How to Insert Variable Into String in MATLAB
-
Insert Variable Into String in MATLAB Using
num2str()
andstrcat()
-
Insert Variable Into String in MATLAB Using
string()
-
Insert Variable Into String in MATLAB Using
join()
andstrjoin()
-
Insert Variable Into String in MATLAB Using
sprintf()
- Insert Variable Into String in MATLAB Using String Interpolation
- Conclusion
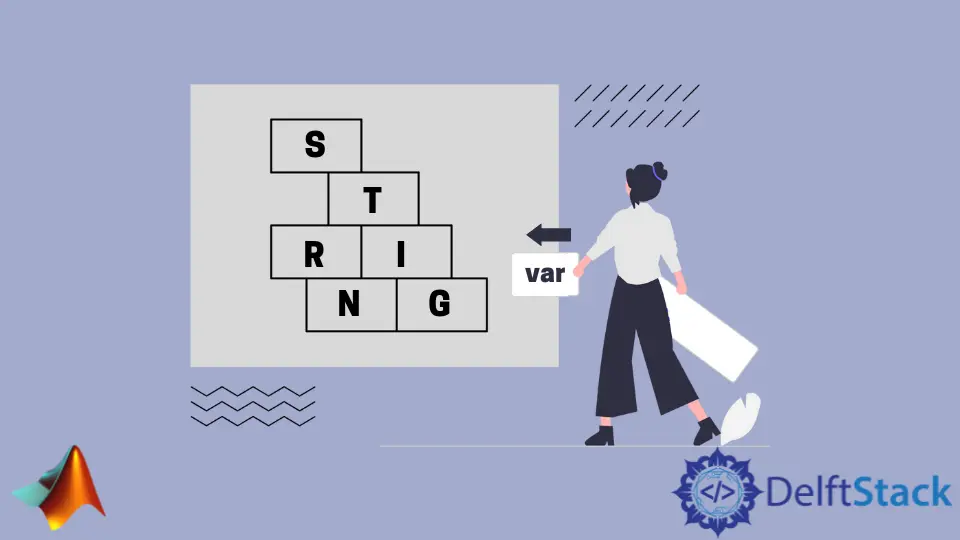
When working with MATLAB, you often need to create dynamic strings that incorporate variable values. Whether you’re building user messages, generating reports, or formatting data for display, the ability to insert variables into strings is a fundamental skill.
In this article, we’ll explore various methods to achieve this in MATLAB, ranging from simple string concatenation to more advanced techniques.
Insert Variable Into String in MATLAB Using num2str()
and strcat()
In MATLAB, you can insert variable values into strings using a combination of the num2str()
function and the strcat()
function.
The num2str()
function in MATLAB is used to convert numeric values into their string representations. It is particularly useful when you want to insert numeric variables into a string, as it handles the conversion seamlessly.
The syntax of the num2str
function in MATLAB is as follows:
str = num2str(X, format)
str
: The resulting string.X
: The numeric value or variable you want to convert into a string.format
: An optional argument that allows you to specify additional formatting options. This argument can be used to control the appearance of the string, such as the number of decimal places or the format type (e.g.,%f
for fixed-point notation,%e
for scientific notation, etc.).
On the other hand, the strcat()
function is used to concatenate multiple strings together. It can be employed to combine string literals and variables effectively, creating a dynamic string with variable values.
The basic syntax for using strcat()
is as follows:
result = strcat('string1', 'string2', ...);
result
: The resulting string that concatenates the input strings.'string1', 'string2', ...
: These are the strings you want to concatenate. You can specify multiple input strings, and they will be concatenated in the order you provide.
Let’s start with a simple example where we insert a floating-point number into a string:
clc
clear
n = 100.577;
s1 = num2str(n);
s2 = "value is ";
s3 = strcat(s2, s1);
disp(s3);
In this code, num2str(n)
converts the floating-point number n
into a string, and then strcat()
combines this string with another one. The output will be:
s3 =
"value is 100.577"
Set Precision and Format Significant Digits
num2str()
provides options for controlling the precision or the number of significant digits when converting a numeric value into a string. The second argument of num2str()
is used to set the precision, and it should be a positive integer.
For example, you can convert a floating-point number while limiting the string to display only a certain number of decimal places:
clc
clear
n = 10.212;
s1 = num2str(n, 3);
s2 = "value is ";
s3 = strcat(s2, s1);
disp(s3);
Output:
s3 =
"value is 10.2"
Here, by setting the precision to 3
, we obtain a string with only one decimal place.
Additionally, you can format the number to display a specific number of significant digits:
clc
clear
n = 10.218;
s1 = num2str(n, '%0.2f');
s2 = "value is ";
s3 = strcat(s2, s1);
disp(s3);
Output:
s3 =
"value is 10.22"
In this case, we’ve rounded the number to two significant digits using the %0.2f
format specifier. This format specifier is applied to control the number of significant digits in floating-point numbers.
It’s worth noting that you can define strings using both single and double quotation marks in MATLAB. However, when using single quotes, be cautious about adding spaces or other characters between variables and strings.
Here’s an example illustrating the issue:
clc
clear
n = 10.218;
s1 = num2str(n, '%0.2f');
s2 = 'value is ';
s3 = strcat(s2, s1);
disp(s3);
Output:
s3 =
'value is10.22'
In this example, we’ve added a space in the s2
string, but the space is not present in the s3
string.
If we use the plus sign (+
) for concatenation, the output will be the same. To avoid this issue, ensure that at least one of the strings being concatenated is a string, not a character vector or array.
While num2str()
and strcat()
are powerful tools, if you prefer a more versatile approach to handling strings, you can use the string()
function.
Insert Variable Into String in MATLAB Using string()
In MATLAB, one of the most flexible and convenient methods for inserting variable values into strings is by using the string()
function. This function is used to create string objects.
A string object is a sequence of characters enclosed in double quotes ("
). These objects are more versatile than character arrays and support various operations for manipulating and formatting strings.
You can use string()
to efficiently insert variable values into strings.
The basic syntax for using string()
is as follows:
result = string(expression)
result
: The variable where the string object will be stored.expression
: This can be a character vector, a numeric value, a cell array, or other data types that can be converted into a string.
Here’s a simple example:
name = 'John';
age = 30;
sentence = string("My name is " + name + " and I am " + age + " years old.");
disp(sentence);
In this code, the string()
function creates a string object by concatenating string literals and variables with the +
operator. The output will be:
My name is John and I am 30 years old.
You can use string()
with various data types, making it a versatile tool for inserting variable values into strings.
Inserting string variables is straightforward:
product = 'laptop';
price = '$1000';
message = string("The " + product + " costs " + price + ".");
You can insert numeric variables without explicit conversion:
radius = 5;
area = pi * radius^2;
result = string("The area of a circle with a radius of " + radius + " is " + area + " square units.");
You can also use string()
to format and insert values from arrays:
fruits = {'apple', 'banana', 'cherry'};
list = string("My favorite fruits are " + strjoin(fruits, ', ') + ".");
In this example, we use the strjoin()
function to concatenate the elements of the fruits
cell array and insert them into the string.
You can format and insert values from structures as well:
person.name = 'Alice';
person.age = 25;
greeting = string("Hello, my name is " + person.name + ", and I am " + person.age + " years old.");
Now, if we have a long array of characters or strings and want to join them and include a space or another delimiter between them, we can use the join()
and strjoin()
functions.
Insert Variable Into String in MATLAB Using join()
and strjoin()
Creating dynamic strings that incorporate variable values can also be accomplished using the functions join()
and strjoin()
. These functions are especially useful when you need to concatenate string arrays or cell arrays of strings, making your code more readable and maintainable.
Both join()
and strjoin()
are MATLAB functions designed for combining strings, but they differ in the types of input they accept.
join()
works with string arrays. It concatenates the elements of a string array, optionally inserting a delimiter between them.
Meanwhile, strjoin()
is used for cell arrays of strings. It concatenates the elements of a cell array of strings, also with an optional delimiter.
These functions can significantly simplify the task of inserting variable values into strings, especially when you’re working with collections of strings. Let’s dive into how to use each of them.
Using join()
for String Arrays
Suppose you have a string array and you want to insert variable values into a string:
names = ["Alice", "Bob", "Charlie"];
age = 25;
greeting = join(["Hello, my name is " names " and I am " num2str(age) " years old."]);
disp(greeting);
In this example, we create a string array named names
. Using join()
, we concatenate the elements of the array along with the variable age
, inserting a space as a delimiter.
The result is a single string that incorporates the array elements and variable value.
Using strjoin()
for Cell Arrays of Strings
If you’re working with a cell array of strings, you can use strjoin()
in a similar manner:
fruits = {'apple', 'banana', 'cherry'};
sentence = strjoin(['My favorite fruits are' fruits 'and they are delicious.']);
disp(sentence);
In this case, we have a cell array of strings, fruits
. The strjoin()
function concatenates these strings with the specified text and space as delimiters.
The resulting string includes the variable values and the elements of the cell array.
Both join()
and strjoin()
allow you to specify custom delimiters to control how the elements and variables are separated in the final string. By default, they use a space as the delimiter, but you can change it as needed.
For instance:
names = ["Alice", "Bob", "Charlie"];
delimiter = ", ";
greeting = join(["Hello, my name is " names " and I am " num2str(age) " years old."], delimiter);
In this example, we use a comma and space as the delimiter, resulting in a string like "Hello, my name is Alice, Bob, Charlie, and I am 25 years old."
Insert Variable Into String in MATLAB Using sprintf()
In MATLAB, the sprintf()
function is a versatile tool for formatting strings and embedding variable values within them. Whether you want to create custom output messages, generate data reports, or format strings for display, sprintf()
allows you to efficiently combine text and variables in a structured manner.
This function has the following syntax:
formatted_string = sprintf(format, A, B, ...)
formatted_string
: Resulting formatted string where the placeholders in theformat
string are replaced by the values ofA
,B
, and so on.format
: Character vector or string specifying how the variablesA
,B
, etc., should be formatted within the output string. Placeholders for variables are indicated by format specifiers (e.g.,%s
for strings,%d
for integers,%f
for floating-point numbers, etc.).A, B, ...
: Variables or values that you want to insert into theformatted_string
. The number of variables should match the number of placeholders in theformat
string.
The sprintf()
function in MATLAB allows you to format strings by specifying placeholders for variables. These placeholders, often referred to as format specifiers, are represented by percent signs followed by conversion characters.
When sprintf()
is executed, it replaces these placeholders with the corresponding variable values. Here’s a basic example:
name = 'John';
age = 30;
message = sprintf('My name is %s and I am %d years old.', name, age);
disp(message);
When you run this code, MATLAB replaces %s
with the value of the name
variable and %d
with the value of the age
variable. The output will be:
My name is John and I am 30 years old.
sprintf()
works with various data types, including strings, numbers, and more complex structures. Let’s explore how to use sprintf()
for different data types.
You can easily format and insert string variables within your text:
product = 'laptop';
price = '$1000';
message = sprintf('The %s costs %s.', product, price);
sprintf()
can handle numeric variables without the need for explicit conversion:
radius = 5;
area = pi * radius^2;
result = sprintf('The area of a circle with a radius of %d is %f square units.', radius, area);
You can use sprintf()
with arrays to create dynamic output:
fruits = {'apple', 'banana', 'cherry'};
list = sprintf('My favorite fruits are %s, %s, and %s.', fruits{:});
You can format and insert values from structures:
person.name = 'Alice';
person.age = 25;
greeting = sprintf('Hello, my name is %s, and I am %d years old.', person.name, person.age);
Format Specifiers
In order to format variables correctly, it’s important to understand format specifiers:
%s |
String |
%d or %i |
Signed integer |
%f |
Floating-point number |
%e or %E |
Scientific notation |
%g or %G |
Compact decimal or scientific notation |
%u |
Unsigned integer |
%c |
Character |
%x or %X |
Hexadecimal representation |
%o |
Octal representation |
You can also use additional flags for formatting, like specifying field width, precision, and alignment. For example:
value = 123.4567;
formatted = sprintf('%.2f', value); % Formats the number with 2 decimal places
Check this link for more details about the sprintf()
function.
Insert Variable Into String in MATLAB Using String Interpolation
String interpolation is a powerful feature introduced in MATLAB R2016b and later versions that simplifies the process of inserting variable values into strings. It involves embedding variables directly into string literals using double quotes ("
).
The variables are indicated by a dollar sign and curly braces (${variable}
) within the string. When the string is evaluated, these placeholders are replaced with the actual values of the corresponding variables.
Here’s a simple example:
name = 'John';
age = 30;
sentence = "My name is ${name} and I am ${age} years old.";
disp(sentence);
When you run this code, MATLAB will replace ${name}
with the value of the name
variable and ${age}
with the value of the age
variable.
The output will be:
My name is John and I am 30 years old.
String interpolation works with various data types, including strings, numbers, and even more complex data structures like arrays or structures. Let’s dive deeper into the details of using string interpolation effectively.
You can easily interpolate string variables within your text. For example:
product = 'laptop';
price = '$1000';
message = "The ${product} costs ${price}.";
String interpolation can handle numeric variables without the need for explicit conversion:
radius = 5;
area = pi * radius^2;
result = "The area of a circle with a radius of ${radius} is ${area} square units.";
String interpolation can also be used with arrays to create more dynamic output:
fruits = {'apple', 'banana', 'cherry'};
list = "My favorite fruits are ${fruits{1}}, ${fruits{2}}, and ${fruits{3}}.";
You can even interpolate values from structures:
person.name = 'Alice';
person.age = 25;
greeting = "Hello, my name is ${person.name}, and I am ${person.age} years old.";
If you need to include a literal dollar sign or curly braces within your string, you can escape them by doubling the characters ($$
for a dollar sign and {{
or }}
for curly braces). For example:
amount = 100;
message = "The cost is $$${amount}";
In this case, ${amount}
is not treated as a variable interpolation, and the output will be:
The cost is $100
Conclusion
Inserting variable values into strings is a common and essential task in MATLAB programming. The method you choose depends on your specific requirements and coding style.
Simple concatenation, sprintf()
, string()
, and specialized functions like join()
and strjoin()
offer flexibility and efficiency for different use cases. When crafting MATLAB code, consider the readability, maintainability, and performance of your chosen approach, and select the method that best suits your needs.