Kotlin flatMap() Function
-
Common Pitfall When Using the
map()
Function in Kotlin -
Kotlin
flatMap()
Function -
an Alternative of the
flatMap()
Function in Kotlin - Conclusion
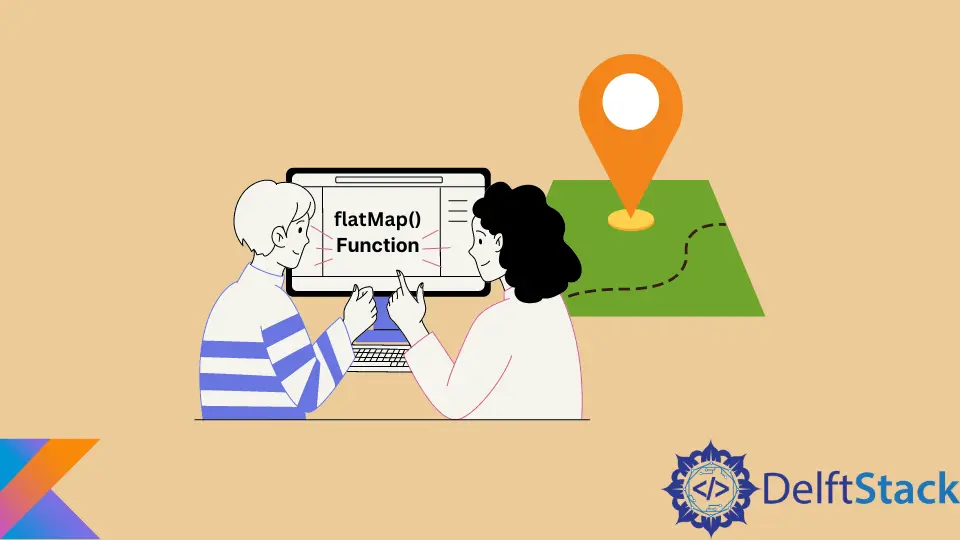
When developing applications, we always use the collections framework throughout the applications. If you have any list, you have interacted with collections.
We use collections to store related data temporarily in memory to execute different computations on the data. For example, collections help us find the data size from the database or a file.
These computations were quite hard to implement before streams were introduced in Java 8 because developers used an imperative programming approach.
The stream
API made the computations with collections quite easy to implement because the code uses a declarative programming approach.
In Kotlin, we can also use this programming approach with the same methods used in the stream
API, such as filter()
, map()
, flatMap()
, and others.
In this tutorial, we will learn how the flatMap()
function works in Kotlin, and the difference between flatMap()
and map()
functions.
Common Pitfall When Using the map()
Function in Kotlin
When using the map()
function, you might decide to get a single list after operating on two lists, but the function does not return a single list.
This is a common problem using the map()
method. The next section shows how the issue occurs.
Open IntelliJ development environment and select File
> New
> Project
. Enter the project name
as kotlin-flatmap
, select Kotlin
on the Language
section, and Intellij
on the Build system
section.
Create a Main.kt
file under the kotlin
folder and copy and paste the following code into the file.
val stringArray = arrayOf("Harry", "potter");
fun getUniqueCharacters(): List<List<String>> {
return stringArray.map { word -> word.split("") }
.distinct()
.toList();
}
fun main(){
println(getUniqueCharacters());
}
Our objective for all the examples we will cover in this tutorial is to return a single list containing distinct characters from an array with two strings, as shown above.
If you noted, the getUniqueCharacters()
method returns a list of lists List<List<String>>
which is the return type of the map()
function.
The map()
function returns a list containing two distinct lists, which goes against our objective. Run the code to verify that the map()
function returns two distinct lists, as shown below.
[[, H, a, r, r, y, ], [, p, o, t, t, e, r, ]]
Kotlin flatMap()
Function
Comment out the previous example and copy and paste the following code into the Main.kt
file after it.
val stringArray = arrayOf("Harry", "potter");
fun getUniqueCharacters(): List<String> {
return stringArray.map { word -> word.split("") }
.flatMap { it }
.distinct()
.toList();
}
fun main(){
println(getUniqueCharacters())
}
The getUniqueCharacters()
function returns a list containing a string which is the return type of the flatMap()
function.
The flatMap()
returns a single list containing unique elements by transforming the array using the split()
method to individual characters. The distinct()
method ensures the list returned contains unique characters.
Run the above code to verify a single list containing unique character results.
[, H, a, r, y, p, o, t, e]
an Alternative of the flatMap()
Function in Kotlin
Comment out the previous example and copy and paste the following code into the Main.kt
file after it.
val stringArray = arrayOf("Harry", "potter");
fun getUniqueCharacters(): List<String> {
return stringArray.map { word -> word.split("") }
.flatten()
.distinct()
.toList()
}
fun main(){
println(getUniqueCharacters())
}
In this example, we have used the flatten()
function which is similar to the flatMap()
function. The flatten()
function returns a single list by adding all the existing collections elements in one list.
Run this example to verify that it returns a single list containing all the unique characters from our array.
[, H, a, r, y, p, o, t, e]
Conclusion
In this tutorial, we have learned how the flatMap()
function works when you want to return a single list from multiple collections. We have also learned the drawback of using the map()
method to achieve the same objective.
If you want to read more about how other functions work in Kotlin, visit this Kotlin documentation which covers the functions in detail.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub