How to Save Plots as an Image File Without Displaying in Matplotlib
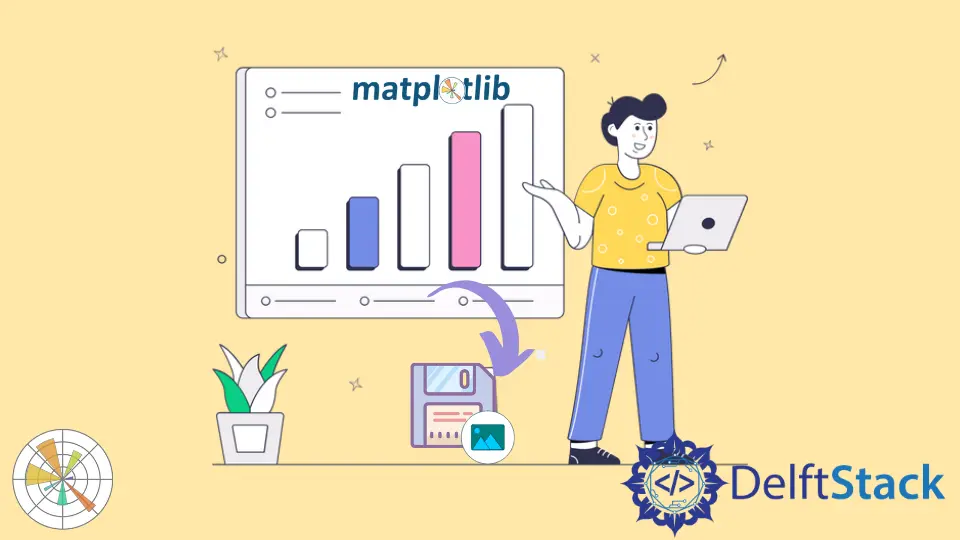
We can simply save plots generated from Matplotlib using savefig()
and imsave()
methods. If we are in interactive mode, the plot might get displayed. To avoid the display of plot we use close()
and ioff()
methods.
Matplotlib savefig()
Method to Save Image
We can save plots generated from Matplotlib using matplotlib.pyplot.savefig(). We can specify the path and format in savefig()
in which plot needs to be saved.
Syntax:
matplotlib.pyplot.savefig(
fname,
dpi=None,
facecolor="w",
edgecolor="w",
orientation="portrait",
papertype=None,
format=None,
transparent=False,
bbox_inches=None,
pad_inches=0.1,
frameon=None,
metadata=None,
)
Here, fname
represents filename relative to the path with respect to the current directory.
Save Plot Without Displaying in Interactive Mode
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.savefig("Plot generated using Matplotlib.png")
This saves the generated plot with the name as Plot generated using Matplotlib.png
in the current working directory.
We can also save plots in other formats like png
, jpg
, svg
, pdf
and many more. Similarly, we can use different arguments of the figsave()
method custom the image.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.savefig("Customed Plot.pdf", dpi=300, bbox_inches="tight")
This saves the plot as Customed Plot.pdf
inside the working directory. Here, dpi=300
represents 300 dots per inch in the saved image, and bbox_inches='tight'
represents no bounding box around output image.
Save Plot Without Displaying in Non-Interactive Mode
However, if we are in interactive mode, the figure is always shown. To avoid this, we forcefully close the figure window to prevent the display of the plot using close()
and ioff()
methods. The interactive mode is set on because of ion()
method.
Avoid Display With close()
Method
matplotlib.pyplot.close
can be used to close the figure window.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
fig = plt.figure()
plt.ion()
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.close(fig)
plt.savefig("Plot generated using Matplotlib.png")
Avoid Display With ioff()
Method
We can turn the interactive mode off using matplotlib.pyplot.ioff()
methods. This prevents figure from being displayed.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
fig = plt.figure()
plt.ion()
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.ioff()
plt.savefig("Plot generated using Matplotlib.png")
matplotlib.pyplot.imsave()
Method to Save Image
We can use matplotlib.pyplot.imsave()
to save arrays as image files in Matplotlib.
import numpy as np
import matplotlib.pyplot as plt
image = np.random.randn(100, 100)
plt.imsave("new_1.png", image)
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn