Division in Bash
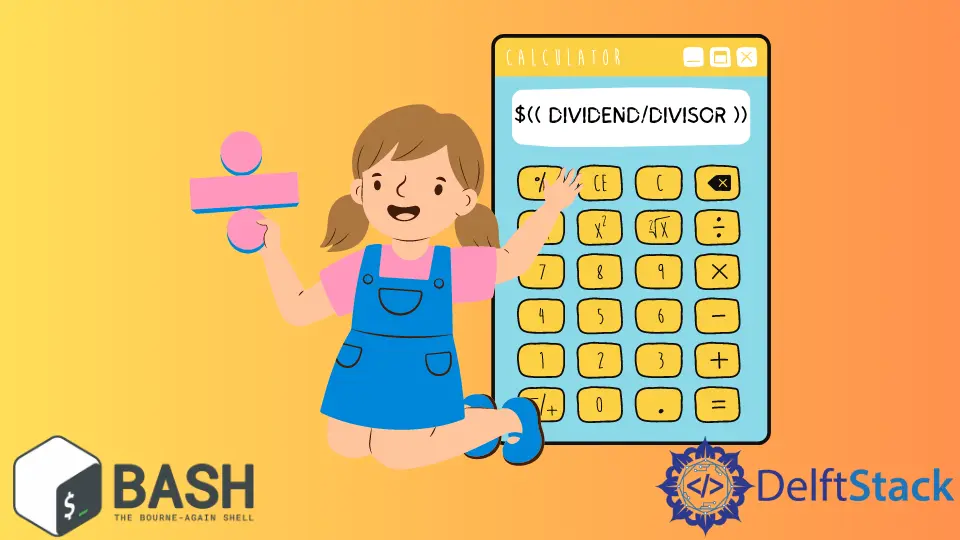
When working with Bash, performing division can be a bit tricky due to its nature as a shell scripting language primarily designed for file manipulation and process control. However, mastering division in Bash is essential for anyone looking to automate tasks or perform calculations directly in the command line.
In this tutorial, we will explore how to divide numbers using Bash, including different methods and practical examples. Whether you’re a seasoned developer or a newcomer to scripting, understanding these techniques will enhance your productivity and efficiency. Let’s dive into the world of Bash division!
Understanding Division in Bash
Bash does not support floating-point arithmetic natively. Instead, it handles integer arithmetic. When you attempt to divide two integers, Bash will return an integer result, discarding any remainder. This can be limiting, but there are ways to manage floating-point division using external tools like bc
or awk
.
Method 1: Basic Integer Division using Bash Arithmetic
The simplest way to perform division in Bash is through arithmetic expansion using the (( ))
syntax. This method is straightforward, but it only handles integer division.
#!/bin/bash
num1=10
num2=3
result=$((num1 / num2))
echo "The result of $num1 divided by $num2 is: $result"
Output:
The result of 10 divided by 3 is: 3
In this example, we defined two variables, num1
and num2
, and used the arithmetic expansion syntax to perform the division. The result is stored in the result
variable. When we print the result, we see that Bash returns 3, which is the integer quotient of 10 divided by 3.
This method is efficient for simple tasks but remember that it only provides whole numbers. If you need a more precise calculation, you will need to look into alternative methods.
Method 2: Floating-Point Division using bc
For scenarios where you need to perform floating-point division, the bc
command-line calculator comes in handy. It allows you to handle decimal numbers effortlessly.
#!/bin/bash
num1=10
num2=3
result=$(echo "scale=2; $num1 / $num2" | bc)
echo "The result of $num1 divided by $num2 is: $result"
Output:
The result of 10 divided by 3 is: 3.33
In this script, we use echo
to pass the division expression to bc
. The scale
variable defines the number of decimal places to return. In this case, we specified scale=2
, which gives us two decimal points in the result. The output shows the division result as 3.33, demonstrating how bc
can provide more accurate calculations than simple integer division.
Method 3: Using awk
for Division
Another powerful tool for division in Bash is awk
, a versatile programming language designed for pattern scanning and processing. It can handle complex calculations, including floating-point arithmetic.
#!/bin/bash
num1=10
num2=3
result=$(awk "BEGIN {printf \"%.2f\", $num1/$num2}")
echo "The result of $num1 divided by $num2 is: $result"
Output:
The result of 10 divided by 3 is: 3.33
In this example, we utilize awk
to perform the division. The BEGIN
block allows us to execute code before any input is processed. The printf
function formats the output to two decimal places. This method is particularly useful when you need to format the output neatly while performing calculations, making it a favorite among many Bash users.
Conclusion
In this tutorial, we explored various methods for performing division in Bash, from basic integer division to more advanced floating-point calculations using tools like bc
and awk
. Each method has its strengths, and the choice of which to use largely depends on your specific needs and the level of precision required. By mastering these techniques, you can enhance your scripting capabilities and make your Bash scripts more powerful and efficient.
FAQ
- How does Bash handle division?
Bash performs integer division by default, discarding any remainder.
-
Can Bash perform floating-point division?
Yes, by using external tools likebc
orawk
, you can achieve floating-point division. -
What is the
bc
command used for?
bc
is a command-line calculator that allows for precise mathematical operations, including floating-point arithmetic. -
Is
awk
better thanbc
for division?
It depends on the context.awk
is great for formatted output and complex calculations, whilebc
is straightforward for basic arithmetic. -
Can I use division in Bash scripts?
Absolutely! Division can be performed in Bash scripts using the methods discussed in this tutorial.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn