How to Get File Name and Extension Using Bash
- Get File Name and Extension Using Bash
- Executing the Bash File to Get File Name and Extension
- Permission Related Issues
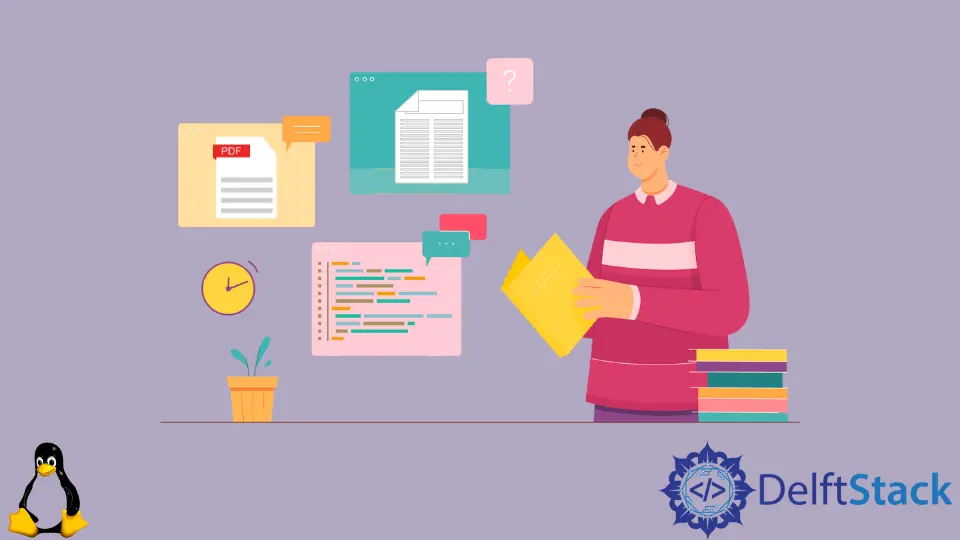
Bash scripting is becoming quite popular in these recent years, be it for your everyday Linux administration task or an entire DevOps automation task.
Suppose you find yourself in a situation where you want to extract a filename and its extension separately in a bash script. For example, there is a python file with the name server.py
. We want to slice this string into two parts by separating the name server
and the extension py
.
Get File Name and Extension Using Bash
We have written a bash script for demonstration purposes. You can use this code anywhere in your script if you want to.
- First, we will check if the user has provided the proper arguments or not. The following code snippet uses an
if
statement becoming equal=
to zero0
, which would mean that no arguments have been provided; in that case, we would usepwd
or our current working directory as the default argument.
#!/bin/bash
# Checking if arguments have been provided:
if [[ $# == 0 ]]; then
cDir=$(pwd) # Setting the cDir variable to current directory
echo "[-] No directory provided!"
else
cDir=$1
fi
echo "[+] Setting the directory to $cDir"
- Next, we check if the directory provided by the user exists or not. If it does not exist, we exit the script.e
# Checking if the directory exists:
if [[ ! -d $cDir ]]; then
echo "[-] Directory $cDir doesn't exist!"
exit
fi
- Now, we start extracting the files available in the directory (of course, if it’s valid) in the
allFile
variable using the simplels
command, which runs in Linux as well as PowerShell in Windows operating systems.
# Main extraction:
allFile=`ls $cDir`
echo;
Here, we check if the last value of the path provided is not /
, and if that is not the case, we add it to the end to avoid any errors.
# Checking if the last value of the path is '/' or not:
if [[ ${cDir: -1} != '/' ]]; then
cDir+='/'
fi
- Next, we iterate over everything present in the folder using
for loop
and the variable$allFile
. Remember from earlier that$allFile
has a value ofls $cDir
.
# Iterating over everything in the folder
for item in $allFile; do
# Appending path to each file:
item="$cDir$item"
We check if the file detected is of type file
using the -f
flag in the loop. If that is the case, we remove the dot part from the file name - for example, the extension part.
In the end, we print the results in our desired way using echo
.
# Checking if current item is a file:
if [[ -f $item ]]; then
ext=`ls $item | rev | cut -d '.' -f 1 | rev`
file_name=`ls $item | rev | cut -d '.' -f 2 | rev`
echo "File Name: $file_name -> Extension: $ext"
fi
This script checks if a specific directory exists or not. And if the directory exists, it will then mention all the files along with their extension.
┌─[root@parrot]─[/home/user/Downloads]
└──╼ #./test2.sh
[-] No directory provided!
[+] Setting the directory to /home/user/Downloads
File Name: /home/user/Downloads/ExtraCredit_Sockets_Intro -> Extension: pdf
File Name: /home/user/Downloads/project -> Extension: sh
File Name: /home/user/Downloads/test2 -> Extension: sh
File Name: /home/user/Downloads/test -> Extension: txt
Each line of the code is commented on, so you can check it out if you have any confusion.
If you want to use the script, here is its full version.
#!/bin/bash
# Checking if arguments have been provided:
if [[ $# == 0 ]]; then
cDir=$(pwd) # Setting the cDir variable to current directory
echo "[-] No directory provided!"
else
cDir=$1
fi
echo "[+] Setting the directory to $cDir"
# Checking if the directory exists:
if [[ ! -d $cDir ]]; then
echo "[-] Directory $cDir doesn't exist!"
exit
fi
# Main extraction:
allFile=`ls $cDir`
echo;
# Checking if the last value of the path is '/' or not:
if [[ ${cDir: -1} != '/' ]]; then
cDir+='/'
fi
# Iterating over everything in the folder
for item in $allFile; do
# Appending path to each file:
item="$cDir$item"
# Checking if current item is a file:
if [[ -f $item ]]; then
ext=`ls $item | rev | cut -d '.' -f 1 | rev`
file_name=`ls $item | rev | cut -d '.' -f 2 | rev`
echo "File Name: $file_name -> Extension: $ext"
fi
done
Executing the Bash File to Get File Name and Extension
In case you are a newbie trying to learn bash and do not know how to run, use this code; all you have to do is make a file with the .sh
extension, which means it is a bash file.
After that, navigate to that directory using the terminal of your choice and type ./filename
, which should execute the file.
If you are on a Windows operating system, type bash file.sh
, and it should run without any problems.
Remember to use the right file name of the script in your case.
Permission Related Issues
Permission Denied
error while running the script.You need to provide the right permissions using chmod +x [file name]
in the terminal.
Run the PowerShell or command prompt as administrator if you are on windows to avoid any permission-related issue.