MATLAB Diagonal Matrix
-
Make Diagonal Matrix Using
diag()
Function in MATLAB -
Make Diagonal Matrix Using
spdiags()
Function in MATLAB
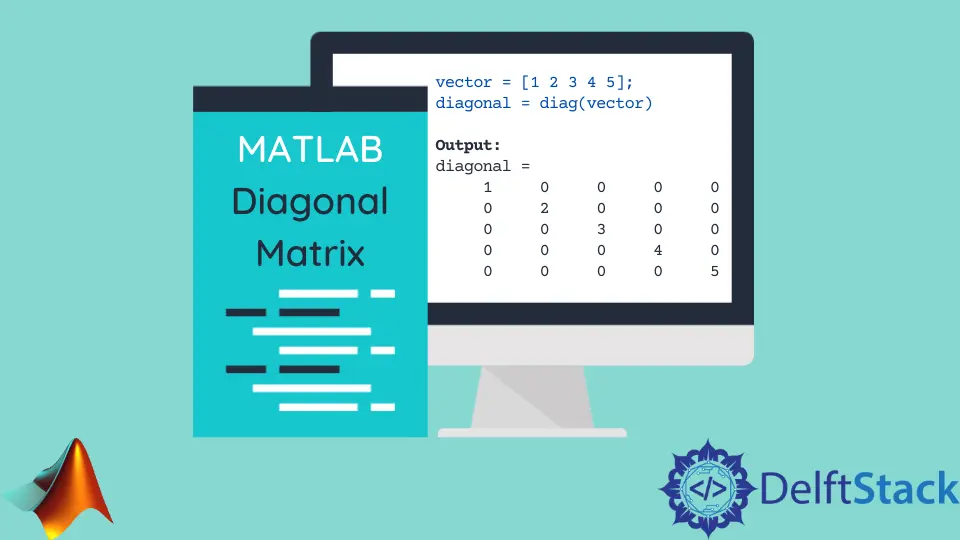
In this tutorial, we will discuss how to make a diagonal matrix using the diag()
and spdiags()
function in MATLAB.
Make Diagonal Matrix Using diag()
Function in MATLAB
To make a diagonal matrix or to get the diagonal entries of a matrix, you can use the diag()
function in MATLAB. For example, let’s make a diagonal matrix from a given vector. See the code below.
vector = [1 2 3 4 5];
diagonal = diag(vector)
Output:
diagonal =
1 0 0 0 0
0 2 0 0 0
0 0 3 0 0
0 0 0 4 0
0 0 0 0 5
In the above code, we made a 5x5
diagonal matrix with the diagonal entries stored in the variable vector
. The diag()
function creates a square matrix depending on the number of elements present inside the given vector. If the number of elements is 5, then the diagonal matrix will be 5x5
. You can also change the position of the diagonal by using a second argument inside the diag()
function. If the second argument is equal to 0, the vector will be placed on the main diagonal. If the second argument is greater than 0, the vector will be placed above the main diagonal. If the second argument is less than 0, the vector will be placed below the main diagonal. For example, let’s place the vector above the main diagonal. See the code below.
vector = [1 2 3 4 5];
diagonal = diag(vector,1)
Output:
diagonal =
0 1 0 0 0 0
0 0 2 0 0 0
0 0 0 3 0 0
0 0 0 0 4 0
0 0 0 0 0 5
0 0 0 0 0 0
In the above code, we created a diagonal matrix with the elements placed above the main diagonal. As you can see, the size of the diagonal is 6x6
now because the vector is placed above the main diagonal, and to ensure all the elements are placed inside the matrix, the size of the matrix increased. You can also use the diag()
function to get the diagonal entries from a matrix. For example, let’s get the main diagonal entries from a given matrix. See the code below.
vector = [1 2 3;4 5 6;7 8 9]
diagonal = diag(vector)
Output:
vector =
1 2 3
4 5 6
7 8 9
diagonal =
1
5
9
In the above code, we used the diag()
function to find the diagonal entries from the given matrix, and you can see the function returned the diagonal entries of the given matrix. You can also get the diagonal entries above and below the main diagonal using the second argument inside the diag()
function as we used above to make the diagonal matrix with elements above the main diagonal. Check this link for more information about the diag()
function.
Make Diagonal Matrix Using spdiags()
Function in MATLAB
To make a diagonal matrix with more than one diagonal or to get all the diagonal entries of a matrix, you can use the spdiags()
function in MATLAB. The first argument passed inside the spdiags()
function are the values, the second argument is the diagonal places, and the third and fourth argument is the size of the diagonal matrix. For example, let’s make a tridiagonal matrix from a given vector. See the code below.
v1 = [1 2 3 4 5].';
v2 = [2 2 2 2 2].';
v3 = [3 3 3 3 3].';
diagonal = spdiags([v2 v1 v3],-1:1,5,5);
matrix = full(diagonal)
Output:
matrix =
1 3 0 0 0
2 2 3 0 0
0 2 3 3 0
0 0 2 4 3
0 0 0 2 5
In the above code, we made a 5x5
tridiagonal matrix with the diagonal entries stored inside the three variables: v1
, v2
, and v3
. You can create the diagonal matrix with as many values as you want. You can also change the diagonal values of a given matrix using the springs()
function. To do that, pass the new values in the first argument, the place of the values as the second argument, and the matrix whose values you want to change as the third argument. For example, let’s change the main diagonal entries of the above tridiagonal matrix. See the code below.
v1 = [1 2 3 4 5].';
v2 = [2 2 2 2 2].';
v3 = [3 3 3 3 3].';
diagonal = spdiags([v2 v1 v3],-1:1,5,5);
matrix1 = full(diagonal)
v4 = [9 9 9 9 9].';
diagonal = spdiags(v4,0,diagonal);
matrix2 = full(diagonal)
Output:
matrix1 =
1 3 0 0 0
2 2 3 0 0
0 2 3 3 0
0 0 2 4 3
0 0 0 2 5
matrix2 =
9 3 0 0 0
2 9 3 0 0
0 2 9 3 0
0 0 2 9 3
0 0 0 2 9
In the above code, we changed the values of the main diagonal of matrix1
. The values are changed, and the new matrix is saved in the variable matrix2
. You can also extract all the non-zero diagonal entries from a given matrix using the spdaigs()
function. For example, let’s extract the non-zero entries of the above matrix. See the code below.
v1 = [1 2 3 4 5].';
v2 = [2 2 2 2 2].';
v3 = [3 3 3 3 3].';
diagonal = spdiags([v2 v1 v3],-1:1,5,5);
matrix = full(diagonal)
diag_Values = spdiags(matrix)
Output:
matrix =
1 3 0 0 0
2 2 3 0 0
0 2 3 3 0
0 0 2 4 3
0 0 0 2 5
diag_Values =
2 1 0
2 2 3
2 3 3
2 4 3
0 5 3
The diagonal values of the given matrix are extracted and stored inside the variable diag_values
. The diagonal values are stored in the columns of the matrix. The first diagonal entries are stored in the first column and so on. Check this link for more information about the diag()
function.