How to Add Numbers in Bash
-
Using
expr
for Addition in Bash - Using Arithmetic Expansion for Addition in Bash
-
Using
bc
to Add Floating-Point Numbers in Bash -
Using
awk
to Add Floating-Point Numbers in Bash
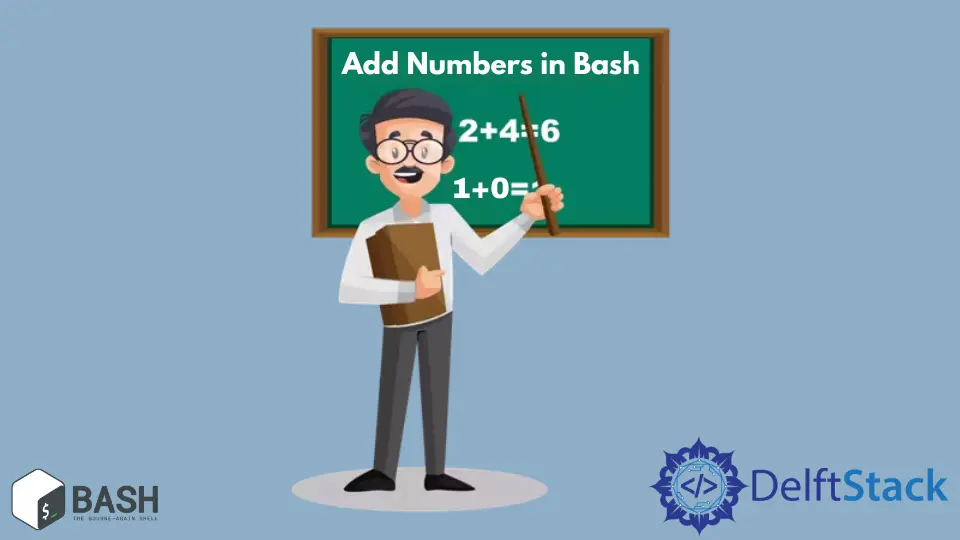
This tutorial shows different ways of adding numbers in a bash script using expr
, arithmetic expansion, bc
, and awk
.
Using expr
for Addition in Bash
expr
is a command-line Unix utility used to evaluate a given expression and prints out the value to the standard output. expr
can evaluate integer or string expressions, including pattern matching and regular expressions. expr
uses the syntax below.
$expr expression
To add two integers in a bash script using expr
, use either of the following syntaxes. The first syntax uses quotes, while the second syntax uses parenthesis and starts with a dollar symbol.
total=`expr $num1 + $num2`
total=$(expr $num1 + $num2)
Please note that there is no space before and after the =
sign. Not having space when assigning values to variables is the built-in bash syntax.
Let us write a bash script that adds two numbers using expr
with quotes. The two integers, 2
and 3
, are assigned to the variables num1
and num2
, respectively. The sum of the two variables is assigned to the total
variable, and the echo
command is used to print the value of the total
variable to the standard output.
num1=2
num2=3
total=`expr $num1 + $num2`
echo $total
Run the script.
bash add.sh
Running the script gives the following output.
5
Let us also create a bash script that uses expr
with parenthesis to add two integers.
num1=2
num2=3
total=$(expr $num1 + $num2)
echo $total
Run the script.
bash add.sh
Once the script is executed, it produces the following output.
5
Using Arithmetic Expansion for Addition in Bash
Arithmetic expansion in Linux uses the built-in shell expansion to use parenthesis for mathematical calculations. The following syntax is used for arithmetic expansion.
$(( arithmentic expression ))
The script below demonstrates adding two integers using arithmetic expansion.
num1=2
num2=3
total=$(($num1 + $num2))
echo $total
Run the script.
bash add_int.bash
The script produces the output below.
5
Using bc
to Add Floating-Point Numbers in Bash
bc
is a short form for Bash Calculator. It is used to perform floating-point arithmetic operations. The -l
option tells bc
to use the predefined math routines.
num1=1.1
num2=1.4
echo $num1 + $num2 | bc -l
Run the script.
bash add_float.sh
Running the script above produces the following output.
2.5
Using awk
to Add Floating-Point Numbers in Bash
The script below can also be used to add two floating-point numbers using the awk
command and print the output to the standard output. The variable $1
has the value 1.5 and the variable $2
has the value 3.3.
echo 1.5 3.3 | awk '{print $1 + $2}'
Run the script.
bash add_float.sh
Running the above script produces the following output.
4.8