How to Create Random Matrix in MATLAB
-
Generate Random Numbers Using the
rand()
Function in MATLAB -
Generate Random Numbers Using
randi()
Function in MATLAB -
Generate Random Numbers Using
randn()
Function in MATLAB -
Generate Random Numbers Using
randperm()
Function in MATLAB -
Generate Random Numbers Using
betarnd()
Function in MATLAB -
Generate Random Numbers Using
random()
Function in MATLAB
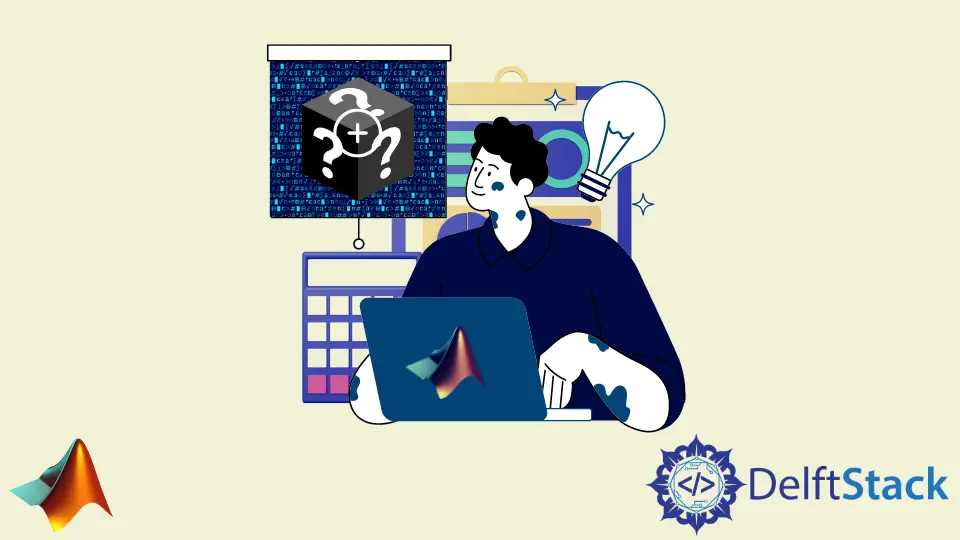
This tutorial will discuss how to generate or create random numbers using the rand()
, randi()
, randn()
, randperm()
, betarand()
, and random()
function in MATLAB.
Generate Random Numbers Using the rand()
Function in MATLAB
If you want to generate uniformly distributed random numbers, you can use the rand()
function in MATLAB, which generates random numbers between 0 and 1. You can also specify the size of the matrix containing random values, and each value will be between 0 and 1, which you can scale according to your requirements by multiplying them with a scaler. For example, let’s generate a 2-by-2 matrix of random values using the rand()
function. See the code below.
rn = rand(2)
Output:
rn =
0.2259 0.2277
0.1707 0.4357
As you can see in the output, a 2-by-2 matrix containing random values between 0 and 1 is generated. If you want to specify the range of the random numbers, you have to use the below formula.
rn = a + (b-a).*rand(n,1)
In this formula, a
is the lower limit, b
is the upper limit, and n
is the length of the random numbers. For example, let’s generate ten random numbers in the range of 2 to 8. See the code below.
a = 2;
b = 8;
n = 10;
rn = a + (b-a).*rand(n,1)
Output:
rn =
7.5403
4.5812
3.1089
7.4293
7.8785
4.6332
2.6667
3.5484
4.4523
5.5694
There are ten random numbers in the range of 2 to 8. If you only want integers in the output, you can convert these random numbers to integers using the round()
function, which rounds a floating-point number to the nearest integer. You can also clone the size and data type of the random numbers from an existing array using the size()
function for size and like
property for the data type. For example, let’s create an array and generate random values according to that array’s size and data type. See the code below.
v = [2 3 1 5]
rn = rand(size(v),'like',v)
Output:
v =
2 3 1 5
rn =
0.4886 0.5785 0.2373 0.4588
The size and the data type of the array and the random numbers are the same. Check this link for more details about the rand()
function.
