MATLAB Optional Arguments
-
Understanding
nargin
andvarargin
- Creating Flexible Functions with Optional Arguments
- Best Practices for Using Optional Arguments
- Conclusion
- FAQ
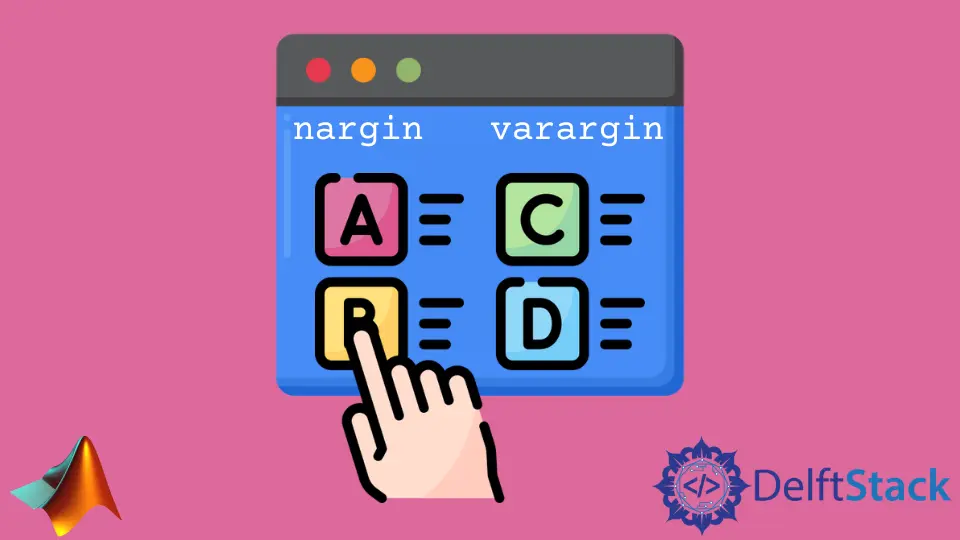
In the world of programming, flexibility often leads to more efficient code. MATLAB, a powerful tool for numerical computing, allows developers to create functions that can accept optional arguments. This can make your functions more versatile and user-friendly. By utilizing the nargin
and varargin
properties, you can tailor your functions to accept a varying number of inputs.
In this article, we will explore how to effectively implement optional arguments in MATLAB, providing you with practical examples and explanations. Whether you’re a beginner or an experienced programmer, understanding how to handle optional arguments can significantly enhance your coding capabilities.
Understanding nargin
and varargin
To grasp how optional arguments work in MATLAB, it’s essential to understand the two primary properties: nargin
and varargin
.
-
nargin
is a function that returns the number of input arguments passed to a function. This allows you to determine if the user has provided the expected number of arguments or if some optional ones are missing. -
varargin
is a cell array that holds any additional input arguments that are not explicitly defined in the function signature. This is where the magic happens, as it allows you to capture an arbitrary number of inputs.
Here’s a simple example to illustrate this concept:
function myFunction(arg1, arg2, varargin)
disp(['Argument 1: ', num2str(arg1)]);
disp(['Argument 2: ', num2str(arg2)]);
if nargin > 2
disp('Optional Arguments:');
for i = 1:length(varargin)
disp(['Optional Argument ', num2str(i), ': ', num2str(varargin{i})]);
end
else
disp('No optional arguments provided.');
end
end
When you call the function with different numbers of arguments, you can see how it behaves:
myFunction(1, 2)
Output:
Argument 1: 1
Argument 2: 2
No optional arguments provided.
myFunction(1, 2, 3, 4, 5)
Output:
Argument 1: 1
Argument 2: 2
Optional Arguments:
Optional Argument 1: 3
Optional Argument 2: 4
Optional Argument 3: 5
In this example, the function myFunction
accepts two mandatory arguments and can take any number of optional arguments. The use of nargin
allows the function to check how many arguments were passed, while varargin
captures any additional inputs.
Creating Flexible Functions with Optional Arguments
Now that we have a basic understanding of nargin
and varargin
, let’s delve deeper into creating flexible functions. The ability to handle optional arguments means you can design functions that adapt to different use cases without having to create multiple versions of the same function.
Consider a scenario where you want to create a function that calculates the area of a rectangle. You might want to allow the user to specify the height and width, but also provide an option to specify a scaling factor. Here’s how you can implement this:
function area = calculateArea(height, width, varargin)
area = height * width;
if nargin > 2
scalingFactor = varargin{1};
area = area * scalingFactor;
disp(['Scaled Area: ', num2str(area)]);
else
disp(['Area: ', num2str(area)]);
end
end
When you run this function with different parameters:
calculateArea(5, 10)
Output:
Area: 50
calculateArea(5, 10, 2)
Output:
Scaled Area: 100
In this example, the calculateArea
function computes the area of a rectangle based on the height and width provided. If an optional scaling factor is given, it scales the area accordingly. This shows how optional arguments can enhance the functionality of your MATLAB functions, making them more adaptable to various scenarios.
Best Practices for Using Optional Arguments
While using optional arguments can greatly enhance your functions, it’s essential to follow some best practices to maintain code clarity and usability. Here are a few tips to consider:
-
Documentation: Always document your functions clearly. Specify which arguments are mandatory and which are optional. This helps users understand how to use your function effectively.
-
Default Values: Consider setting default values for optional arguments. This way, if a user does not provide them, your function can still execute without errors.
-
Input Validation: Implement input validation to ensure that the optional arguments, if provided, are of the expected type or format. This can prevent runtime errors and improve user experience.
-
Keep It Simple: Avoid overloading your functions with too many optional arguments. If you find that your function is becoming too complex, it might be worth considering breaking it into smaller, more focused functions.
By adhering to these best practices, you can ensure that your functions not only work well but are also easy to understand and maintain.
Conclusion
In conclusion, mastering optional arguments in MATLAB through the use of nargin
and varargin
can significantly enhance the flexibility and usability of your functions. By allowing users to provide varying numbers of inputs, you can create more versatile and powerful code. Remember to document your functions, set default values, and validate inputs to ensure a smooth user experience. Whether you’re building simple scripts or complex applications, understanding how to manage optional arguments will undoubtedly elevate your programming skills.
FAQ
-
What is
nargin
in MATLAB?
nargin
is a function that returns the number of input arguments passed to a function. -
How does
varargin
work?
varargin
is a cell array that captures any additional input arguments that are not explicitly defined in the function signature. -
Can I set default values for optional arguments in MATLAB?
Yes, you can set default values by checking the number of arguments passed usingnargin
and assigning default values if necessary. -
What are some best practices for using optional arguments?
Best practices include documenting your functions, setting default values, validating inputs, and keeping functions simple. -
Can optional arguments improve the usability of my functions?
Absolutely! Optional arguments allow for more flexible and user-friendly functions that can adapt to various use cases.