How to Convert Number to String in MATLAB
-
Convert a Number to a String in MATLAB Using the
num2str()
Function -
Convert a Number to a String in MATLAB Using the
int2str()
Function -
Convert a Number to a String in MATLAB Using the
string()
Function - Conclusion
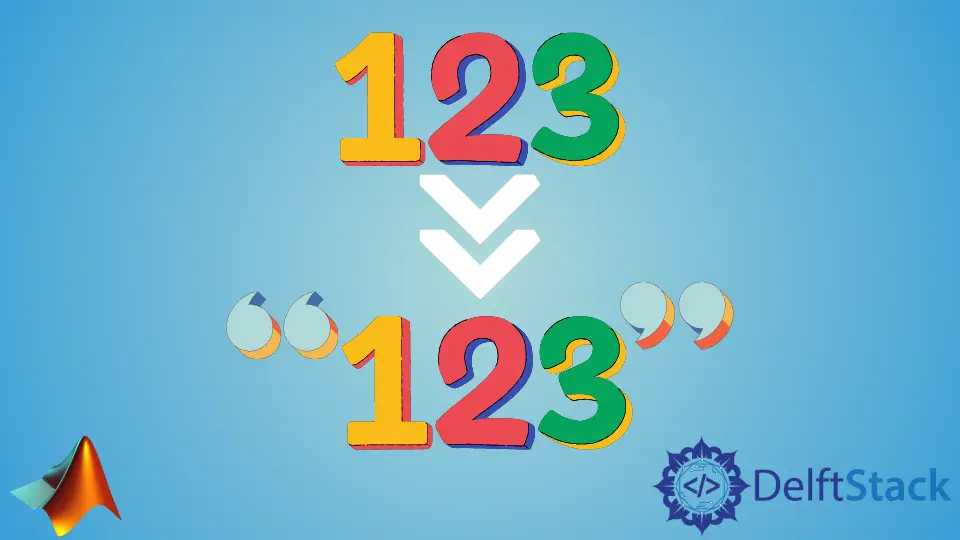
In MATLAB, the need to convert a numerical value to a string arises frequently in programming tasks. Fortunately, MATLAB offers several versatile methods to achieve this conversion, each catering to specific requirements and preferences.
This comprehensive article will explore three primary methods: num2str()
, int2str()
, and string()
. By the end of this guide, you’ll have a thorough understanding of these functions and be equipped to choose the most suitable approach for your MATLAB programming needs.
Convert a Number to a String in MATLAB Using the num2str()
Function
One of the essential functions for converting numerical values into string format for display or further processing is the num2str()
function. This function in MATLAB has a straightforward syntax:
str = num2str(number);
Here, number
is the numerical value you wish to convert to a string, and the result is stored in the variable str
.
Additionally, you can use the num2str()
function with some optional parameters to customize the output. Here are a few variations:
str = num2str(number, formatSpec)
str = num2str(number, precision)
Where:
formatSpec
: Optional parameter that allows you to specify a format for the output string. It can include format specifiers similar to those used in thesprintf
function.precision
: Optional argument that allows you to control the number of significant digits.
The num2str()
function can handle various numeric data types, such as integers, floats, and matrices.
Example 1: Basic Conversion
Let’s start with a basic example. Suppose we have a numerical value, and we want to convert it into a string.
clc
number = 42;
str = num2str(number);
disp(['Number: ' num2str(number)]);
disp(['String: ' str]);
In this example, we showcase the fundamental use of the num2str()
function by converting a basic integer. We assign the value 42
to the variable number
and then employ num2str()
to convert it into a string, storing the result in the variable str
.
The disp
function is used to display both the original number and the resulting string. The output confirms the successful conversion, illustrating that the number 42
has been transformed into the string 42
.
Output:
Number: 42
String: 42
Example 2: Handling Decimal Numbers
The num2str()
function can also handle decimal numbers. Let’s demonstrate this with an example.
clc
decimalNumber = 3.14159;
strDecimal = num2str(decimalNumber);
disp(['Decimal Number: ' num2str(decimalNumber)]);
disp(['String: ' strDecimal]);
This example demonstrates the versatility of num2str()
in handling decimal numbers. We initialize the variable decimalNumber
with the value 3.14159
and proceed to use num2str()
to convert it into a string, storing the result in strDecimal
.
The disp
function then presents both the original decimal number and its string counterpart. The output confirms the successful conversion, showcasing that the decimal number 3.14159
has been accurately transformed into the string 3.14159
.
Output:
Decimal Number: 3.14159
String: 3.14159
Example 3: Handling Arrays
num2str()
is not limited to single numbers; it can handle arrays as well. Here’s an example with an array of integers.
clc
numbersArray = [10, 20, 30, 40];
strArray = num2str(numbersArray);
disp(['Array: ' mat2str(numbersArray)]);
disp(['String Array: ' strArray]);
In this example, we create an array numbersArray
containing integers 10
, 20
, 30
, and 40
. The num2str()
function is applied to the entire array, resulting in a string array stored in the variable strArray
.
The disp
function then displays both the original array and the string array. The output confirms the successful conversion, indicating that the array [10, 20, 30, 40]
has been transformed into the string array '10 20 30 40'
.
Output:
Array: [10, 20, 30, 40]
String Array: 10 20 30 40
Example 4: Combining With Other Functions
You can combine num2str()
with other MATLAB functions for more complex operations. Here, we’ll extract and convert individual digits.
clc
number = 9876;
strNumber = num2str(number);
secondDigit = strNumber(2);
convertedDigit = str2num(secondDigit);
disp(['Number: ' num2str(number)]);
disp(['Second Digit: ' secondDigit]);
disp(['Converted Digit: ' num2str(convertedDigit)]);
Here, we showcase a more advanced application of num2str()
by combining it with other MATLAB functions. We start with the number 9876
, convert it into a string using num2str()
, and then extract the second digit.
The extracted digit is converted back to a number using str2num()
, and all three values are displayed using disp
. The output confirms the successful extraction and conversion, demonstrating that the second digit (8
) has been accurately obtained from the original number 9876
.
Output:
Number: 9876
Second Digit: 8
Converted Digit: 8
Example 5: Handling Numbers in Scientific Notation
This example demonstrates the use of num2str()
with numbers in scientific notation. We will create a number in scientific notation and convert it to a string.
clc
scientificNumber = 6.022e23;
strScientific = num2str(scientificNumber);
disp(['Scientific Number: ' num2str(scientificNumber)]);
disp(['String: ' strScientific]);
Here, we explore the application of num2str()
with numbers represented in scientific notation.
We first initialize a numerical variable, scientificNumber
, with the value 6.022e23
, denoting Avogadro’s number. The num2str()
function is then employed to convert this scientific number into a string, stored in the variable strScientific
.
The disp
function is used to present both the original scientific number and its string representation, demonstrating the successful conversion of the scientific number to a string.
Output:
Scientific Number: 6.022e+023
String: 6.022e+023
Example 6: Custom Formatting
In this example, we showcase the use of sprintf
in combination with num2str()
for custom formatting of the string representation.
clc
number = 3.14159;
formattedString = sprintf('The value is %.2f', number);
disp(['Number: ' num2str(number)]);
disp(['Formatted String: ' formattedString]);
Here, the numerical variable number
is set to 3.14159
, and sprintf
is employed to create a formatted string with two decimal places. The formatted string is then displayed alongside the original number using the disp
function.
This example showcases how the combination of num2str()
and sprintf
allows for precise control over the format of the resulting string.
Output:
Number: 3.14159
Formatted String: The value is 3.14
Example 7: Custom Precision Formatting for Numerical Array
The num2str()
function also allows you to convert a numerical array A
to a string with a specified precision for formatting. Here’s an example demonstrating this scenario:
clc
A = [pi, sqrt(2), 3.56789, 7.12345];
strWithPrecision = num2str(A, 3);
disp(['Original Numerical Array: ' mat2str(A)]);
disp(['Formatted String with Precision: ' strWithPrecision]);
In this example, we start by creating a numerical array A
containing the values of pi
, sqrt(2)
, 3.56789
, and 7.12345
. We then use the num2str()
function with the 3
format specifier to specify a precision of 3 significant digits.
This precision formatting is applied to each element of the numerical array A
. The original numerical array and the formatted string are displayed using the disp
function.
Output:
Original Numerical Array: [3.14159265358979 1.4142135623731 3.56789 7.12345]
Formatted String with Precision: 3.14 1.41 3.57 7.12
In this output, you can observe that the original numerical array is displayed with its default precision, while the formatted string with a precision of 3 significant digits is presented.
Feel free to explore these examples and adapt them to your specific MATLAB programming needs. The num2str()
function provides a convenient way to handle numerical-to-string conversions in various scenarios.
Convert a Number to a String in MATLAB Using the int2str()
Function
In MATLAB, the int2str()
function provides a specific approach for converting integers to strings. Its simple syntax makes it an ideal choice for situations where you need to represent numerical data as strings for display, analysis, or other purposes.
Its syntax is as follows:
str = int2str(number)
Here, number
represents the integer you intend to convert, and str
is the resultant string.
Example 1: Basic Integer Conversion
Let’s walk through a basic example to illustrate the usage of int2str()
:
clc
integerNumber = 75;
strInteger = int2str(integerNumber);
disp(['Integer: ' num2str(integerNumber)]);
disp(['String: ' strInteger]);
In this example, we assign the value 75
to the variable integerNumber
. The int2str()
function is then applied to convert this integer to a string, stored in the variable strInteger
.
