How to Read a File Into an Array Using Bash
-
Use the
readarray
Method to Read a File Into an Array Using Bash - Use the General Method to Read a File Into an Array Using Bash
-
Use a
while
Loop to Read a File Into an Array Using Bash -
Use
mapfile
to Read a File Into an Array Using Bash -
Use a
for
Loop to Read a File Into an Array Using Bash - Conclusion
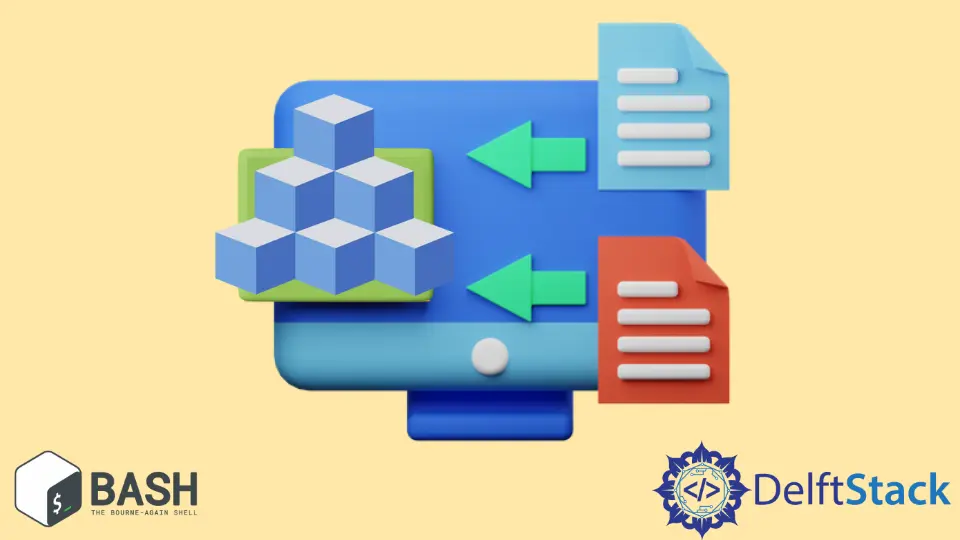
This tutorial provides a comprehensive understanding of different approaches to read lines from a file and load them into an array using Bash, catering to various versions of the Bash shell.
Use the readarray
Method to Read a File Into an Array Using Bash
The readarray
function, available in Bash 4.0 and later versions, is an efficient way to read a file into an array.
If you’re unsure about the version of Bash you’re using, you can determine it with the following command:
echo ${Bash_VERSION}
For versions prior to 4.0, the readarray
method won’t be applicable, so we’ll explore an alternative method later.
In this tutorial, we’ll utilize a sample file named numbers.txt
with the following content:
1 1
2 1
3 1
4 1
5 1
6 1
7 1
8 1
9 1
10 1
To read this file and store its contents in an array, you can use the readarray
command with the following syntax:
readarray -t Arr < numbers.txt
Here, readarray
is the command, -t
removes newline characters, Arr
is the array where the file contents will be stored, and numbers.txt
is the file to be read.
If the file isn’t in the same folder as your script, ensure to provide the complete path to the file.
You can access and display specific lines of the array using indices, like in this example:
echo ${Arr[1]}
This code will output the line at index 1
of the array, which corresponds to the file’s second line.
The output will be:
2 1
To display the entire array, use the following syntax:
echo ${Arr[@]}
The output will be:
1 1 2 1 3 1 4 1 5 1 6 1 7 1 8 1 9 1 10 1
Use the General Method to Read a File Into an Array Using Bash
In case your Bash version is older than 4.0, the readarray
method won’t work for you. Instead, you can use a more general approach to read a file into an array.
The syntax for this general method is as follows:
IFS=$'\r\n' GLOBIGNORE='*' command eval 'ArrName=($(cat filename))'
To read this array, you can utilize a similar syntax to the previous example:
echo ${ArrName[1]}
This Bash line will display the contents at index 1
of the array. If you want to display the entire array’s contents, you can use the following command:
echo ${ArrName[@]}
Description of the General Method
In this syntax, ArrName
represents the array’s name, and filename
after the cat
is the name of the file to be read. These variables can be customized based on your requirements.
The IFS
variable defines the breaking characters, which are \r
and \n
in this case. \r
represents the carriage return, and \n
is the line-feed character.
Typically, \n
is used as a new line character in modern systems.
Setting GLOBIGNORE='*'
is a safety measure to handle unusual edge cases with filenames. It’s important to note that this command specifically requires Bash and may not work with other shells such as zsh
or fish
, primarily due to the use of GLOBIGNORE
.
It’s worth mentioning that this solution is notably slower than using readarray
. The recommendation to use this method is primarily for cases where your Bash version is older than 4.0.
Let’s expand on reading lines from a file and loading them into an array using Bash by incorporating the while
loop, mapfile
, and for
loop methods.
Use a while
Loop to Read a File Into an Array Using Bash
The while
loop is a fundamental construct in Bash that can also be used to read lines from a file and load them into an array.
Assuming we have a file called numbers.txt
with the desired content, you can use the following script to read the file line by line and store the lines in an array:
# Initialize the array
Arr=()
# Read the file line by line and store it in the array
while IFS= read -r line; do
Arr+=("$line")
done < numbers.txt
In this script, the while
loop reads each line from numbers.txt
and appends it to the array Arr
. The read
command with the -r
option ensures that backslashes are not interpreted.
To display the contents of the array, you can use the same echo
commands as shown in the previous examples.
Use mapfile
to Read a File Into an Array Using Bash
The mapfile
command, built into Bash, simplifies reading lines from a file and storing them directly into an array.
The basic syntax for using mapfile
to read lines from a file into an array is as follows:
mapfile -t array_name < filename
Parameters:
mapfile
: This is the command to read lines from standard input (or a file) and store them in an array.-t
: This option is used to remove trailing newline characters from each line.array_name
: Replace this with the name of the array you want to store the lines in.< filename
: This redirects the content of the filefilename
to be read as input formapfile
.
Here’s an example usage of mapfile
:
mapfile -t Arr < numbers.txt
This command reads lines from numbers.txt
and stores them directly into the array Arr
.
Displaying the contents of the array can be done using the same echo
commands mentioned earlier.
Use a for
Loop to Read a File Into an Array Using Bash
A for
loop can also be utilized to read lines from a file and populate an array.
Here’s a script that demonstrates this approach:
# Initialize the array
Arr=()
# Read the file using a for loop
while IFS= read -r line; do
Arr+=("$line")
done < numbers.txt
In this script, the for
loop reads each line from numbers.txt
and appends it to the array Arr
.
Displaying the contents of the array can be achieved using the same echo
commands as demonstrated in previous examples.
Conclusion
We have explored various methods in Bash to read lines from a file and load them into an array. The readarray
function for Bash 4.0+ efficiently handles this, while while
loops and mapfile
provide alternatives for earlier versions.
Utilizing a for
loop is also an option. Each approach caters to different Bash versions, offering flexibility based on specific requirements and preferences for processing file content into arrays.
Choose the most suitable method based on your Bash version and the specific needs of your script.