="w",
orientation="portrait",
papertype=None,
format=None,
transparent=False,
bbox_inches=None,
pad_inches=0.1,
frameon=None,
metadata=None,
)
The fname
in the parameter section represents the name and path of the file relative to the working directory. If we use .pdf
as the extension to filename, the plot is saved as a PDF file.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 50)
y = np.cos(2 * np.pi * x)
plt.scatter(x, y)
plt.plot(x, y)
plt.title("Plot of cosx")
plt.xlabel("x")
plt.ylabel("cosx")
plt.show()
plt.savefig("Save Plot as PDF file using savefig.pdf")
This saves the generated plot with filename Save Plot as PDF file using savefig.pdf
in the current working directory.
savefig()
Method of the PdfPages
Class
If we wish to plot multiple plots in a single, we can use the savefig()
method of the PdfPages
class.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.backends.backend_pdf import PdfPages
x = np.linspace(-3, 3, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = 1 / (1 + np.exp(-x))
y4 = np.exp(x)
def retFig(x, y):
fig = plt.figure()
a = plt.plot(x, y)
return fig
fig1 = retFig(x, y1)
fig2 = retFig(x, y2)
fig3 = retFig(x, y3)
fig4 = retFig(x, y4)
pp = PdfPages("Save multiple plots as PDF.pdf")
pp.savefig(fig1)
pp.savefig(fig2)
pp.savefig(fig3)
pp.savefig(fig4)
pp.close()
This saves 4 generated figures in Matplotlib in a single PDF file with the file name as Save multiple plots as PDF.pdf
in the current working directory.
Here, we return figures for each plot and then save all figures into a single PDF file by passing individual figures in the savefig()
method of the PdfPages
class.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn