How to Sum a List of Numbers in a Text File Using Bash
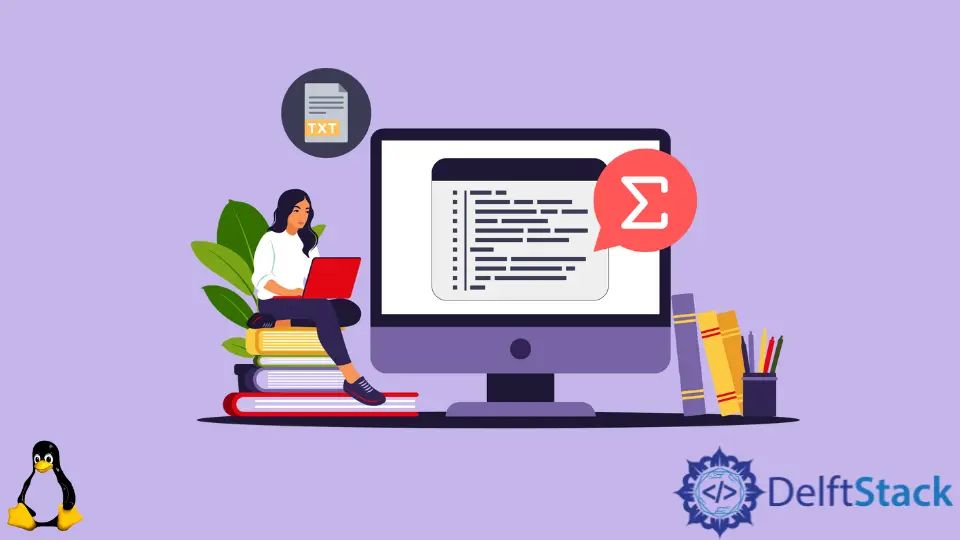
This tutorial will cover multiple methods for summing a list of numbers in a file using Bash scripting. The first method involves using the paste
command, and we will discuss two cases: Using an existing file and reading a file using stdin
.
Lastly, we will discuss the cut
and awk
commands to get the sums.
Sum a List of Numbers in a Text File Using Bash
The methods described below will assume that we have an input file with the title numbers.txt
.
numbers.txt
File:
1
2
3
4
5
6
7
8
9
10
Use an Existing File
If we have all the numbers stored in a simple text file with each number in a separate line, then we can call the following command in Bash and get the sum.
Command:
paste -sd+ numbers.txt | bc
Output:
55
Use stdin
(Standard Input)
The following command can read from a file and get the sum of numbers.
Command:
cat numbers.txt | paste -sd+ | bc
Output:
55
In this case, we use the command cat numbers.txt
to read from the file. This command can be replaced with any other that returns a list of numbers.
It should be noted that while paste
has a POSIX-defined specification, not every implementation is the same. The following command would need to be used in Mac OS to achieve the same output.
Command:
<cmd> | paste -sd+ - | bc
Use the cut
Command
Suppose we have a text file having multiple columns, and we want to get a sum of a specific column. We can achieve this using the cut
command.
Assume that we have a numbers.txt
file defined as follows:
1 1
2 1
3 1
4 1
5 1
6 1
7 1
8 1
9 1
10 1
We can separately sum up each of the columns using the command below.
Command:
cat numbers.txt | cut -d ' ' -f 2 | paste -sd+ | bc -l
The argument after -f
represents the column number to be summed. It should also be noted that the count for column numbers, in this case, begins with 1
, not 0
.
As we added passed 2
for the -f
flag, we will get the sum of the second column.
Output:
10
Use the awk
Command
The awk
command, named after its authors, is more suited to the task than our previous methods. When measured, awk
performs much better than our previous methods.
Command:
awk '{s+=$1} END {print s}' numbers.txt
Output:
55
You can replace numbers.txt
with the name of the file containing your numbers. This also allows us to bypass reading the file through cat
, which is partially responsible for the superior performance.