如何将图另存为图像文件而不在 Matplotlib 中显示
Suraj Joshi
2023年1月30日
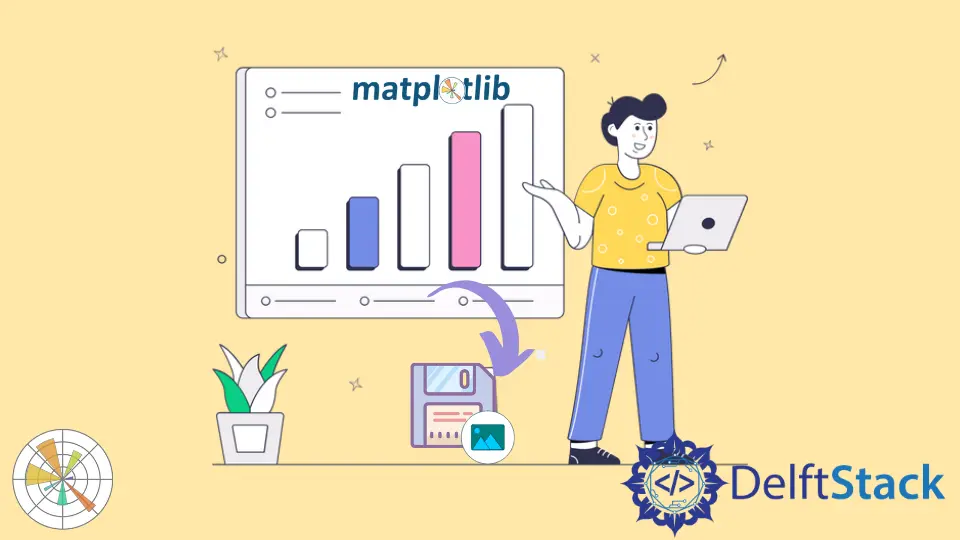
我们可以简单地使用 savefig()
和 imsave()
方法保存从 Matplotlib 生成的图。如果我们处于交互模式,则可能会显示该图。为了避免显示绘图,我们使用 close()
和 ioff()
方法。
Matplotlib 中使用 savefig()
方法保存图像
我们可以使用 matplotlib.pyplot.savefig()
保存从 Matplotlib 生成的图。我们可以在 savefig()
中指定需要保存绘图的路径和格式。
语法:
matplotlib.pyplot.savefig(
fname,
dpi=None,
facecolor="w",
edgecolor="w",
orientation="portrait",
papertype=None,
format=None,
transparent=False,
bbox_inches=None,
pad_inches=0.1,
frameon=None,
metadata=None,
)
在这里,fname
代表相对于当前目录的路径的文件名。
Matplotlib 中保存图形而不以交互模式显示
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.savefig("Plot generated using Matplotlib.png")
这会将生成的图以 Plot generated using Matplotlib.png
的名称保存在当前工作目录中。
我们还可以将图保存为其他格式,例如 png,jpg,svg,pdf 等。同样,我们可以使用 figsave()
方法的不同参数来定制图像。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.savefig("Customed Plot.pdf", dpi=300, bbox_inches="tight")
这会将图另存为工作目录中的 Customed Plot.pdf
。在这里,dpi=300
表示保存的图像中每英寸 300 个点,而 bbox_inches='tight'
表示输出图像周围没有边框。
Matplotlib 中保存图形而不以非交互模式显示
但是,如果我们处于交互模式,则始终显示该图。为了避免这种情况,我们使用 close()
和 ioff()
方法强行关闭图形窗口以防止图形显示。由于使用了 ion()
方法,因此设置了交互模式。
避免使用 close()
方法显示
matplotlib.pyplot.close
可用于关闭图形窗口。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
fig = plt.figure()
plt.ion()
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.close(fig)
plt.savefig("Plot generated using Matplotlib.png")
避免使用 ioff()
方法显示
我们可以使用 matplotlib.pyplot.ioff()
方法关闭交互模式。这样可以防止显示图形。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 5, 100)
y = np.sin(x)
fig = plt.figure()
plt.ion()
plt.plot(x, y)
plt.title("Plot generated using Matplotlib")
plt.xlabel("x")
plt.ylabel("sinx")
plt.ioff()
plt.savefig("Plot generated using Matplotlib.png")
使用 matplotlib.pyplot.imsave()
方法保存图像
我们可以使用 matplotlib.pyplot.imsave()
将数组另存为 Matplotlib 中的图像文件。
import numpy as np
import matplotlib.pyplot as plt
image = np.random.randn(100, 100)
plt.imsave("new_1.png", image)
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn