MATLAB Line Continuation
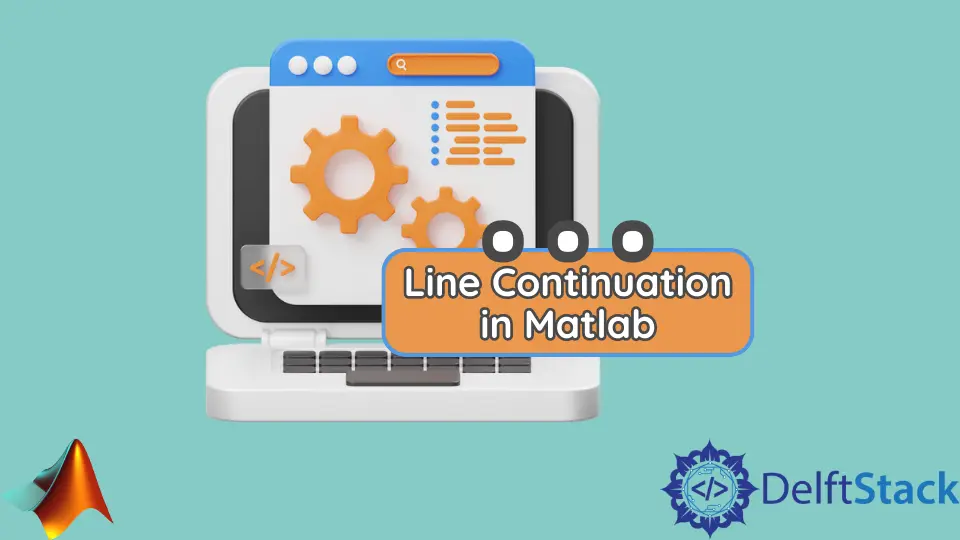
This tutorial will discuss how to continue a line using 3 dots (.
) symbols in Matlab.
MATLAB Line Continuation
Sometimes, while writing code in Matlab, we have to write a long line of code which is not good if we want to view the whole code. In Matlab, we can use the 3 dots ...
in a line after a comma, then move to the following line and continue writing the code.
For example, let’s define a vector using the 3 dots. See the code below.
My_string = [1,2,3,...
4,5]
Output:
My_string =
1 2 3 4 5
In the above code, we passed 3 values in the vector in the first line and then moved to the following line using the 3 dots after that comma and passed two more values. We can see in the above output that all the values are added to the same variable.
We can also do this operation in case of function parameters, defining other data types, and so on. For example, if we want to write a long string and we don’t want to write the whole string in a single line, we can write the string in the first line, and at the end of the line, we can add a comma and 3 dots and move to the next line and write the next line of string on the second line and so on.
For example, let’s define a string using the 3 dots. See the code below.
My_string = ['hello world',...
' hello']
Output:
My_string =
'hello world hello'
Matlab automatically saved all the words as a single string in the output above because we defined the string using single quotations. If we define the strings using double quotation marks, the output will not be a single string.
So, we must define the strings in single quotation marks or as character arrays for the above method to work. In the above code, if we want to write another line of string, we have to add a comma and three dots at the end of the second line, and then we can move to the third line.
We can also do the above operation using the strcat()
function, which is used to concatenate strings. We have to save each line of string in a unique variable, and then we can pass all the variables inside the strcat()
function, which will join them and return a single string.
Using the strcat()
function, we don’t have to worry about the single and double quotations because this function always returns a single string. For example, let’s repeat the above example using the strcat()
function.
See the code below.
s1 = 'hello'
s2 = ' world'
s3 = ' hello'
s4 = strcat(s1,s2,s3)
Output:
s1 =
'hello'
s2 =
' world'
s3 =
' hello'
s4 =
'hello world hello'
In the above code, we have to add space at the start of the stings manually; otherwise, the words will be joined together without space. If we want to join strings using space or any other delimiter, we can use the join()
function of Matlab.
We must ensure the strings are defined with double quotation marks and inside a vector. By default, the join()
function adds space between each string, but if we want to add another delimiter, we can pass the delimiter as the second argument inside the join()
function.
For example, let’s join the above strings without adding a space at the start using the join()
function. See the code below.
s = ["hello world",...
"hello"]
s1 = join(s)
s2 = join(s,'-')
Output:
s =
1×2 string array
"hello world" "hello"
s1 =
"hello world hello"
s2 =
"hello world-hello"
We joined the given strings in the above code using space and the -
delimiter. We can see in the output that the given string is not a single string because it is defined with double quotation marks.
If we define strings using single quotation marks or curly brackets, we cannot use the join()
function. In the case of strings defined using curly brackets and single quotation marks, we can use the strjoin()
function instead of the join()
function to attach the strings in the given array.
For example, let’s repeat the above example using the curly brackets and single quotation marks. See the code below.
clc
clear
s = {'hello world',...
'hello'}
s1 = strjoin(s)
s2 = strjoin(s,'-')
Output:
s =
1×2 cell array
{'hello world'} {'hello'}
s1 =
'hello world hello'
s2 =
'hello world-hello'
We cannot define the above strings using double quotation marks because Matlab will give an error that says that the first input must be a string array or cell array of character vectors.