How to Suspend Function in Kotlin
- What is a Suspend Function?
- Defining a Suspend Function
- Calling Suspend Functions from Coroutines
- Combining Multiple Suspend Functions
- Conclusion
- FAQ
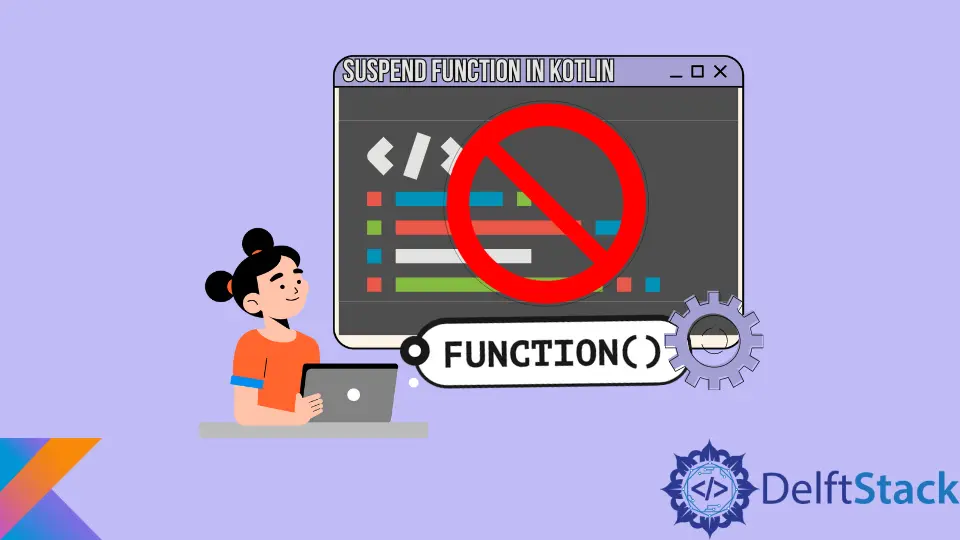
Kotlin, a modern programming language, has gained immense popularity, particularly for Android development. At the heart of Kotlin’s concurrency model is the concept of coroutines, which allows developers to write asynchronous code in a sequential manner. One of the most crucial aspects of coroutines is the suspend function.
This article will introduce you to what suspend functions are in Kotlin coroutines and provide practical guidance on how to implement them effectively. Whether you are a seasoned developer or a newcomer to Kotlin, understanding suspend functions will enhance your ability to handle asynchronous programming with ease.
What is a Suspend Function?
Suspend functions are a unique feature in Kotlin that allow you to pause the execution of a function without blocking the thread. This is particularly useful in scenarios where you are dealing with long-running tasks, such as network requests or database operations. By using suspend functions, you can write code that is more readable and maintainable, mimicking synchronous programming while leveraging the benefits of asynchronous execution.
When you declare a function as a suspend function, you can call other suspend functions within it. This enables you to structure your code in a way that is both efficient and easy to understand. The key to using suspend functions effectively lies in understanding how to define and invoke them correctly.
Defining a Suspend Function
To define a suspend function in Kotlin, you use the suspend
keyword before the function name. Here’s a simple example of how to create a suspend function that simulates a delay:
import kotlinx.coroutines.*
suspend fun simulateDelay() {
delay(1000) // Simulates a long-running task
println("Delay completed")
}
In this example, the simulateDelay
function is defined as a suspend function. The delay
function, which is also a suspend function, pauses the execution for one second. When you call simulateDelay
, it will pause for a second before printing “Delay completed.”
Output:
Delay completed
When you invoke this function, it is essential to call it from a coroutine context. This can be done using the runBlocking
or launch
functions. Here’s how you can call simulateDelay
within a coroutine:
fun main() = runBlocking {
simulateDelay()
}
This main
function uses runBlocking
, which creates a coroutine that blocks the main thread until the coroutine completes. This is a great way to test suspend functions in a simple application.
Output:
Delay completed
Calling Suspend Functions from Coroutines
To effectively utilize suspend functions, you must call them from a coroutine. This can be done using launch
or async
. Here’s an example using launch
:
import kotlinx.coroutines.*
fun main() {
GlobalScope.launch {
simulateDelay()
}
Thread.sleep(2000) // Wait for the coroutine to finish
}
In this example, we use GlobalScope.launch
to launch a new coroutine. The simulateDelay
function is called within this coroutine. The Thread.sleep(2000)
is used to keep the main thread alive long enough for the coroutine to complete its execution.
Output:
Delay completed
Using GlobalScope
is convenient for quick tests, but in production code, it’s better to manage coroutines with structured concurrency to avoid memory leaks.
Combining Multiple Suspend Functions
One of the powerful features of suspend functions is their ability to work together. You can create complex workflows by combining multiple suspend functions. Here’s an example where we define two suspend functions and call them sequentially:
import kotlinx.coroutines.*
suspend fun fetchData(): String {
delay(1000) // Simulate fetching data
return "Data fetched"
}
suspend fun processData(data: String) {
delay(500) // Simulate processing data
println("Processed: $data")
}
suspend fun fetchAndProcess() {
val data = fetchData()
processData(data)
}
fun main() = runBlocking {
fetchAndProcess()
}
In this code, fetchData
simulates fetching data, while processData
simulates processing that data. The fetchAndProcess
function orchestrates the two, calling fetchData
and then processData
with the result.
Output:
Processed: Data fetched
By structuring your code this way, you can maintain clarity and ensure that each part of your asynchronous workflow is easy to follow.
Conclusion
Suspend functions in Kotlin are a powerful tool for managing asynchronous programming. By allowing you to write code that looks synchronous while handling long-running tasks efficiently, they simplify the complexity often associated with concurrency. Understanding how to define and call suspend functions is essential for any Kotlin developer looking to leverage coroutines effectively. As you gain experience with these concepts, you’ll find yourself writing cleaner, more maintainable code that enhances the user experience in your applications.
FAQ
-
what is a suspend function in Kotlin?
A suspend function is a special type of function in Kotlin that can pause its execution without blocking the thread, allowing for asynchronous programming. -
how do I call a suspend function?
You can call a suspend function from within a coroutine using eitherlaunch
orrunBlocking
to ensure the function is executed in the correct context. -
can I call a suspend function from a regular function?
No, suspend functions can only be called from within a coroutine or another suspend function. -
what is the difference between launch and async in Kotlin coroutines?
launch
is used for fire-and-forget coroutines, whileasync
is used when you need to return a result from the coroutine. -
how do I handle errors in suspend functions?
You can use standard Kotlin error handling techniques, such as try-catch blocks, to manage exceptions in suspend functions.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn