MATLAB Brackets
- Use the Square Brackets to Create Arrays and Matrices in MATLAB
- Use the Square Brackets to Concatenate Arrays in MATLAB
- Use the Square Brackets for Indexing and Slicing Arrays in MATLAB
- Use the Curly Brackets to Create and Access Cell Arrays in MATLAB
- Use the Curly Brackets to Concatenate Cell Arrays in MATLAB
- Use the Curly Brackets to Create Nested Cell Arrays in MATLAB
- Conclusion
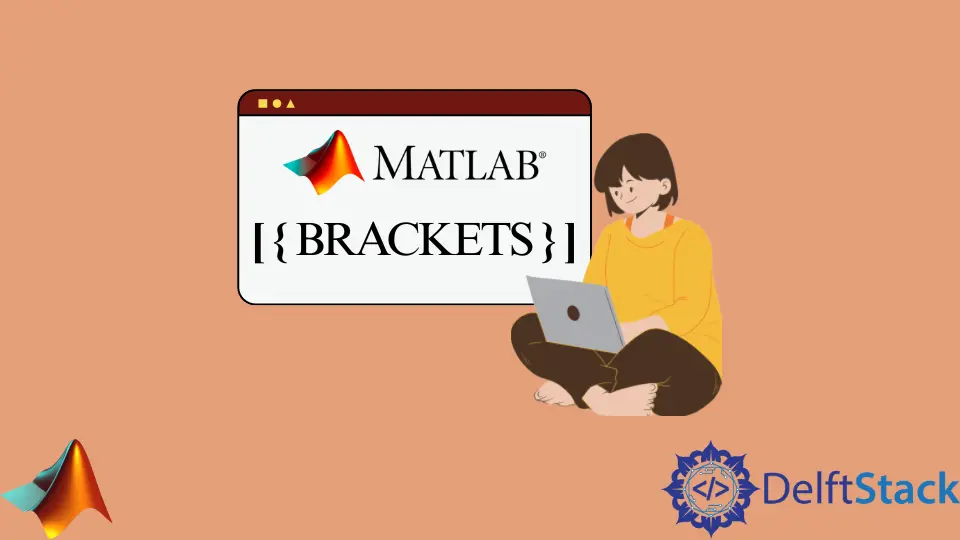
MATLAB, a powerful numerical computing environment, relies extensively on the effective use of brackets to perform various tasks. Brackets in MATLAB come in two primary forms: square brackets ([]
) and curly brackets ({}
).
Understanding how to wield these symbols is essential for any MATLAB user, as they play a pivotal role in defining arrays, matrices, cell arrays, and more. From creating and manipulating numerical structures to organizing heterogeneous data types, brackets are a cornerstone of MATLAB programming.
In this article, we will delve into the intricacies of using both square and curly brackets in MATLAB. Through a series of detailed code examples and explanations, we will explore how these brackets facilitate tasks such as array creation, concatenation, indexing, and the handling of cell arrays.
Use the Square Brackets to Create Arrays and Matrices in MATLAB
One fundamental feature in MATLAB is the use of square brackets. Square brackets are commonly used to create arrays and matrices by enclosing a set of elements.
The elements can be numeric values, variables, or expressions.
Let’s start with a basic example:
Example: Creating a Row Vector
rowVector = [1, 2, 3, 4, 5];
disp(rowVector);
Here, we create a row vector named rowVector
using square brackets. The elements 1
, 2
, 3
, 4
, and 5
are enclosed within the brackets, defining a one-dimensional array.
The disp
function is then used to display the contents of the vector.
Output:
1 2 3 4 5
Example: Creating a 2x3 Matrix
matrix2x3 = [10, 20, 30; 40, 50, 60];
disp(matrix2x3);
In this example, we create a 2x3 matrix named matrix2x3
by specifying two rows separated by a semicolon. Each row contains three elements. The disp
function is used to display the matrix.
Output:
10 20 30
40 50 60
Use the Square Brackets to Concatenate Arrays in MATLAB
Square brackets can also be used to concatenate arrays horizontally or vertically. Here are examples of both operations:
Example: Concatenating Two Row Vectors Horizontally
vectorA = [1, 2, 3];
vectorB = [4, 5, 6];
concatenatedVector = [vectorA, vectorB];
disp(concatenatedVector);
Here, we concatenate two-row vectors (vectorA
and vectorB
) horizontally using square brackets. The result is stored in the variable concatenatedVector
, and the disp
function is used to display the concatenated vector.
Output:
1 2 3 4 5 6
Example: Concatenating Two Matrices Vertically
matrixC = [7, 8, 9; 10, 11, 12];
matrixD = [13, 14, 15; 16, 17, 18];
concatenatedMatrix = [matrixC; matrixD];
disp(concatenatedMatrix);
In the above example, we concatenate two matrices (matrixC
and matrixD
) vertically using square brackets. The result is stored in the variable concatenatedMatrix
, and the disp
function is used to display the concatenated matrix.
Output:
7 8 9
10 11 12
13 14 15
16 17 18
Use the Square Brackets for Indexing and Slicing Arrays in MATLAB
Square brackets are essential for indexing and slicing arrays in MATLAB. Let’s look at some examples:
Example: Indexing a Specific Element in a Vector
vectorE = [21, 22, 23, 24, 25];
elementAtIndex3 = vectorE(3);
disp(elementAtIndex3);
We access the third element of the vector vectorE
using indexing. The result is stored in the variable elementAtIndex3
, and the disp
function is used to display the value.
Output:
23
Example: Slicing a Portion of a Matrix
matrixF = [31, 32, 33; 34, 35, 36; 37, 38, 39];
slicedMatrix = matrixF(2:3, 1:2);
disp(slicedMatrix);
Here, we slice a portion of the matrix matrixF
using indexing.
The result is a submatrix containing elements from the second and third rows and the first and second columns. The disp
function is used to display the sliced matrix.
Output:
34 35
37 38
Square brackets in MATLAB are a versatile tool for creating, concatenating, indexing, and slicing arrays and matrices. Understanding their various applications is fundamental for effective MATLAB programming.
Use the Curly Brackets to Create and Access Cell Arrays in MATLAB
In MATLAB, curly brackets {}
are a powerful tool primarily used for cell arrays. Cell arrays allow the storage of data of different types and sizes within a single variable.
Let’s explore some fundamental examples:
Example: Creating a Cell Array
cellArray = {'apple', 3.14, [1, 2, 3]};
disp(cellArray);
As you can see, we create a cell array named cellArray
using curly brackets.
The elements within the curly brackets can be of different types, including strings, numbers, and even other arrays. The disp
function is then used to display the content of the cell array.
Output:
{'apple'} {[3.1400]} {[1 2 3]}
Example: Accessing Elements in a Cell Array
secondElement = cellArray{2};
disp(secondElement);
Here, we use curly brackets for indexing to access the second element of the cellArray
. The result is stored in the variable secondElement
, and the disp
function is used to display its value.
Output:
3.1400
Use the Curly Brackets to Concatenate Cell Arrays in MATLAB
Curly brackets can be employed to concatenate cell arrays, both horizontally and vertically. Let’s explore these concatenation operations:
Example: Concatenating Two Cell Arrays Horizontally
cellArrayA = {'apple', 'orange'};
cellArrayB = {'banana', 'grape'};
concatenatedCellArrayH = [cellArrayA, cellArrayB];
disp(concatenatedCellArrayH);
In this code, we concatenate two cell arrays (cellArrayA
and cellArrayB
) horizontally using square brackets. The resulting cell array is stored in the variable concatenatedCellArrayH
, and disp
is used to display its content.
Output:
{'apple'} {'orange'} {'banana'} {'grape'}
Example: Concatenating Two Cell Arrays Vertically
cellArrayA = {'apple', 'orange'};
cellArrayB = {'banana', 'grape'};
concatenatedCellArrayV = {cellArrayA; cellArrayB};
disp(concatenatedCellArrayV);
Here, we create a nested cell array named nestedCellArray
. It contains sub-cells for both fruits and numbers, demonstrating the hierarchical structure achievable with curly brackets. The disp
function is then used to display the content of the nested cell array.
Output:
'fruits' {1x3 cell} 'numbers' {1x3 cell}
Curly brackets play a crucial role in MATLAB when working with cell arrays. Understanding how to create, access, concatenate, and nest cell arrays using curly brackets provides MATLAB users with the flexibility to handle diverse data types and structures efficiently.
Conclusion
The use of brackets in MATLAB is a fundamental aspect of programming that empowers users to define arrays, matrices, cell arrays, and more.
Square brackets are essential for creating and manipulating numerical data structures, allowing for seamless operations such as concatenation, indexing, and slicing. They provide a versatile means to organize and work with data in a straightforward manner.
On the other hand, curly brackets are a key component in dealing with cell arrays, enabling the storage of heterogeneous data types within a single variable. Their usage extends beyond mere storage, allowing for the creation of nested structures and facilitating the representation of complex data hierarchies.
Whether it’s square brackets for numerical arrays or curly brackets for cell arrays, a solid understanding of these symbols is important for MATLAB users.