Generate Random Numbers Using randi()
Function in MATLAB
The above function generates floating-point random numbers, but if you want to generate random integer numbers, you can use the randi()
function in MATLAB, which generates random integer numbers from 1 to a specified integer which you can specify as a first argument in the randi()
function. You can also specify the size of the output matrix containing random values as the second and third argument. For example, let’s generate a 3-by-3 matrix containing random integer numbers from 1 to 15. See the code below.
rn = randi(15,3,3)
Output:
rn =
6 1 8
11 12 14
3 8 10
The matrix is of size 3-by-3 which contains random integer numbers between 1 and 15. You can also generate random integer numbers between a specific range, and you just have to pass the range in box brackets as the first argument of the randi()
function. For example, let’s generate 10 random numbers between -10 to 10. See the code below.
rn = randi([-10,10],10,1)
Output:
rn =
2
8
6
2
-7
-5
8
-10
0
-7
There are ten random numbers in the range of -10 to 10. You can also define the data type of the integer numbers by passing the datatype name in the randi()
function. The data types that you can choose are: 'single'
, 'double'
, 'int8'
, 'uint8'
, 'int16'
, 'uint16'
, 'int32'
, or 'uint32'
. You can define the size of the random numbers from the size of the existing array using the size()
function and the numeric datatype using the like
property. For example, let’s generate a matrix of random values depending on the size and numeric datatype of an existing array. See the code below.
v = [1 2; 6 7]
rn = randi(7,size(v),'like',v)
Output:
v =
1 2
6 7
rn =
6 1
7 7
The size and the data type of the array and the random numbers are the same. Check this link for more details about the randi()
function.
Generate Random Numbers Using randn()
Function in MATLAB
If you want to generate normally distributed random numbers, you can use the randn()
function in MATLAB. The randn()
function is the same as the rand()
function with only the difference in distribution type. The rand()
function generates uniformly distributed random numbers while the randn()
function generates normally distributed random numbers. You can use any of these functions depending on your requirements. Check this link for more details about the randn
function.
Generate Random Numbers Using randperm()
Function in MATLAB
If you want to generate a random permutation of integers, you can use the randperm()
function in MATLAB. The random permutation of the integers will be between 1 to a specific number which you can define in the randperm()
function as the first argument. You can also define the number of integers you want to generate as the second argument in the function. For example, let’s generate a random permutation of 6 unique integers. See the code below.
rn = randperm(10,5)
Output:
rn =
2 3 10 8 7
All the integers are unique and range from 1 to 10. Note that the randperm()
function is the same as the randi()
function with a difference that the randperm()
generates unique integers, whereas in the randi()
function, the integers can be repeated. Check this link for more details about the randperm
function.
Generate Random Numbers Using betarnd()
Function in MATLAB
If you want to generate random numbers from the beta distribution, you can use the betarnd()
function in MATLAB. This function generates random integers specified by the first and second argument: vectors, matrices, or arrays of the same size. For example, let’s generate a 1-by-5 matrix of random numbers from beta distribution using two vectors as input. See the code below.
a = [1 2 3 4 5];
b = [9 8 7 6 5];
rn = betarnd(a,b)
Output:
rn =
0.1234 0.1847 0.3334 0.2689 0.3678
You can also specify the size of the output matrix by defining it in the third and fourth argument of the betarnd()
function. Check this link for more details about the betarnd()
function.
Generate Random Numbers Using random()
Function in MATLAB
If you want to generate random numbers from a specified distribution type, you can use the random()
function in MATLAB. You have to define the name of the distribution as the first argument, and then after that, you need to pass the distribution parameters. For example, let’s generate normally distributed random numbers using a sigma value of 0 and a mu value of 1 using the random()
function. See the code below.
s = 0;
m = 1;
rn = random('Normal',s,m)
Output:
rn =
-0.1649
You can define your required distribution name in the function. You can use many types of distributions like: Beta
, Binomial
, Exponential
, Gamma
, and many more. Check this link for more details about the random
function.
Related Article - MATLAB Random
- Selection Methods for a Random Sample From Matrix or Array With Dataset in MATLAB
- How to Get Random Permutation Using MATLAB