How to Concatenate String in MATLAB
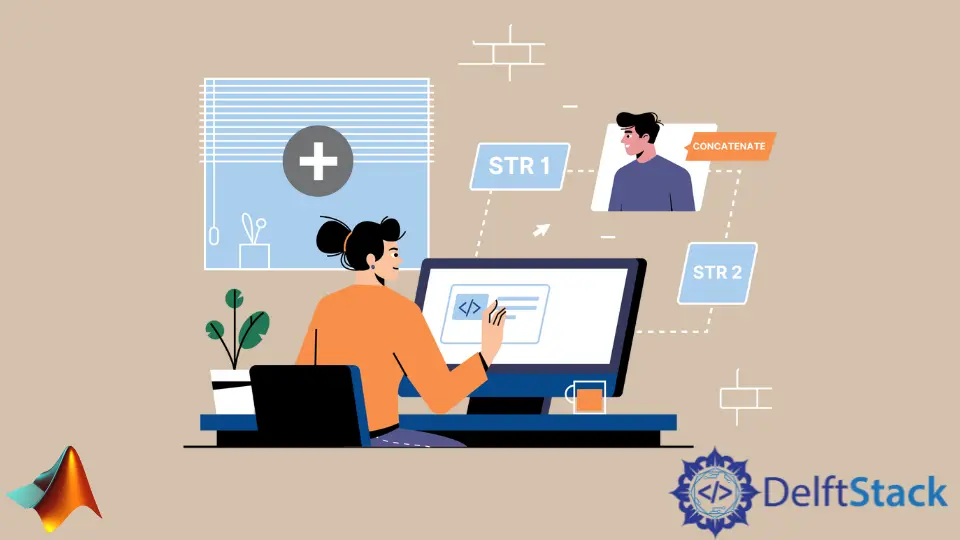
This tutorial will discuss concatenating strings using the function strcat()
in Matlab.
Concatenate Strings Using the strcat()
Function in MATLAB
To compare two strings, we can use the Matlab built-in function strcat()
. We need to pass the strings that we want to concatenate inside the function to concatenate them. For example, Let’s create two strings and join them using the function strcat()
in Matlab. See the code below.
s1 = "Hello"
s2 = "World"
s3 = strcat(s1,s2)
Output:
s1 =
"Hello"
s2 =
"World"
s3 =
"HelloWorld"
In the output, the two strings s1 and s2, have been concatenated and saved in s3. We can also concatenate two cell arrays using the strcat()
function. In the case of cell arrays, the function will join the first entry of the first cell array with the first entry of the second cell array and the second entry of the first cell array with the second entry of the second cell array. For example, let’s create two cell arrays containing strings and concatenate them using the strcat()
function. See the code below.
s1 = {'Hello', 'Day'};
s2 = {'World', '10'};
s3 = strcat(s1,s2)
Output:
s3 =
1×2 cell array
{'HelloWorld'} {'Day10'}
The variable s3 contains two elements in the output because there are two elements in each cell array. The cell array should be of the same size. Otherwise, there will be an error. As you can see, there is no space between the strings when they are concatenated, but we can put it using a third cell array that will contain the space. For example, let’s put a pace between the above strings using a third cell array. See the code below.
s1 = {'Hello', 'Day'};
s2 = {'World', '10'};
space = {' '};
s3 = strcat(s1,space,s2)
Output:
s3 =
1×2 cell array
{'Hello World'} {'Day 10'}
In the output, the two strings now have a space between them. We can put any string as we like between the two strings, like a comma or full stop, etc. We can also put space inside the strings s1 or s2 instead of putting it separately. You can also use the + operator to concatenate two strings instead of the strcat()
function, but make sure you use double quotation marks to define the strings. Otherwise, the result will be numeric because if you define the strings in single quotation marks, Matlab will consider them as character vectors. For example, define two strings using the double quotation marks and two strings using the single quotation marks, and concatenate them using the + operator. See the code below.
s1 = 'Hello';
s2 = 'World';
s3 = s1+s2
ss1 = "Hello";
ss2 = "World";
ss3 = ss1+ss2
Output:
s3 =
159 212 222 216 211
ss3 =
"HelloWorld"
The first output is numeric because of single quotation marks, but the second output is in string form because of double quotation marks.