The disp
function is used to present both the original integer and the resulting string.
Output:
Integer: 75
String: 75
Example 2: Handling Negative Integers
int2str()
is designed to handle negative integers as well. In this example, we convert a negative integer to a string.
clc
negativeInteger = -123;
strNegativeInteger = int2str(negativeInteger);
disp(['Negative Integer: ' num2str(negativeInteger)]);
disp(['String: ' strNegativeInteger]);
Here, the negative integer -123
is assigned to the variable negativeInteger
. The int2str()
function is then employed to convert this negative integer to a string, stored in the variable strNegativeInteger
.
The disp
function showcases both the original negative integer and the resulting string.
Output:
Negative Integer: -123
String: -123
Example 3: Handling Arrays of Integers
Similar to num2str()
, int2str()
can handle arrays of integers. Let’s explore this capability.
clc
integerArray = [15, -20, 0, 42];
strIntArray = int2str(integerArray);
disp(['Integer Array: ' mat2str(integerArray)]);
disp(['String Array: ' strIntArray]);
In this example, the array [15, -20, 0, 42]
is created and assigned to the variable integerArray
. The int2str()
function is then applied to the entire array, resulting in a string array stored in the variable strIntArray
.
The disp
function presents both the original integer array and the string array.
Output:
Integer Array: [15, -20, 0, 42]
String Array: 15 -20 0 42
While int2str()
is straightforward, it lacks some of the customization options found in other conversion functions. If you need more control over the output format, consider using num2str()
with additional parameters, such as precision and format specifications.
Convert a Number to a String in MATLAB Using the string()
Function
The string()
function in MATLAB is another powerful and flexible utility that allows users to convert different numeric types, including integers and floats, into string format. Its syntax is straightforward:
str = string(number)
Here, number
represents the numeric value you wish to convert, and the resulting string is stored in the variable str
.
Example 1: Basic Conversion
Let’s begin with a basic example demonstrating the conversion of an integer to a string using string()
.
clc
integerNumber = 42;
strInteger = string(integerNumber);
disp(['Integer: ' num2str(integerNumber)]);
disp(['String: ' char(strInteger)]);
In this example, an integer value of 42
is assigned to the variable integerNumber
. The string()
function is then used to convert this integer to a string, stored in the variable strInteger
.
The disp
function displays both the original integer and the resulting string, with char()
used to convert the string to a character array for display.
Output:
Integer: 42
String: 42
Example 2: Handling Decimal Numbers
One notable advantage of the string()
function is its ability to handle various numeric types. Whether you’re working with integers, floats, or other numeric data, the string()
function adapts, providing a unified approach to numeric-to-string conversion.
In this example, we convert a decimal number to a string.
clc
decimalNumber = 3.14159;
strDecimal = string(decimalNumber);
disp(['Decimal Number: ' num2str(decimalNumber)]);
disp(['String: ' char(strDecimal)]);
Here, the decimal number 3.14159
is assigned to the variable decimalNumber
. The string()
function is applied to convert this decimal number to a string, stored in the variable strDecimal
.
The disp
function showcases both the original decimal number and the resulting string.
Output:
Decimal Number: 3.1416
String: 3.1416
Example 3: Handling Arrays of Numbers
Similar to previous functions, string()
can handle arrays of numbers. Let’s explore this with an example.
clc
numberArray = [10, 20, 30, 40];
strArray = string(numberArray);
disp(['Number Array: ' mat2str(numberArray)]);
disp(['String Array: ' strjoin(cellstr(strArray), ' ')]);
In this example, an array [10, 20, 30, 40]
is assigned to the variable numberArray
. The string()
function is then applied to the entire array, resulting in a string array stored in the variable strArray
. The disp
function showcases both the original number array and the string array, with cellstr()
and strjoin()
used for appropriate formatting.
Output:
Number Array: [10, 20, 30, 40]
String Array: 10 20 30 40
These examples showcase the usage of the string()
function in MATLAB, providing an alternative method for converting numerical values to strings.
Conclusion
MATLAB provides multiple methods to convert a number to a string, offering flexibility to cater to diverse programming requirements.
The num2str()
function is a general-purpose tool suitable for converting both integers and decimals, and it supports array transformations. If you specifically work with integers, the int2str()
function offers a specialized solution.
Additionally, the string()
function provides an alternative, handling various numeric scenarios and supporting seamless integration with other MATLAB functionalities.
Whether you need basic conversions, precision formatting, or intricate operations involving arrays and character manipulations, MATLAB’s comprehensive set of conversion functions ensures that you can effortlessly transform numerical values into string representations.
Choose the method that aligns with your specific needs and the nature of your numerical data to optimize your MATLAB programming experience.